Introduction: LinkIt ONE - Communication With Python
Hi fellow makers!
Mediatek's new board, LinkIt ONE offers great potential - GPS, WiFi, Bluetooth, GSM, extensibility with gpio, strong processor and more. But sometimes you still need the comfort of python for parsing files or drawing graphs. Even if you haven't read this 'Ible's title you now already know what it's going to be about: making LinkIt ONE communicate with python running on PC. Now let's get started!
Step 1: Installing Software
Aside from you will also need PySerial library, which can be obtained here. Which version to choose depends on which Python you are running. For purpose of this Ible I willll be using the newest python, in my case version 3.5, but you can use any version you are comfortable working with.
You can obtain latest version of Python here and compatible PySerial library here. Installation is straight forward but you will also need to have LinkIt ONE drivers installed for computer to recognize the device.
Step 2: The Code
Two things are left to be done. The first is to upload code for parsing commands and doing whatever you want done to LinkIt ONE and the other one is to write Python script for sending and receiving data.
I won't dive into explaining first part as it is a standard Arduino type of program. It listens to serial inputs until it detects a carriage return character ("\r") and stores string into a variable which can then be used to decide what to do or used as data for function. Check attached "serialResponse.ino" code.
The second part, Python code, Isn't any more difficult but has a few tricks you should know about.
import serial
That is the first thing you have to do - it imports serial library into Python script
ser=serial.Serial(7)
This part of code stores reference to port you want to be using in "ser" variable for ease of use. Note that this opens COM8 port. As with all programming, first element on list has index 0. For using this script with LinkIt ONE you will want to open COM8 most of the time but if you aren't sure, you can check which port you've opened, you can do it lie that:
ser.name
Note this is not a function but a variable, meaning it has no parentheses.
And now to writing on serial port.
ser.write("text".encode())
This function takes one parameter, which must be a string. You can replace "text" with whatever string or variable (that contains string) but you must call .encode() function on it or it will not work.
And now two methods of reading data, received through port.
ser.read()
This function returns first character available. Useful but if you want to get a word you must join characters in a string. Python does this itself if you call following function:
ser.readline()
This function listens for data on serial bus up to first newline character ('\n'). Be careful as program will hang up if no newline character is received.
That is everything you need to know to make any extension for LinkIt ONE board with Python. Check example code for reference.
Step 3: Example of Use
As an example of how this can be used, take a look at attached code which converts your LinkIt ONE board in GSM modem for sending SMS. Upload code on LinkIt ONE, insert SIM card (must not be pin-locked), connect GSM antenna to appropriate connector, connect LinkIt ONE to PC via USB cable (make sure switches are on UART and USB) and run python code to see how this works. The code is very simple to understand as it is almost the same as one from last step.
Python sends two strings - firs one is recipient's phone number and the other one is SMS content. LinkIt ONE parses both strings and sends SMS. Check code to see how that is done.
One thing you have to be careful about is not to try to send too long SMS as that will not work.
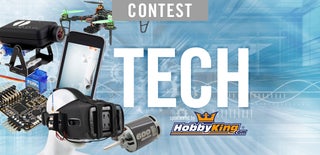
Participated in the
Tech Contest