Introduction: Beginning Arduino: Delay Without Delay(), Multiple Times
A while back I did an instructable where I used millis() instead of delay() to demonstrate how to time an event and do something else at the same time. This is not possible when using delay() because it stops the program from doing anything during the delay. Two people have asked for an instructable using multiple timed events with different lengths running at the same time. So here it is.
There are four LEDs attached to four digital pins. Each has a timed interval two times greater than the previous LED to create a binary counter.
This is also part of my Beginning Arduino collection, available here:
Step 1: LED and Resistor
This is optional, but I found that having a bunch of these made up makes breadboarding a lot easier.
Solder a resistor to the cathode lead of some LEDs. The cathode lead is the shorter negative (ground) lead. 270 - 560 ohms works good for on the RaspberryPi, for an Arduino use 330 - 680 ohm resistors.
I usually make them with 470 - 560 ohm resistors so they will work with both.
Step 2: Build the Circuit
Build the circuit as shown in the diagram above.
Step 3: The Code
Copy this code into the Arduino IDE and upload it to your Arduino:
/********************************************************* * Demonstration using millis() instead of delay() so * another activity can happen within the delay. * * The anode of four LEDs are connected to pins 2 - 5. * The cathodes are connected to ground through resistors. * * LEDs are timed to make a binary counter. * *********************************************************/ unsigned long time[4]; //Holds time for each LED. int pin[8] = {2.3.4.5}; //Pin numbers for LEDs. int toggle[8] = {0,0,0,0}; //Toggles for LEDs. (0 or 1) /********************************************** * setup() function **********************************************/ void setup() { for(int i=0;i<4;i++) { pinMode(pin[i], OUTPUT); //Set LED pins as output. digitalWrite(pin[i], LOW); //All LEDs are off at start. time[i] = millis(); //Start timers for all LEDs. } } /********************************************** * loop() function **********************************************/ void loop() { int interval[4]={1000,2000,4000,8000}; for(int i=0;i<4;i++) //For each LED in turn. { if(millis()-time[i] > interval[i]) //Has time passed interval? { toggle[i] = !toggle[i]; //If so not toggle, time[i] = millis(); //reset time, digitalWrite(pin[i], toggle[i]); //and toggle LED, } } }
Attachments
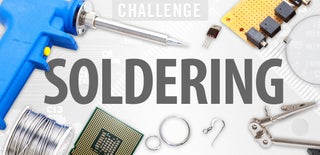
Participated in the
Soldering Challenge