Introduction: Arduino Computer Control
This instructable might seem a bit brief, but there isn't so much to say. I will be showing you the code needed to control the LED on an Arduino board, using a Visual Basic application. If you just want it as a demo, rather than editing the code, there is a zip file attached with the application I wrote in it. Just unzip, and run setup to install it.
Attachments
Step 1: Arduino Code
Here is the code for the Arduino, I assume you know how to use it. if not, the arduino website has a good reference section. This code can be modified to control a number of things. To control an output on a different pin, change the value of int ledPin = ?;. To control more than one thing, add another pin and send C or D as well as A and B etc. You can also change the function of receiving the letter by changing the code in the if statement.
int ledPin = 13;
int incomingByte;
void setup() {
Serial.begin(19200);
pinMode(13, OUTPUT);
}
void loop(){
if (Serial.available() > 0) {
incomingByte = Serial.read();
if (incomingByte == 'A') {
digitalWrite(13, HIGH);
}
if (incomingByte == 'B') {
digitalWrite(13, LOW);
}
}
}
Some or all of the code in this instructable may have come from another source, and if so, thank you very much, but I honestly can't remember. I have put it here more to show the sections needed and how they can be modified, rather than to steal other peoples work.
Step 2: Visual Basic
I don't have the space to explain how to use Visual basic , but I am 15, and I've been using it for years, so anyone can use it. This is the code you will need for the windows form application. Create two buttons, and leave them with their default names. Then, create a Serialport.
Imports System.IO
Imports System.IO.Ports
Imports System.Threading
Public Class Form1
Shared _continue As Boolean
Shared _serialPort As SerialPort
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
With SerialPort1
.Close()
.PortName = "COM4"
.BaudRate = 19200
.Parity = Parity.None
.DataBits = 8
.StopBits = StopBits.One
.DtrEnable = True
.RtsEnable = True
.ReceivedBytesThreshold = 1
.Open()
End With
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
SerialPort1.Write("A")
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
SerialPort1.Write("B")
End Sub
End Class
Step 3: Test!
Once you have uploaded the sketch to the arduino, and built the application, or opened mine, you should be able to control the LED. here is a video showing my setup:
http://www.youtube.com/watch?v=bL2LGQBHYxY
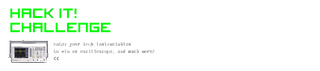
Participated in the
Hack It! Challenge