Introduction: 3D Printer Filament Color Sensing Mood Light
This is my first Instructable so if there are any questions, or suggestions please leave a comment below.
In this Instructable I will show you how to make a filament color detection mood light for your 3D printer. The color sensor will detect the color of the filament you are using and light up your 3D printer with that color. You can use it to pimp out your 3D printer while printing your parts for the 3D printing contest ;)
This Instructable is pretty easy and can be put together for less than $10 (€ 9). I have tried to explain all steps and included some background information.
Parts you will need:
1x Arduino (preferably a Nano or Mini)
1x TCS3200 color sensor:
http://www.ebay.com/itm/TCS230-TCS3200-Color-Recog... $3 (€ 2.6)
1x WS2812 strip of RGB LEDs:
https://www.sparkfun.com/products/12661 $6 (€ 5.3)
http://www.ebay.com/itm/351493895711 $1.5 (€ 1.3)
Step 1: How the Color Sensor Works
Before we start lets take a moment to see how the color sensor works and is able to detect colors. This step is optional so you can skip ahead if you want.
Now is also a good time to download the datasheet of the TCS3200 which can be found here: http://www.mouser.com/catalog/specsheets/TCS3200-...
The TCS3200 color sensor has a bunch of small photodiodes (64 to be exact) inside its package. Photodiodes generate current when exposed to light. The more light there is the more current is generated.
In the sensor these photodiodes have filters on top of them: 16 of them have red filters, 16 have green filters, 16 have blue filters and 16 have no filters. A colored filter will only let light with that color through and block all other colors. So the diodes with the red filters will only measure the red light, the diodes with blue filters will measure the blue light, and the diodes with green filters will measure the green light. More information about color filters can be found here: http://www.education.com/science-fair/article/col...
The sensor measures the current passing through the photodiodes and converts it into pulses. The more current the quicker the pulses (the frequency) are. By counting the pulses in a fixed time we can determine how much red, green and blue is present. A picture of this is shown from the datasheet:
Interesting side note:
If you go to page 5 in the datasheet of the sensor you can find this super complicated looking graph, this shows us how the sensor sees the red, green and blue. The sensor works very similar to our eyes. In our eyes we have three types of cone cells; Short, Medium and Long cone cells. These are the same as the three different types of photodiodes in the sensor. The way our eyes see the colors are given below. As you can see our eyes are much better at distinguishing the different primary colors, each with about the same sensitivity and no ‘bumps’ at different wavelengths. ( https://en.wikipedia.org/wiki/Cone_cell )
Step 2: The Electronics
Let’s start by hooking up the sensor, Arduino and RGB LEDs. This is fairly simple, see the Fritzing diagrams for reference. The RGB strip may require soldering some connecters to it.
Below is an explanation of the pins on the color sensor and RGB strip is given below:
Color Sensor:
GND: Connect this pin to the ground on your Arduino.
VCC: Connect this pin to the 5V on the Arduino
S0,S1: These two pins can be used to select the scaling of the output frequency. The higher the output frequency the more detail we will be able to detect in the colors. We won’t change this in software so we connect both pins to 5V to get 100% frequency output.
S2,S3: These two pins are used to select the color we want to measure. We will measure the colors one at a time and use these pins to tell the sensor which color we want to measure. By default this is pin 3 for S2 and pin 4 for S3 in the code.
OE: This pin is used to enable and disable the sensor output. We will not be needing this so to keep the sensor enabled we connect this to GND.
OUT: This pin will generate the pulses, the quicker the pulses the more light is present. This is the input to our application. By default this is pin 2 in the code.
RGB Strip:
GND: Connect this pin to the ground on your Arduino.
4-7VDC: Connect this pin to the 5V on the Arduino
DIN: Data input for the LEDs. By default this is pin 5 in the code.
Now connect power to your Arduino. If all is well the LEDs on the color sensor should light up.
Step 3: The Code
Instead of just dumping the code I will first explain a bit how the code works. If you are not interested you can just download the code in the end of this step.
The code requires the Adafruit_Neopixel library. To install it go to Sketch->Include Library->Manage Libraries and type Neopixel in the search box, click on the Adafruit NeoPixel and click on Install.
With the sensor now hooked up we can try to read some values from it. This is actually quite easy. All we need to do is select the color we are interested in and count how many times the output pin changes from high to low in a given time. We then repeat this for each color and we have our measurements. Download and unzip the code to follow along with the explanation below.
Reading from the sensor:
The measure color function does all the work. The outer loop is a loop that loops 3 times, once for red, green and blue. Inside the loop the current time is stored and a while loop is entered for 25ms. Inside the while loop we keep checking the state of the OUT pin, if it changed from HIGH to LOW or LOW to HIGH we increment the count and save the state. We keep doing this until the measurement time is up. After this we save the count as the raw value for that color, reset it and select the next color. By the time this function is finished the ColorData struct has the raw measured values for red, green and blue. It’s that simple.
Converting to colors:
In order to get the actual colors we will need to calibrate the sensor, we will do this in the next step. But first lets explain what we are calibrating. We assume the sensor is linear, so if the output count increases the amount of light increases at the same rate. https://en.wikipedia.org/wiki/Linear_function Therefore the color is given by a function in the form of color = a*(count)+b . This is explained for the sensor at https://arduinoplusplus.wordpress.com/2015/07/15/tcs230tcs3200-sensor-calibration/ and we will use the same method to calibrate the sensor.
The last thing we will do is scale up the color we sensed. We do this to prevent dim LEDs. So we take the highest color and set it to the maximum of 255 and scale the other two colors accordingly.
RGB LED:
The LEDs are set in the main loop. After reading the color we will loop through all the LEDs and set the color of the LEDs to the color we read.
Attachments
Step 4: Calibration
In order to recognize the colors we need to calibrate the sensor. You might need to repeat this calibration based on the ambient light. I have made a simple page with different colors on it you can print it out to calibrate and test the sensor, or you can use white and black paper.
Upload the code to the Arduino and open the serial monitor with a baud rate of 115200. You should see the monitor spitting out lines like: Red: 746 0 Green: 493 0 Blue: 572 0. The first number after the color is the count from the frequency.
Calibration
If you remember from science class white consists of all colors and black of no colors. We can use this to calibrate our sensor by measuring the maximum (white) and minimum (black) amount of color..
Place the white paper about 2.5 cm (1 inch) in front of the sensor and you should see all the measured values go up. Record the maximum count number for each color.
Place the black part in front of the sensor and all measured values decrease. Record the minimum count number for each color.
Find the follow lines in the beginning of the code and replace them with your measured values:
/* Calibration data */
#define RED_MIN 748
#define GREEN_MIN 648
#define BLUE_MIN 791
#define RED_MAX 3117
#define GREEN_MAX 2948
#define BLUE_MAX 3102
Re upload the code and put some colored things on top of the sensor. The LEDs should change to the color of the object. I also made a Processing sketch that you can download which changes the screen color based on the sensed color, you might need to change the COM port in the sketch though.
Step 5: 3D Print Stand/cover and Assembly
To hold and align the sensor towards the filament roll, I created a 3D part that can be printed. Simply print it with a layer height of 0.25mm and preferably with a non glossy black filament and orientated as shown in the image.
The stand can either stand or lay down. Once you have assembled the sensor on the stand it has to be aimed at the filament, by about the same distance that it was calibrated with. You will also need to perform the calibration again.
To assemble solder the sensor wires to the connectors on the Arduino Nano and connect the RGB strip with longer wires to make it possible to mount it on your printer
Step 6: Enjoy
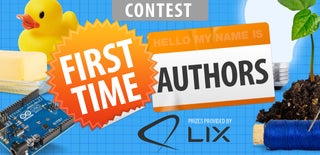
Participated in the
First Time Author Contest 2016
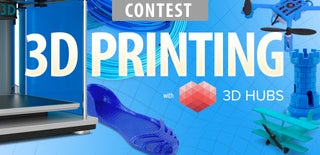
Participated in the
3D Printing Contest 2016