Introduction: Interfacing BMP180 ( Barometric Pressure Sensor) With Arduino
The BMP-180 is a digital Barometric Pressure sensor with an i2c interface. This tiny sensor from Bosch is quite handy for it's small size, low power consumption and high accuracy.
Depending on how we interpret the sensor readings, we could monitor changes in weather, measure relative altitude or even find the vertical speed(rise/fall) of an object.
So for this instructable, I'll be focusing on just getting the sensor to work with Arduino.
Step 1: A Bit of History on Barometers: the Pressure Is On!
Barometers measure the absolute pressure of the air around it. The pressure varies depending on weather and altitude. The usage of the barometer to predict storms has been going on since the 17th century. Back then barometers were long glass rods filled with liquid mercury. And hence came the unit of 'mercury pressure'.
In just a couple decades, the instrument became a real handy item. Everyone had them, from professional scientists and sea faring men to amateurs. They noticed that a sudden change in air pressure would lead to a 'foul weather'. These forecasts were nowhere near accurate, until the mid 18th century when gradually a detailed forecast table was developed. If you're interested about the history of barometers and how to do weather forecasts from the values, feel free to check out this link.
Other than meteorological observations, another novel use for the barometric pressure sensor is to calculate the relative altitude of a place. Now this is where things get interesting.
Remember the formula, (P = h * rho * g) from physics class? Turns out we can calculate the relative altitude of a place using the BMP-180. Neat, huh?
Step 2: Gather the Equipment!
Time to get back to the 21st century. Now that we had a 'very' important history lesson on barometers, let's get back to the list of items we need for this inscrutable.
1. Breadboard and jumpers
2. BMP-180
3. Any Arduino board. (I'm using an Arduino Pro Micro, but any arduino board will suffice)
4. A USB cable and a computer that can run the Arduino IDE
Step 3: Wiring It Up!
Since the BMP-180 runs on a i2c interface, it's a breeze to connect it. Depending on what Arduino board you are using, find the two i2c pins.
Board ----------------------------------->I2C / TWI pins
Uno, Ethernet, Pro mini ----------------->A4 (SDA), A5 (SCL)
Mega2560 ----------------------------->20 (SDA), 21 (SCL)
Leonardo, Pro Micro -------------------->2 (SDA), 3 (SCL)
Due ------------------------------------>20 (SDA), 21 (SCL), SDA1, SCL1
For the VCC pin, make sure to check if your sensor is 5v tolerant or not. If it is not, just power it up to 3.3v. The breakout board that I am using has a built in 3.3v regulator which makes it 5v tolerant.
So my circuit connections are something like this:
Arduino -> BMP-180
D2 (SDA) -> SDA
D3(SCL) -> SCL
5v -> VCC
GND -> GND
Things that can go wrong in this step:
1. Double check the VCC and GND lines before powering it up. You might damage the sensor.
2. SDA --> SDA and SCL --> SCL, don't mix them up.
Step 4: Choosing the Right Library
Now to choose a library to make our life easier with the BMP-180. Inspite of being such a nifty sensor, there is alot of complicated math involved to use it properly. Calculations such as conversion from units of pressure to correcting sea level pressure... It certainly makes things harder for someone who skipped to many physics classes to begin with.... :(
The solution? Libraries!
So far I have used 3 different libraries for the BMP180.
1. The sparkfun BMP180 library
2. The Adafruit BME085 API (v1) (i'll be using this one for this instructable)
3. The Adafruit BME085 API (v2)
The reason why I am linking all three libraries is because each of them has it's pros and cons. If you just want to get the job done, the Adafruit libraries are great. They're easy to use and come with very nice documentation. On the other hand, the sparkfun library provides alot of additional learning as you'll have to do alot of the calculations manually. If you're interested in that, check out this amazing tutorial from sparkfun.
Step 5: Downloading the Arduino Library
Download the Library from this link.
You should have a zip file.
Step 6: Installing the Arduino Library
To install the Library you just downloaded,
Go to 'Sketches' -> 'Include library' -> 'Add a zip library'
Then locate the zip file you downloaded and open it.
Voila, you should have the Library installed.
Step 7: Running the Example Code
To check if everything is working correctly so far, let's run the example code provided with the library.
Navigate to 'Files' -> 'Examples' -> 'Adafruit BMP085 Library' and open 'BMP085 Test'
Plug in your arduino, check your COM port and upload!
After the the code is successfully uploaded, open the Serial Monitor to check the sensor readings.
Things that can go wrong in this step:
1. If you cant find the Adafruit BMP085 listed on examples, the library didn't install properly. Go back and check again.
2. If your code doesn't upload, check your COM port or your USB connection
3. If you get an error message on the serial monitor, recheck your circuit connections.
Step 8: Figuring Out the Code
The sample code very much self explanatory, but just to be sure let's dig into it a bit.
The very first thing we need to do is to include the Wire.h library to get the i2c interface on the Arduino working. Then we include the Adafruit BMP085 library and create a sensor object. (bmp in this case)
#include <Wire.h> #include <Adafruit_BMP085.h> Adafruit_BMP085 bmp;
The bmp.begin() function returns a boolean value depending if the i2c interface detects the BMP-180. It is run once in setup() to check if the device is communicating or not.
void setup() {
Serial.begin(9600); if (!bmp.begin()) { Serial.println("Could not find a valid BMP085 sensor, check wiring!"); while (1) {} } }
Now for the basic functions, we have
1. bmp.readTemperature()
2. bmp.readPressure()
3. bmp.readAltitude()
4. bmp.readSealevelPressure()
here, bmp being the sensor object we created. Feel free to pick whatever variable name you can think of.
Now notice that readAltitude() can accept an integer as a parameter. This is to get a more precise measurement of altitude if you know the current sea level pressure. Now this will vary with weather and geographical position of where the reading is being taken at. Make sure to convert the Sea level pressure in Pascals and pass to this function.
Attachments
Step 9: Bonus Step! (slow Claps)
So I've worked with the BMP180 sensor for a long time now and here are some tips
1. Don't enclose the sensor completely. If you need to build a housing for it, make sure there's sufficient airflow. Since the sensor measures ambient air pressure, it needs to be exposed to open air.
2. The sensor starts acting up crazy when the altitude gets high. I had the luxury of sending a BMP-180 to 32,450m using a weather balloon. The plot above shows GPS altitude data vs BMP-180 altitude data. At lower altitudes, the BMP-180 is much more reliable as it is harder for GPS to get a lock. But at higher altitudes, the GPS data becomes more accurate as air pressure quickly falls above 9,000m.
3. The sensor data fluctuates on direct sunlight. I was a bit too late to notice that on the data sheet for a project.
4. The i2c address on the BMP180 is 0x77. And it is fixed, making it impossible to add multiple sensors to the same i2c bus.
So that's about all on the BMP-180. This is my first instructable (I hope those GIFs are working!!). Feel free to ask more questions.
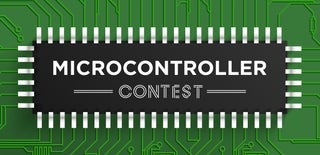
Participated in the
Microcontroller Contest