Introduction: Adapted Remote Controlled Outlet
I help out a local hospital by adapting toys and devices for children in speech, physical, and occupational therapy. One kid loved the sound of a box fan- however the AC part of adapting scared me off a bit both for kid safety and for liability reasons.
My workaround was to adapt the 12 volt DC remote instead. That's when my learning curve got steeper-
Requirements
1. a timer that controls an on switch and an off switch since the switch on the remote is designed to keep things on or off. (Decided on Arduino Nano)
2. a electrically safe means of controlling 12v supply with 5v signal. (Morphed from transistor to optocouplers)
3. plus a request from the therapist to make it adjustable for how long the device would remain on before shutting off.
Disclaimer- Apart from some LED's and example sketches from Arduino, this is my first microcontroller project. I'm sure this could be done cheaper/faster/more efficiently- but right now I'm just trying to keep it safe, keep my costs down, and get things done with time for that kid to turn on and off some Christmas cheer.
I'd love feedback if anyone has advice for cheaper or more elegant solutions. I liked that at the end of the day the device is scalable (more outlets), portable with Arduino, and relatively cheap (under $10 total) apart from the $20 for the outlets on Amazon.com.
Step 1: The Circuit
This particular remote had a common 12V source and appeared to be switching things on the ground side of the switch. I'd originally wanted to use some 3904 NPN transistors (first time) but was thwarted when the common ground between the Arduino and the remote led to false signals.
This led me to try some optocouplers.
Optocouplers:
I love these little guys! I used two 4N35 optocouplers to create two separate circuits. (Arduino & Remote). Since they are electrically separate I could switch the two remote buttons individually using a pair of them.
*Note - If you haven't used them before- half of the optocoupler is an LED hidden inside there. DON"T FORGET YOUR RESISTOR! (Sorry to shout- I burned one of mine out in about .5 seconds!!!)
Resistors and Potentiometers:
There are 3 resistors in there- two to protect the optocoupler and one 1 Mega-ohm resistor in there as a pull-down resistor. (Pull-down resistors leech away the trickle of power that each pin might get to make sure your signals are definitely ON or OFF. Without it- things were acting rather finicky! -I'm hoping I didn't mix that up!)
The potentiometer (variable resistor or "trim pot") became my analog input whose resistance determined the delay in milliseconds between the ON signal and the OFF.
Notes on Cost:
Approximate price list:
$4 Arduino Nano
$1 Optocouplers (2) - (Ignoring the one I fried!)
$.50 Resistors
$.15 trim-pot
$.75 Female headphone jack
$.50 Header (male and female) for Arduino and plugging unused headers for end-user.
$.50 protoboard and solder
$7.45 Total + Several Nights of Frustration & Learning= Priceless
Step 2: Arduino Code
My code for the program was a mash up of several internet searches. Sorry to the contributors for my lack of citations. Thank you to the giant brains that helped me tease something workable from your previous work.
To help me check my resistor values I tried a serial monitor for testing the timing out and (Surprise!) it was super helpful.
// constants won't change. They're used here to // set pin numbers: const int pushButton = 7; // the number of the pushbutton pin const int turnOn = 12; // the number of the LED pin that turns circuit on const int turnOff = 10; // the number of the LED pin that turns circuit off // variables will change: int buttonState = 0; // variable for reading the pushbutton status // int duration = 0; int timerPin = A5; // select the input pin for the potentiometer int sensorValue = 0; // variable to store the value coming from the sensor void setup() { Serial.begin(9600); pinMode(turnOn, OUTPUT); // initialize the LED pin as an output: pinMode(turnOff, OUTPUT); // initialize the LED pin as an output: pinMode(pushButton, INPUT); // initialize the pushbutton pin as an input: pinMode(timerPin, INPUT); } void loop() { //Read Sensors sensorValue = analogRead(timerPin); // read the value from the sensor: buttonState = digitalRead(pushButton); // read the state of the pushbutton value: //Print to Monitor int sensorValue = analogRead(A5)*30;// read the input on analog pin 6: //float voltage = sensorValue * (5.0 / 1023.0); // Convert the analog reading (which goes from 0 - 1023) to a voltage (0 - 5V): Serial.println(sensorValue); // print out the value you read: //Decide and Act if (buttonState == HIGH) // check if the pushbutton is pressed. If it is, the buttonState is HIGH: { digitalWrite(turnOn, HIGH); // turn LED on: delay(500); digitalWrite(turnOn, LOW); // turn LED off: delay(sensorValue); // set delay value to potentiometer value digitalWrite(turnOff, HIGH); // turn LED on: delay(500); digitalWrite(turnOn, LOW); // turn LED off: } else { digitalWrite(turnOn, LOW); // turn LED off: digitalWrite(turnOff, LOW); // turn LED off: } }
Step 3: Final Thoughts and Instructions for End-User
After a couple weeks of late nights I'm super excited to have it working. There are some quick instructions above for sharing if you want.
My next plans are to see if I can:
1. fit it more elegantly on a protoboard
2. add a potentiometer instead of a trim-pot. (No screwdriver required.)
3. more robust packaging
4. other changes based on feedback from therapist and families.
Probably not but maybe:
5. Some means of visual for the delay time. (Not needed but perhaps a good next learning step for me.)
Thanks for reading and best of luck if you are inspired to try something similar!
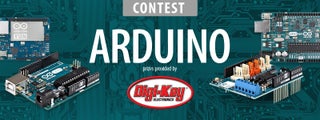
Participated in the
Arduino Contest 2016