Introduction: Create Your Own 2D Video Game!
Okay, so if your anything like me you've probably always dreamed of creating your very own video game. You might be surprised how easy it actually is to get started. I hope to explain this so that everyone from absolute beginner to an experienced programmer can create there own game. I will be showing how to create a game using the XNA Game Studio© and Microsoft Visual C# 2010©. You can download the XNA Game Studio© HERE and Microsoft Visual C# 2010© HERE. For this instructable we will be using a 2d platformer starter kit to learn the basics, and then finishing a few incomplete features as well as customizing it as you like! You can download my edited distribution of the starter kit on my website unleashedinteractivestudios.pcriot.com.
Please note that most of the code, images, and sounds in the starter kit are not mine!
Or you can also download the original starter kit HERE. You may notice that the starter kit is also for creating games on Xbox 360 and Windows phones, however I removed this in my version because I have no way of testing this. (Also, there are tons of restrictions on making games for the Xbox 360.)
Step 1: 1. Basics
Opening the Project
After you have installed both the XNA Game Studios and Microsoft Visual C# 2010 Express© go inside the starter kit folder and double click the file named ‘Platformer (Windows).sln’. If it asks you what it should open with select Microsoft Visual C# 2010 Express©.
Debugging
Since we’re using a starter kit, as soon as you open the project you can test it! You can do this by clicking on the green arrow at the top of the screen or by pressing F5. As you can see the game is not complete.
Step 2: 2.LEVELS
Creating new Levels
Instead of getting overwhelmed by all the code lets jump to the fun part.
1.create a new text file and name it 4.txt or a number one higher than the current highest level.
2.look at the level files in the starter kit to see what they should look like.
3. use the block guide below to customize your level!
Blocks
'.' = Blank space
'X' = Exit
'G' = Gem
'-' = Floating platform
'A' = Enemy
'B' = Enemy
'C' = Enemy
'D' = Enemy
'~' = platform block
':' = Passable block
'1' = player starting point
'#' = impassable block
4.Make sure your level has both a starting point '1' and an exit 'X' or else you will get an error and freeze the game. You can also change the size of the level by adding more lines or making each line longer however, each line MUST be the same length. NOTE: making the level longer will likely require you to adjust you background image size and require you to adjust the window size to make everything fit!
(I will talk more on this towards the end)
Importing Levels
1. In the 'Solution Explorer' tab, expand the 'CONTENT' Group then Right click 'LEVELS'.
2. Click 'ADD > Exsisting Item' Select the new Level file named 1.txt,2.txt,3.txt, etc.
Step 3: 3. Backgrounds & Resources
Importing Backgrounds
Each level needs its own background. each background is made of 3 layers. for example, if you create a forth level you will need 3 new PNG images named Layer3_0, Layer3_1, and Layer3_2. the first number represents the level (level 1 starts at 0) and the second number represents the 3 layers (first layer starts at 0)
1.In the 'Solution Explorer' tab, expand the 'CONTENT' Group then Right click 'BACKGROUNDS'.
2.Click 'ADD > Existing Item' Select the new Backgrounds named Layer3_0.png Layer3_1.png Layer3_2.png or something similar depending on which level the background is for.
3.
You will then have to change this line of code towards the beginning of Platformergame.cs
// Change this to the number of levels in your game
private const int numberOfLevels = 3;
(change the number to the number of levels in your game)
Resources
Before we start with the code, lets take a look at adding your own characters, animations, Tiles, sounds, and more! Right now we will just be replacing the resources already imported into the game; so this is actually quite simple. (later on we will import new objects into the game.) Before we begin I recommend downloading Gimp (for images) and Audacity (for Audio).
1.locate your content folder. (if you saved your starter kit to ‘my documents’ it should be as follows.)
C:\Users\YOUR_NAME\Documents\Instuctables_2d_Platformer\Platformer\Content
2. Lets start by making a new character. Inside the content folder go to sprites>player. This is where all of the textures are for the character you will control. You will need Gimp for this or another image editing software that supports transparency. Start by opening Run.png. Notice that this is the animation for your character to run. There are 10 frames for this animation and 11 for the others (not sure why this is) In other words, there should be 10 or 11 pictures of your character (64px by 64px) side by side that when run together look as if the character is running (or what ever the animation is for.) you should then do the same thing for the other pictures in the folder. (Monsters are the same way) I am not a very artistic person, so I am unable to give a more detailed look into creating animations.
3.Next lets move on to the tiles. These are the blocks characters and monsters will walk on.
They are located here:
C:\Users\YOUR_NAME\Documents\Instuctables_2d_Platformer\Platformer\Content\Tiles
Blocks textures A0 through A6 and B0 through B1 will will alternate when there corresponding block is placed. All of the Tiles are pretty easy to change because there are no animations however, I still recommend using Gimp because The Exit Texture requires transparency to look right. The Overlay textures are the same way.
4. Lastly we have the sounds. Now I am not a musician but audacity made it pretty easy to at least make some sound effects. As for the music that will play in the background, i used a virtual piano program and then edited with audacity.
All of the Resources I created are in the folder named Extras
Step 4: 4. Coding
Coding
Okay, now its time for the slightly more boring part. Before we change the settings so on the side bar the line numbers are shown. This will help you to follow along.
Click on Tools > Options then at the bottom select ‘show all options’. Next, click Text Editor > All languages and under display select Line Numbers. Click OK.
Lets start with a few
easy customizations in the code.
Line 204 string timeString = "TIME: " + level.TimeRemaining.Minutes.ToString("00") + ":" + level.TimeRemaining.Seconds.ToString("00");
You can change ‘TIME:’ to any thing else that will be displayed before the remaining time.
Line 210 timeColor = Color.Yellow;
You can change ‘Yellow’ to any color (this changes time font color)
Line 214 timeColor = Color.Red;
You can change ‘Red’ to any color (this changes time font color when Warning time is reached)
Line 43 private static readonly TimeSpan WarningTime = TimeSpan.FromSeconds(30);
You can change ‘30’ to the number of seconds remaining until time starts blinking
Line 220 DrawShadowedString(hudFont, "SCORE: " + level.Score.ToString(), hudLocation + new Vector2(0.0f, timeHeight * 1.2f), Color.Yellow);
You can change ‘SCORE:’ to any thing else that will be displayed before the remaining time.
You can change ‘Yellow’ to any color to change score font color.
2.If you remember, in the beginning i mentioned something about making your game Full Screen. The problem is, because i want to keep the code as simple as possible so to do this you will need to change the background image size and the size of your level.
First, the Code:
Put this after Content.RootDirectory = "Content"; on line 59
this.graphics.PreferredBackBufferWidth = 48;
this.graphics.PreferredBackBufferHeight = 8;
this.graphics.IsFullScreen = true;
Second, for the Background I used a resolution of 1600x900px (You can play around with the size if you want)
Third, for the level size mine is 23Lines by 33Collumns.
You may also want to change the background color of the screen not covered by your image.
Line 184 graphics.GraphicsDevice.Clear(Color.CornflowerBlue); Change ‘CornflowerBlue’ to any other color. 3. If You made your game full screen you probably want a way to exit, Right? Just add the following code after Line 133. if (keyboardState.IsKeyDown(Keys.Escape)) Exit();
You can now exit the game by clicking the Escape key.
4.Now lets take a look at Level.cs
Line 98 timeRemaining = TimeSpan.FromMinutes(2.0); You can change the number of minutes given by changing ‘2.0’ (.5 equals 30 seconds) Line 71 private const int PointsPerSecond = 5; You can change ‘5’ to the number of points you want your player to receive per second remaining when you reach the exit. 7.Now lets add a new Monster! Add the following code after line 208 in level.cs:
case 'S':
return LoadEnemyTile(x, y, "MonsterS");
You can add this to your level with ‘S’ But first in the solution explorer panel, right click ‘Sprites’ select add new and then folder. Then in our case you will name it MonsterS, and then import new animations like we did earlier. NOTE: If you would like me to right a tutorial on customizing Monsters please say so in the reviews. 8. Now lets add some new blocks. (Very similar to Monsters)Add the following code after line 226 in level.cs
// passable block
case 'V':
return LoadVarietyTile("BlockV", 1, TileCollision.Passable);
You can change ’V’ to any other letter or symbol not currently used to place anything in to levels. You can also change ‘BlockV’ to any not used, but remember ‘BlockV’ is the name of the Png file that should be placed in your ‘Tiles’ folder. ‘1’ is the number of frames used for your block. You can also change ‘Passable’ to ‘impassable’ to decide of the player or enemys can travel through the block. Lastly be sure to import the png image back into the tiles folder the same way we did before. Okay, before we go on there are two important features left out of the game; however I was unable to find an ideal solution to incooperate these features. Those features are, making the score carryover between levels, and making the player start over when time runs out (or dies). I will be looking to fix this, but if anyone finds am ideal way before me please share! (I will give you credit)
Step 5: Finishing Touches!
ALMOST DONE!
1. One last thing to do before you publish your game is to test out your game (if you forgot how just click the green arrow or press F5). You should make sure you can play through your whole game with out errors and make sure your happy with it. 2. It’s time to publish your very own game! Click Project>Publish Platformer (Windows) select where to publish your game. 3.Now click on your application, let it install, and Play! Congratulations! You Just created you own video game.
Other Stuff
-If Somethings not working PLEASE let me no and I try to help as best I can.
-If you would like me to create another instructable for adding more advanced features please leave a comment. -Please see the document I left in my version of the starter kit (Just in case there are any typos in the code here they should be correct in the text document)
Legal Stuff -I am NOT associated in any way with Microsoft. XNA Game Studio 4.0 and Microsoft Visual C# 2010 Express Edition are trademarks or copyright of Microsoft. The 2d Platformer Starter kit is also copyrighted by Microsoft including my version (as far as I know) I have only added my textures, animations, sounds, etc. Which are copyrighted by me ( and my website unleashedinteractivestudios.pcriot.com) I am also not associated with any other program used in this instructable. Credits This Instructable was created by (me) Tony Cicero
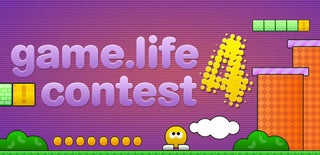
Participated in the
Game.Life 4 Contest