Introduction: Making Your First Game on a 3DS With SmileBASIC
Video games. Ah, the fun things we play on computers and consoles. I've played video games since I was five years old. I've always been interested in playing them. One day, I thought, "How do people make them?" Well, that's what you must be here for. They put in code that tells the computer what to do and how to react to user inputs. So you think, "What do I have to put in to make a game?" Well, we'll go over that. I thought using SmileBASIC would be a good start for beginners. It's made to get people into programming. It's pretty simple too. So let's get started on coding.
Step 1: Hello World
I think it would be best to start with something simple so we can get a good start. The first thing you'll do is tap where it says, "Create Programs with SmileBASIC." Then, tap the blue button that says, "EDIT." So let's make it say something. This is the code, I'll explain what it does:
LOCATE 1,1 PRINT "Hello World!"
What the command, "PRINT" does is it "prints" whatever is in the quotes. "LOCATE" puts the text in the position you specify. "LOCATE 1,1" will put the text one line down, and one character width across. You can also print variables by using something like this:
PRINT X
Step 2: Input, Buttons, and Loops
Now I'm going into some of the main things you'll need to make a game. Let's start with input:
CLS INPUT "What is your name";NAME$ PRINT NAME$
Okay, so "CLS" clears the screen of text. "INPUT" lets you type in some text, and what it says after the semicolon is what you typed in(NAME$). You already learned "PRINT."
Buttons are different. Here's another example code:
@LOOP CLS PRINT BUTTON() WAIT 1 GOTO @LOOP
Okay, so I went over BUTTON(), WAIT, and GOTO. "BUTTON()" is equal to what button you are pushing. The way it works is BUTTON() is a number*. "WAIT" is just as it sounds. It waits for as long as you put. "WAIT 1" would wait for 1/60 of a second. "WAIT 60" would wait for one second. The reason it's there is because it would be looping so fast it wouldn't work well. Using the "@" symbol before some text makes your loop. You can name it anything you want, as long as it doesn't have spaces or weird symbols. "GOTO @(name)" does just as it sounds. It "goes to" that line. I hope that wasn't too much to take in. You can mess around with the code to learn just what it does.
*Example: When you press the "A" button, BUTTON() equals 16. By pressing buttons with my code listed above, you can learn what each button value is. An easier way is to remember that you can use #(button name). Example: The "A" button's value is #A. Just like up on the arrow pad is #UP.
Step 3: Move Your Character
Now let's get into some real stuff. We know how to use buttons, put text on the screen, and loop our code so that it doesn't stop. Here's the code:
X=24 Y=12 @GAMELOOP CLS LOCATE X,Y PRINT "0" IF BUTTON()==#UP THEN Y=Y-1 IF BUTTON()==#DOWN THEN Y=Y+1 IF BUTTON()==#LEFT THEN X=X-1 IF BUTTON()==#RIGHT THEN X=X+1 IF X<0 THEN X=0 IF X>48 THEN X=48 IF Y<0 THEN X=0 IF Y>24 THEN Y=24 WAIT 1 GOTO @GAMELOOP
Woah! That's a lot of code! Let's start with "IF" and "THEN." It's just as it says. It means that if the button you're pressing is up, then y will decrease by one every loop. The best way for you to learn is by experimenting with the code.
Step 4: Sprites and Using the Circle Pad
Next, we'll cover using the circle pad, and using sprites. So, just what is a sprite? Well, it is "a computer graphic that may be moved on-screen and otherwise manipulated as a single entity." So, how do we use these? SmileBASIC makes it very simple. "SPSET" will put any sprite you want on the screen. I think it's time for another example
X=184:Y=114 @LOOP CLS STICK OUT SX,SY SPSET 0,0 SPOFS 0,X,Y X=X+SX*2 Y=Y-SY*2 IF X<0 THEN X=0 IF X>383 THEN X=383 IF Y<0 THEN Y=0 IF Y>223 THEN Y=223 WAIT 1 GOTO@LOOP
"STICK OUT SX,SY" makes SX and SY equal to the X and Y position of the circle pad. These variables can be called anything you like, but SX and SX are easy to remember and short. "SPSET 0,0" places the sprite "0" with the definition number of 0. The first number listed is the definition number*. The second is what sprite it is**. "SPOFS" locates the sprite where you want it to be. Since it says, "SPOFS 0,X,Y", that means that it will locate the sprite with the definition number 0 at the x position of X and the y position of Y. "X=X+SX*2" and "Y=Y-SY*2" will add SX times 2 to X, and subtract SY times 2 from Y. This is what moves the sprite's position.
*The definition number is what the sprites reference is. Example: SPOFS 0,X,Y the first number listed is the definition number so that it knows what sprite it's affecting.
**You can see more sprites and their numbers by tapping the little green button at the bottom middle of the screen that says, "SMILE," then tapping where it says, "SPDEF." By changing the second number of SPSET, you change the sprite itself. Example: SPSET 0,2 will place a cherry sprite.
Step 5: Making the Game!
You made a sprite move, but what next? Well let's make a game where you shoot things for points! Here's the code:
ACLS XSCREEN 2'Tap the ? button to see what each command does DISPLAY 0 VISIBLE 1,1,1,1 X=167:Y=197:X=16:BX=X:BY=181:P=-100 @HITENEMY P=P+100 SHOOT=FALSE EX=-16 @ENEMYLOOP EY=RND(80) EX=-16 @MAIN CLS LOCATE 5,1 PRINT "Points ";P STICK OUT CX,CY SPSET 0,1251'The ship SPOFS 0,X,Y X=X+CX*5 IF X<0 THEN X=0 IF X>272 THEN X=272 SPSET 1,1343 SPOFS 1,BX,BY<br>IF SHOOT==FALSE THEN SPHIDE 1 IF SHOOT==TRUE THEN SPSHOW 1 BY=BY-4 IF SHOOT==FALSE && BUTTON(2) AND #A THEN BX=X+16 SHOOT=TRUE BEEP 10 IF BY<-16 THEN SHOOT=FALSE BY=181 'Enemy SPSET 2,1281 SPOFS 2,EX,EY SPROT 2,90 EX=EX+5 IF EX>415 THEN GOTO@ENEMYLOOP 'Collision IF BX>=EY-32 AND BX<=EY-16 AND BY<EY+16 THEN GOTO @HITENEMY WAIT 1 GOTO @MAIN
Alright, "RND()" generates a random number between zero and the number you put in the parenthesis minus one. "BUTTON(2) AND #A" is another way of using buttons. Using "AND" allows the user to be pushing A and any other buttons, and it will still recognize that you are pushing A. Why I put the number 2 in the parenthesis of "BUTTON()" is because using 2 means that when it's tapped it makes BUTTON() equal to the button you are pushing. "SPROT" is the command for rotating sprites(SPROT (definition number),(rotation in degrees)).
You can download this entire code by tapping on the title screen, "Publish/Download Projects," then where it says, "Download(Recive) using Public Key." Then, enter this key: K24PEWD Then you can open it by tapping on the title screen where it says, "Browse Projects," then the file called, "GAME_TUTORIAL."
Step 6: You've Made a Game! But Now What?
So now you know some stuff. You can print lines of text, locate them anywhere you want on the screen, use sprites, use button inputs, use the circle pad, use "if then" statements, and take inputs of text. But you want more? If you have troubles programming in SmileBASIC, smilebasicsource.com is the perfect place! There are lots of experienced programmers who are willing to help you. There are also lots of programs to download there.
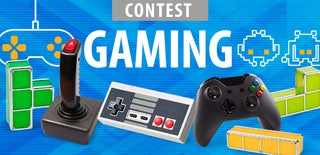
Participated in the
Gaming Contest
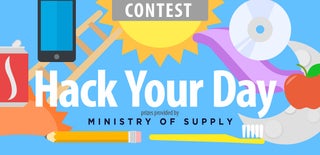
Participated in the
Hack Your Day Contest
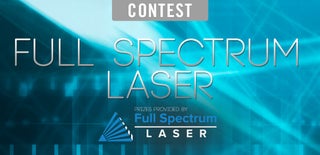
Participated in the
Full Spectrum Laser Contest 2016