Introduction: All in One AVR Programmer
There is a lot of information on how to upload programs to AVR microcontrollers, but I have never been able to find a place that has everything I need. This is my attempt at creating an Arduino shield to program the ATtiny85, the ATtiny84, and burn the bootloader to an ATmega328. It includes the documentation to do everything you may need to do from the Arduino IDE.
You will need:
Arduino (I used an Uno)
Adafruit Proto Shield for Arduino http://www.adafruit.com/product/2077
Extra-long break-away headers http://www.adafruit.com/product/400
Adafruit Perma-Proto Breadboard PCB http://www.adafruit.com/products/1606
2 28-pin ZIF sockets http://www.adafruit.com/product/382
22 gauge hookup wire (I used red, black, green, yellow, and white)
16 MHz Ceramic Resonator http://www.adafruit.com/products/1873
Step 1: Preparing the Shield
Cut three pieces of the short headers that came with the proto shield, one with six pins and two with eight.
Cut one piece of the long headers with four pins. Insert this piece into your Arduino on pins ten through thirteen.
Insert the six and eight pin header pieces into the Arduino.
Set the proto shield on the pins and solder it.
Cut another piece of the short headers with four pins.
Use a pair of pliers to pull the pins out of the plastic insulator.
Press the insulator onto the long pins sticking up on pins ten to thirteen.
Cut three single pin pieces of the short headers.
Solder them into the shield in the holes shown in the second photo, with the insulators on the top side.
Set the Arduino and the shield aside, you won't need them for a while.
Step 2: Wiring the Top Board
Solder the 28 pin ZIF sockets onto the board where shown.
Follow the diagram to solder the wires to the board.
Notice the points where a green wire meets a yellow wire in the same hole.
Two wires are soldered into the same hole. With 22 gauge wire they will barely fit.
The dark spots at holes J28-J31, G32, and in the bottom ground rail at points 18 and 41 are where the top board will mount to the shield. Don't do that yet.
Step 3: The Ceramic Resonator
Cut a piece form one of the female headers that came with the shield, with three pins. This is the socket for the ceramic resonator.
Leave enough extra so you can sand the cut edges smooth.
Solder the three pin header into F-33 through F-35.
Solder the white wires for the ceramic resonator.
There are two wires in holes J-18 and J-19.
You may need to tin the contacts on the ceramic resonator so it will make good contact in the socket.
Step 4: Putting It Together
Place the top board on the shield, make sure the long pins in the shield are in holes J28 through J31.
Solder all seven connections between the shield and the top board.
Step 5: Adding Chip Definitions to the Arduino IDE
You will need to add the chip definitions to the Arduino IDE.
Download the Attiny definitions here: https://github.com/damellis/attiny
Unzip the file, open the folder, and copy the attiny folder into the hardware folder in your sketchbook folder.
If you don't have a hardware folder in your sketchbook folder create one.
.
Open the ~/sketchbook/hardware/attiny folder and edit the boards.txt file.
Add the following lines at the bottom of the file:
##############################################################
# ATmega328 on a breadboard (8 MHz internal clock) ##############################################################
atmega328bb.name=ATmega328 on a breadboard (8 MHz internal clock)
atmega328bb.upload.protocol=stk500 atmega328bb.upload.maximum_size=30720 atmega328bb.upload.speed=57600
atmega328bb.bootloader.low_fuses=0xE2 atmega328bb.bootloader.high_fuses=0xDA atmega328bb.bootloader.extended_fuses=0x05 atmega328bb.bootloader.path=arduino:atmega atmega328bb.bootloader.file=ATmegaBOOT_168_atmega328_pro_8MHz.hex atmega328bb.bootloader.unlock_bits=0x3F atmega328bb.bootloader.lock_bits=0x0Fatmega328bb.build.mcu=atmega328p atmega328bb.build.f_cpu=8000000L atmega328bb.build.core=arduino:arduino atmega328bb.build.variant=arduino:standard
The chip definitions you need will now appear in the Tools=>Board menu in the Arduino IDE.
Step 6: Programming the Attiny84/85
Start the Arduino IDE, click on Tools and open the Board menu.
Select your Arduino board. (I am using an Uno.)
Click on Tools again and this time open the Programmer menu.
Select the AVRISP mkII programmer.
Click on File, open the Examples menu, and click on ArduinoISP.
To program an Attiny85 place the chip in the back of the socket on the left as in the first picture. The alignment dot faces the handle.
To program an Attiny84 place the chip in the front of the socket on the left as in the second picture. The alignment notch faces the handle.
I will be giving instructions for programming the Attiny85. If you are programming an Attiny84 everything works the same. Just use the Attiny84 board definition instead.
Click on Tools, open the Programmer menu and select Arduino as ISP.
Click on Tools again and this time open the Board menu.
You will see a bunch of new boards listed.
There are three listings for the Attiny85 chip:
- Attiny85 (internal 1 MHz clock)
- Attiny85 (internal 8 MHz clock)
- Attiny85 (external 20 MHz clock)
The default setting is Attiny85 (internal 1 MHz clock).
Use the 1 MHz setting if you are operating the chip at low voltage or if you require minimum power usage. The Attiny85 can run on as little as 2.7 volts, 1.8 volts for the Attiny85V.
The Attiny85 (internal 8 MHz clock) is the most used setting for the chip.
Use the Attiny85 (external 20 MHz clock) setting only if you need the speed. The crystal is required and uses up two digital pins. Once the chip is set up to use the external clock it will not run without it.
Loading your program is actually a two step process. First you run the Burn Bootloader option under Tools then you load your program. On Attiny chips the Burn Bootloader option does not actually burn a bootloader. It only sets the internal fuses to set the clock speed.
If you are using the external clock the frequency of the ceramic resonator does not matter when you are burning the boot loader or loading the program. The chip runs at the frequency of the ceramic resonator or crystal. The frequency of the ceramic resonator or crystal must be 20 MHz when you remove the chip from the programmer and put it in your project. This is because a program written in the Arduino IDE will autocratically synchronize the timer interrupts to the 20 MHz clock speed.
After you decide on a clock speed click on Tools, then open the Boards menu to select the board and clock speed.
Click on tools again and then click on Burn Bootloader.
Now you can load your program into the Arduino IDE and click the upload button to load your program.
.
If you would like to see what happens if the clock speed and the timer interrupts are not synchronized burn the boot loader at 1 MHz. Load a blink program that blinks an LED 1 second on and one second off, but select the 8 MHz option. You will notice that the LED blinks much slower.
Step 7: ATmega328: Burning the Bootloader
Start the Arduino IDE, click on Tools and open the Board menu.
Select your Arduino board. (I am using an Uno.)
Click on Tools again and this time open the Programmer menu.
Select the AVRISP mkII programmer.
Click on File, open the Examples menu, and click on ArduinoISP.
Place the chip in the socket on the right with the alignment notch facing the handle.
Click on Tools, open the Programmer menu and select Arduino as ISP.
Click on Tools again and this time open the Board menu.
Select either Arduino Duemilanove w/ATmega328 or ATmega328 on a breadboard (8 MHz internal clock).
If you select Duemilanove w/ATmega328 insert the ceramic resonator.
If you select ATmega328 on a breadboard (8 MHz internal clock) for a chip that already has the standard bootloader you need to insert the ceramic resonator. A chip set to run with the external clock will not run without it.
Now click on Tools, then click on Burn Bootloader.
In my testing I found that after programming Attinys I had to restart the computer, unplug the Arduino, and start this procedure from the beginning to burn a bootloader. Try this if you get errors when trying to burn a boot loader.
I think this programmer will also work on an ATmega168, but I don't have a chip to try it on.
There are instructions on how to program the ATmega328 once the bootloader has been loaded here. But I find it is easier just put the chip in the Arduino since you need to pull the chip from the Arduino anyway. For a standard bootloader use the board setting you would normally use. If you burned the bootloader with the ATmega328 on a breadboard (8 MHz internal clock) use it again when programming the chip.
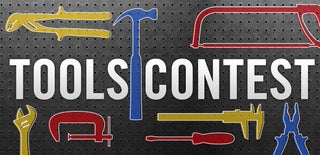
Participated in the
Tools Contest