Introduction: Solid State Optical Sump Pump Controller
Recently I found out my dad had an inconvenient situation with the sump pump in his basement. The sump pump sits at the bottom of a four foot deep well and is activated by a small float that moves up and down. The float is connected to a mechanical switch on the pump housing.
During the wet season, the pump turns on and off about once a minute and the switch contact lasts only about a year before succumbing to mechanical failure. When it does, my dad has to undo the outlet hose, hoist the pump out of the well and replace it with a spare pump he keeps around for the task. Then, he replaces the switch contactor on the broken pump so he can have it ready for next year. This costs about $40 a year, not to mention the labor involved in swapping out the pumps and replacing the contactor each time.
To solve the problem, I set out to design a solid state optical sump pump controller that has no moving parts to wear down. My idea was to bypass the mechanical float on the pump itself and to instead make a new float that would mount to the side of the well. This would consist of a plastic bottle and a dowel rod. The dowel rod would move up and down and trigger two optical sensors mounted on the side of the well. I would then build a circuit to turn the pump on and off using a solid state relay. Because this would be contactless and have no electromechanical parts, it would never fail due to wear.
Supplies
Float Assembly:
- One plastic bottle
- One dowel rod
Optical Float Level Sensor:
- Two Photointerrupters
- Hookup wire
Option 1: TTL Based Control Logic:
- Two 2.2kΩ resistors
- One 100Ω resistor
- One 7400 quad-NAND gate integrated circuit
- One 180Ω resistor (optional)
- One indicator LED (optional)
- One protoboard PCB
Option 2: Arduino Based Control Logic
- One 100Ω resistor
- One Arduino Uno (an ATTiny based board could work as well)
Power Supply:
- One 5V USB charger
- One USB cable
Pump Relay Assembly:
- One solid state relay
- One AC plug
- One AC outlet
Step 1: Making the Optical Water Level Sensor
The water level sensor consists of a shaft with a central hole slightly larger than the dowel rod. When mounted to the side of the well using the attachment bracket, it allows the float and dowel rod to slide freely up and down along with the water level in the well.
At two points along this shaft are positioned photointerrupters. These devices consist of a infrared light source and a light detector. When the dowel rod slides into the groove in the photointerrupter, it interrupts the light beam, turning off the current through the light detector. The photointerrupter sit well above the water level and are not intended to get wet.
I chose to make the water level sensor shaft out of 3D printed segments so that I could easily adjust the length by adding segments as needed. Each segment has a central cutout for inserting a photointerrupter, but only two of the segments will have one.
I also made a bracket for attaching the sensor to the side of the well.
Step 2: Wiring and Operation of the Water Level Sensor
Along the sensor bar, there will be photointerrupters that will be blocked by the dowel rod when the water reaches the low and high point. One side of each photointerrupter contains an LED light source. Both of these will be wired from 5V, in series with a current limiting resistor (shown in the schematic), down through a common ground.
The side of the photointerrupter with a dot contains a light sensitive transistor. The signal lines go to the leads nearest the dot, while the opposite leads are wired to a common ground.
The control logic will pull the two signal lines up to 5V while the phototransistor is in shadow, but if light falls on the transistor, it will conduct and bring the signal line to 0V; therefore, when the dowel rod is present, the signal line will read 5V, while when it is absent, it will read 0V.
I used an ITR9608-F photointerrupter because it has a 5mm gap, which is just wide enough to let my dowel rod through.
Attachments
Step 3: Making a Float With an Adjustable Rod
For the float, I used a small plastic bottle and the dowel rod. I wanted the length of the rod to be adjustable, so I drilled a hole on the bottle cap to allow me to insert the rod partially into the bottle.
I then designed a twist-lock mechanism that allows me to secure the dowel rod at any desired height. The mechanism consists of a threaded cap and a hexagonal nut with small teeth that bite down into the dowel rod to secure it in place.
The ability to adjust the rod in this manner gave me a few inches of up and down adjustment, which makes adjusting the float easier.
Step 4: Option 1: TTL Control Circuit
I chose to use a 7400-series TTL integrated circuit as the heart for my controller. While less used today, 7400-series integrated circuits were popular in the 80s and each chip contain one or more digital logic building blocks that can be wired together to form a digital circuit.
For my circuit, I used a 7400 chip, which contains four NAND gates. One of the NAND gate is used to combine the LO_WATER and HI_WATER signals, two of these NAND gates are wired together to make a NAND-gate latch, while the remaining is wired as an inverting-buffer.
My circuit also contains an indicator light (LED1), two current limiting resistors (R1 for the photointerrupter LEDs , R4 for the indicator LED) and two pull-up resistors (R2 and R3) for the HI_WATER and LO_WATER signals.
One the right side of the schematics is the solid state relay (T1) and the sump pump motor (M1). The solid state relay requires about 10mA of current on the control line. The TTL chip can only source about 400 µA, but it can sink up to 16mA. So I have connected the positive control terminal of the relay to 5V and I am using an inverted buffer to pull the negative terminal down to 0V, allowing the chip to sink rather than source current.
I assembled my circuit on a protoboard using hookup wire. As you can see, soldering up the circuit required quite a bit of work!
Attachments
Step 5: Option 2: Using an Arduino As the Control Circuit
Unlike TTL integrated circuits, which must be wired up to make a specific logic function, an Arduino is a general purpose computer whose behavior can be programmed by software. While the TTL 7400 integrated circuit has a grand total of four logic gates, the Atmel microcontroller at the heart of the Arduino contains millions of logic gates!
Using an Arduino to implement this sump pump controller is like asking a chess grandmaster to play Tic-Tac-Toe, but the Arduino makes the circuit far simpler, with fewer components and interconnections. Although I did not use an Arduino for my setup, I verified that it worked as expected on a breadboard (pictured).
Aside from the Arudino, the only parts needed are a 100Ω current limiting resistor (R1), the two photointerrupters (O1 and O2) and the solid state relay (T1). The pull up resistors on the LO_WATER and HI_WATER lines are provided internally by the Arduino's digital input and a single digital output provides enough current to control the solid state relay.
The following Arduino sketch will turn the sump pump on when the water in the well is above the low and high water level and turn it off when the water in the well is below the low water level:
#define LO_WATER 10 #define HI_WATER 11 #define PUMP_SSR 12 void setup() { pinMode(LO_WATER, INPUT_PULLUP); pinMode(HI_WATER, INPUT_PULLUP); pinMode(PUMP_SSR, OUTPUT); pinMode(LED_BUILTIN, OUTPUT); } void loop() { if(!digitalRead(LO_WATER)) { // Turn off the sump pump when water is below the low water level digitalWrite(LED_BUILTIN, LOW); digitalWrite(PUMP_SSR, LOW); } if(digitalRead(LO_WATER) && digitalRead(HI_WATER)) { // Turn on the sump pump when water is above the low and high water level digitalWrite(LED_BUILTIN, HIGH); digitalWrite(PUMP_SSR, HIGH); } delay(1000); // wait for a second }
Step 6: Obtaining and Wiring Up the Solid State Relay
This step requires you to work with AC line voltage. If you are not qualified for this work, I recommend leaving this part up to a licensed electrician.
For controlling the sump pump, I bought a Schneider Electric SSM1A112BD Solid State Relay from Grainger. This relay takes in a control signal of 4V to 32V DC, which allows it to be driven by the 5V signals from either my TTL-based controller or an Arduino.
This solid state relay can switch up to 12 amps at 280V AC, which is sufficient for the sump pump my dad was using. I wired the relay in series to a power cord and an outlet box so my dad could plug in the sump pump into the relay.
The controller circuit provides a 5V control voltage for the relay so it can switch the pump on and off.
The relay can be mounted to the wall using a DIN rail, or using a small 3D printed bracket.
Attachments
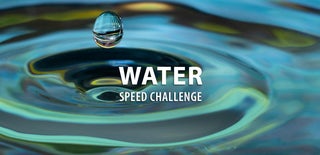
First Prize in the
Water Speed Challenge