Introduction: Street Light Timer Controller
REQUIREMENT
1.RELAY
2.ARDUINO PRO mini,or ARDUINO UNO
3.LCD DISPLAY 16x2
4.RTC REAL TIME CLOCK DS1307
5.12v ADAPTER
6. LM7805 5v regulator
7. FTDI232 FOR upload arduino file
Step 1:
MAKE 12v to 5v DC STEP DOWN
Step 2:
MAKE RELAY CIRCUIT AND DISPLAY CIRCUIT FOR ARDUINO
Step 3:
//CODE SET TIME SWITCH ACCORDING REQUIREMENT
#include "Wire.h"
#includeLiquidCrystal lcd(7,6, 5, 4, 3, 2); //lcd (rs, enable, d4, d5, d6, d7)
const char* AMPM; int bulb=0;
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
char* days[] = { "","Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat" };
int H; String s, m, d, mth, h;
#define DS1307_I2C_ADDRESS 0x68
byte on[8] = { 0b01110, 0b11111, 0b11111, 0b11111, 0b11111, 0b11111, 0b11111, 0b01010 };
byte off[8] = { 0b01110, 0b10001, 0b10001, 0b10001, 0b10001, 0b10001, 0b11111, 0b01010 };
byte spark[8] = { 0b00000, 0b00000, 0b00000, 0b00000, 0b00000, 0b00000, 0b10101, 0b01010 };
void setup()
{
Wire.begin();
lcd.createChar(1, on); // ON bulb cracter
lcd.createChar(0, off); // OFF BULB CRATCTER
lcd.createChar(2, spark); // SPARK BULB
lcd.begin(16, 2);
lcd.print(" DIAMOND AHIR");
lcd.setCursor(0,1);
lcd.print(" X-PERT GROUP");
delay(3000);
Serial.begin(9600);
}
void loop()
{
getDateDs1307(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month, &year); // GET values
ampm();
Convert_Hours();
lcd.clear(); // clear display
displaytime(); // Display Time
match_bulb_time(); // MATCHING WITH SET BULB TIMEING
delay(1000); // Wait 1 second
}
byte bcdToDec(byte val){ return ( (val/16*10) + (val%16) );}
void getDateDs1307(byte *second,byte *minute,byte *hour,byte *dayOfWeek,byte *dayOfMonth,byte *month,byte *year)
{
// Read values from RTC DS1307
Wire.beginTransmission(DS1307_I2C_ADDRESS);
Wire.write(0);
Wire.endTransmission();
Wire.requestFrom(DS1307_I2C_ADDRESS, 7);
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read()); }
void ampm() { (hour > 11)?(AMPM="PM"):(AMPM="AM"); }
void Convert_Hours() { if(hour == 12||hour == 0) H=12; else if(hour > 12)H=hour%12; else H=hour; }
void displaytime()
{
if(minute == 0) // ON EVERY HOURS IT DISPLAY FOR ONE MINUTES
{
lcd.begin(16, 2);
lcd.print(" DIAMOND AHIR");
lcd.setCursor(0,1); (H < 10) ? ( h = "0" + String(H)) : ( h = String(H));
// APPLY ZERO + with 1to9 Hours
(minute < 10) ? ( m = "0" + String(minute) ) : ( m = String(minute) ); // APPLY ZERO + with 1to9 Minutes
(second < 10) ? ( s = "0" + String(second) ) : ( s = String(second) ); // APPLY ZERO + with 1to9 Second
lcd.print(" " + String(h) + ":"+ m +":"+ s + " " + AMPM );
}
else
{
lcd.begin(16, 2);
(H < 10) ? ( h = "0" + String(H)) : ( h = String(H)); // APPLY ZERO + with 1to9 Hours
(minute < 10) ? ( m = "0" + String(minute)) : ( m = String(minute) ); // APPLY ZERO + with 1to9 Minutes
(second < 10) ? ( s = "0" + String(second)) : ( s = String(second) ); // APPLY ZERO + with 1to9 Seconds
(dayOfMonth < 10) ? ( d = "0" + String(dayOfMonth)) : ( d = String(dayOfMonth)); // APPLY ZERO + with 1to9 Day
(month < 10) ? ( mth = "0" + String(month)) : ( mth = String(month)); // APPLY ZERO + with 1to9 Month
lcd.setCursor(0,0);
lcd.print( h + ":" + m + ":" + s + " " + AMPM); // HOURS : MINUTES : SECONDS AM/PM
lcd.setCursor(0,1);
lcd.print( d + ":" + mth + ":" + year + " " + days[dayOfWeek]); //DAY:MONTH:YEAR WEEK
}
}//END FUNCTION
void match_bulb_time()
{
switch (hour) // Apply bulb to On with Hours
{ // 6-AM to 6-PM To On Bulb
case 0: bulb=1; break; //12
case 1: bulb=1; break; //1
case 2: bulb=1; break; //2
case 3: bulb=1; break; //3
case 4: bulb=1; break; //4
case 5: bulb=1; break; //5
case 6: bulb=1; break; //6
case 7: bulb=0; break; //7
case 8: bulb=0; break; //8
case 9: bulb=0; break; //9
case 10: bulb=0; break; //10
case 11: bulb=0; break; //11
case 12: bulb=0; break; //12
case 13: bulb=0; break; //1
case 14: bulb=0; break; //2
case 15: bulb=0; break; //3
case 16: bulb=0; break; //4
case 17: bulb=0; break; //5
case 18: bulb=1; break; //6
case 19: bulb=1; break; //7
case 20: bulb=1; break; //8
case 21: bulb=1; break; //9
case 22: bulb=1; break; //10
case 23: bulb=1; break; //11
default: break;
}
if(bulb==1) //Relay switch
{ digitalWrite(8,LOW); // OR connect To ground
digitalWrite(9,HIGH); //ON RELAY
// Display BULB Icon On LCD
lcd.setCursor(14,0);
lcd.write((byte) 2);
lcd.write((byte) 2);
lcd.setCursor(14,1);
lcd.write((byte) 1);
lcd.write((byte) 1);
}
if(bulb==0)
{
digitalWrite(8,LOW); // Or Connect to ground
digitalWrite(9,LOW); //OFF RELAY
// Display BULB OFF Icon
lcd.setCursor(14,1);
lcd.write((byte) 0);
lcd.write((byte) 0);
}
}
Step 4:
NOW BLUB ON OFF ACCODING TO URS SET TIME
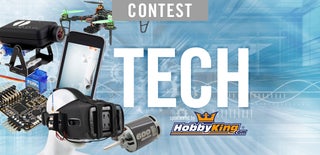
Participated in the
Tech Contest
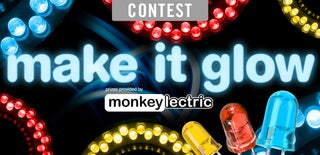
Participated in the
Make It Glow! Contest