Introduction: Name on 7-segment Display Atmel Avr
In this instructable (my first one!) I'd like to show you something I made for a school project. The original assignment was as follows: "In MSVS (or Microsoft visual studio) code in assembly language: Enter your name as an ASCII hex string in the .DATA section of your program. Create a loop and output 1 character at a time in the loop. Hint: NULL terminate your string, use that as a conditional to check for end of string"
Since it was my first time coding in assembly language, it was very scary to see the code mnemonics and try to understand that these short keywords were part of a full instruction set. Getting MSVS set up for assembly language is a very frustrating and complicated task if it is your first time working with it. Every tutroial online seemed to have a different way, linking separate libraries and check this here/that there. I got fed up with trying to compile and run assembly code on MSVS and decided to do something a little different. (I eventually got the machine set up and running they way it should've been.
So, a little background: I have always been fascinated with the arduino and making projects here and there with it. The big world of electronics and hardware just fascinates me so much. I decided that I could do something a little more exciting than just displaying characters to a screen using libraries or APIs to do that for me. I wanted to make something different... I wanted to display my name on a seven segment display, from an Atmel Atmega 328p chip (the chip on arduino uno) strictly in assembly language.
Step 1: Thinking of How a Seven Segment Display Works
So, I've got my project in mind of what I want to do. I wanted to illuminate segments on a 7 segment display in such a way that "letters" would appear on the display. I had knowledge of how a seven segment display works but for those of you who don't...
A seven segment display is basically those "blocky" looking numbers/letters displayed on say a clock or microwave. They're used to display information in a simple fashion. The display is made up of (as the name implies) 7 separate segments which can be illuminated with voltage applied to them. Each segment can be thought of as its own LED, which lights up when power is given to it.
Image from http://www.sentex.ca/~mec1995/tutorial/7seg/7seg.html
Step 2: Creating Letters
We know how a seven segment display works, but how are we going to light the display up in such a fashion to create letters??
Well, I made the diagram posted in my free time, and I apologize that it may be sloppy or hard to see. You can see my design process, placing a '1' where the segment would have to be lighted up and a '0' where we would want the segment to be off. (shame on me for not sticking to the proper 'a,b,c,d,e,f,g' convention of segments but this was off the top of my head) You can see that I have a couple letters left blank or not finished, simply because I was not sure how to display them with the limited segments I was working with.
The '1' signifies the segment being on-or receiving power.
The '0' signifies the segment being off-or not receiving power.
Well, onto the next step: Coding this bad boy up!!
Step 3: Coding Up
I created this source code in AtmelStudio. First, I'd like to link to another instructable which helped me get familiar with outputting "voltages" to pins on the atmega chip.
This instructable here: https://www.instructables.com/id/Command-Line-Assem...
That instructable is absolutely awesome! It let me understand how the physical pins of the atmega chip were controlled from assembly language. While that instructable uses Port B for its outputs, I needed more than only 6 pins. I needed to utilize 7-8 pins. So, for my code, i used Port D, allowing me to use 8 pins for output/input (theoretically)
Step 4: Hooking It Up/ Looking(a Little) More Closely at the Code
From the picture, what I did next was hook my pins from the 7 segment display to resistors and then to the Port D pins of the atmega328p (pins 0-7 on the arduino) I hooked these pins up according to my 'ones and zero' table.
For a quick example, If we wanted to light up 'A' on the display, we would have to look at my chart. We need (according to my drawing/interpretation) to light up pins a,b,c,d,e,f and not g,h... so, based on my pin arrangement my binary string would be: 0b11111100
0b: tells the code that what follows is in binary format
11111100: is the bits that we want to illuminate. Again, '1' being 'on' and '0' being 'off'
The easiest way to think about this is that from right to left (Using Port D) the '1' and '0' complelty match up with pins 0-7 being with-'1' or without-'0' voltage on them. So, loading 0b11111100 on PortD directly turns 'on' pins 2,3,4,5,6,7 on the arduino while keeping 0,1 off.
You'll see at the end of my assembly code there is a mass of "ugly" code, just compares and branches to other functions. These functions are just to place the proper 8 bit sequence of ones and zeros onto the output register to be pushed to Port D based on the ASCII Hex value given.
Step 5: Final Thoughts
While I did not expect this instructable to be an exact "How-to," I wanted to give a little information rather than just posting pictures and short explanations.
I want to use this first instructable to get use to the instructable system and receive some feedback on my code/ design process. Thank you all very much and here's a video of it in its final stage!
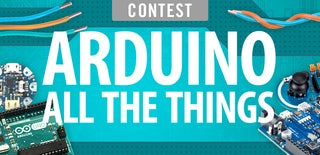
Participated in the
Arduino All The Things! Contest