Introduction: A Guide to Controlling Things With Varying Input (via Arduino)
the heart of this project is a) hooking up a sensor to arduino analog input and b) programming with the if...else statement.
this particular project demonstrates a computer cooling system to maintain certain temperature within a computer tower.
i used the following items and ill explain each later. some aren't needed or can be substituted.
Arduino uno (the programming is so simple just about any arduino or other microcontroller will work)
a 9 volt clip if you don't want to power the arduino via computer
an Atx power supply
jumpers and alligator clips (alligator clips just for testing)
MintDuino kit (suggested for its built in stuff, totally not needed usually)
a thermosister (mine came with the arduino ultimate kit)
usb cable for arduino
I used the seeed studio relay sheild, other things work too
A fan or peltier device
a box to cool (my desktop computer)
the box motherboard/cpu (as something that gets hot)
LCD display (for pizazz)
a 16 pin header (to solder to LCD)
an ide cable or a floppy cable, etc. to extend lcd
wire for extending things
makersheild/protosheild
the Arduino IDE software and drivers
tools
multi-meter for testing
a wire wrap tool or soldering iron
pliers
small flat-head screwdriver for relay shield
any other tools you feel are handy for this stuff
pictures of materials and how to use them will come in following steps
Step 1: Power Management
somehow everything needs to be powered. recently i discovered that you can use an atx power supply (found in most desktop PCs) as a 5 volt or 12 volt power supply. When an atx power supply is plugged into a motherboard of a computer, it provides power to everything when you press the on button of the computer. this happens because the on button connects the green whire to ground, in the atx plug. you'll need to use a jumper wire or alligator clips to connect green to one of the black. now the power supply thinks it is on. you may add a switch for on/off functionality so you dont need to unplug it every time, or use the switch on the supply for this. mine didn't have one.
Step 2: Testing With Meter
Now that the atx thinks it is 'on', test it. the standard-looking plugs pictured have one yellow wire, two black, and one red. the black ones are both ground. red, i found, is about 5 volts (5.16, but when you put a load on it it is about 5) and the yellow wire is just shy of 12 volts. i use a radioshack pocket multi-meter. it is auto range, which means you dont need to turn any knobs to get a proper reading, but it takes longer to get your answer. it was perfect for this project. put the black lead on a ground and test the "hot" lines with your meter probes. check pictures for reference.
Step 3: Thermosister
I needed a way to place the thermosister where i wanted, to i wire wrapped some longer wire onto it.
the wire wrapper is a strong, simple alternative to soldering wires together. in small applications like component legs, it is a must.
as an example, in the pictures, i wire wrapped an led and a resister. you can see the 3.3v led powered by 5volts on arduino pin 1.
this isnt needed by the project, but illistrates how i attach the legs on the thermosister.
how the thermosister works is, it is a resister that changes how much electricity goes through it based on its temperature.
when working with microcontrollers, you need to wire potentiometers, resisters, buttons and the like sort of oddly.
you need to make one leg of the thermosister plug into +5v on the arduino. the other leg needs to go to a resister (in this case i used a 10k potentiometer) then to ground.) this leg also goes strait into an anolog input on the arduino refer to my sharpie-marker schematic for how this looks. it works becuase the 5 volts want to seek ground, and they go through the resister to get there. when you "sample" what is coming in on the analog input, power goes there instead because it is the path of least resistance. any variable analog sensor will use this basic setup. i used the potentiometer so i could adjust the range and set it for various future changes, but you don't need it. i leave the pot cranked to one side, simulating a 10k resistor. put all the components on a breadboard arranged in the circuit on the schematic. A0 is the analog 0 input on the arduino. GND is ground, there are three of these pins on the arduino uno. the masking tape you see on the thermometer is to insulate the pins, so that it can lay against metal and not short the wires out.
Step 4: Analog Sensor Test
now for the software.
you need a way to test the circuit, and make sure it delivers numbers that change with the temperature.
try not to touch the tip of the sensor for a while, so it can stabilize at your rooms temperature.
download the software, set up the drivers, etc. Refer to http://arduino.cc/en/Guide/HomePage for information regarding set up.
if you have the ide working and can program the arduino, time to begin testing.
open the included sketch at [ File>Examples>01.Basic>AnalogReadSerial ]. refer to the picture.
choose the right com port and board from the tools menu.
upload the sketch to the arduino, i pictured this.
once it gives you the ok that the sketch has been uploaded successfully, press either Ctrl+Shift+M or Tools>Serial Monitor.
the window that appears is a serial communication with the arduino. in this case, our program reads out the numbers it is getting from the sensor. if you used a pot in place of the 10k resistor, turn it until the numbers are the largest. the resister will already be at 10k. watch the numbers, while the sensor is at room temperature. then pinch the sensor with your hands or breath on it. if you are using a hot laptop, put the sensor near the heat exhaust. experiment with the sensor and decide on a constant for the temperature.
now is a good time for snacks
Step 5: Connecting Your LCD
in the arduino ultimate kit, i got a small 16x2 character LCD display.
refer to my picture for a wiring diagram on what goes where. the pot in the diagram is another 10k.
it controls the contrast on the screen, more of this pot later.
first the LCD comes as a through-hole type card.
I used the included pin headers, broke it to 16 pins long, and soldered it to the holes.
the one i used is in the middle of the picture, with one end of the pins long enough to go into a breadboard
(or later the IDE cable). and the other end of the pins are long enough to solder onto the circuit board like i did.
I have also seen some push the pins through the holes into a breadboard, which may work for you.
once you have the LCD wired up to the arduino, load the sketch "hello world" under the liquid crystal examples.
when running, watch the screen and turn the contrast pot. somewhere while turning the pot the text should be most visible.
leave it there for now.
Step 6: IDE Cable LCD Extension
use your multimeter in the diode/short mode, it can tell when the two leads are contacting of not. i used alligator clips on the leads, and on the other ends i added two jumper wires, this essentially made my leads tiny on the end. now i check the IDE cable to see what pins one end are connected to what pins on the other. this ensures that my lcd, which we will connect next, is plugged in properly. now push the pins of the lcd into one side of the ide cable. on any row of 16 pins that all were connected to 16 on the other side when you tested. on some of my cables one or two pins didn't short from one cable side to the other so avoid these. plug your jumpers on the arduino side in to the cable as well, making sure they connect in the right order, as they do one the diagram. run the hello world sketch again.
Step 7: Fan Switching
now for the fan.
the fan needs to be switched on and off, but the arduino only outputs 5 volts, while my fan is a 12 volt.
there are multiple solutions, the main one being a relay (mechanical or solid state). i used the SEEED studio relay shield for seeeduino and arduino. it is a card that plugs in on top of the arduino, uses an external 9 volts to power four relays, and you can loop power through these relays to control high power devices with low power. unfortunately the card covers up all arduino pins, without enough extra contacts to run an LCD, receive data from our thermometer, and jump the relays. so i soldered two female headers on the board so that i could trip the relays without it sitting on the arduino. i just need to connect ground and one digital pin that will be my fan "on" or "off". The 12 volts coming from my ATX power supply will go through the screw terminals for one of the relays, on common and normally open. this means that without touching the relay, the circuit is open, so the fan is not on. then if you power one of the pins on the header I soldered in, it closes the circuit between common and N.C. making the fan turn on. you don't need the relay shield for this, look for solid state relays, transistors, Ive seen some people glue a servo to a rocker switch.
i unplugged my fan from where it started on the motherboard, and used the meter in volt mode to determine where ground and +12v were. then i lined up these connections to the plug on the fan, so i know how to hook it up. ground to ground on the ATX, and the yellow wire (+12v) goes through the relay to yellow on the ATX.
Step 8: Putting It All Together
If you removed one part or your project to add another, time to put it all back together. plug in the lcd on it's pins, then pin 7 and ground for the relay, the pot for the lcd, the sensor and pot/resistor on analog 0, and it helps to use a makershield. one came with my arduino ultimate kit. it has breakouts for ground and +5v, a built in 10k pot, and most important, a small breadboard usable for putting components like the pot or resistor on the sensor. also it carries all the pins to the top so you still can plug everything in, unlike on the relay board.
here i set all my components in the box, on my paper from the earlier sharpie schematic as an insulator (recycling, i get points right?) so i dont short out the pins of the arduino , since it is sitting on the metal case of a zip drive, stacked on three floppy drives. these get the components high up in the box so they can plug in to everything. as you see, i pushed the thermosister between two plates of the heat sink near the cpu of the computer.
plug everything in, it is time for programming.
Step 9:
as a test of the relay, locate the "blink" sketch under File>Examples>01.Basic>Blink
find the line of code where it says "int led = 13" and change 13 to 7, like in the
picture. now, instead of putting an on signal to pin 13 (and the led connected to it) the arduino will put the signal out to pin 7. pin 13 has a resistor built in, and all the others do not, and pin 7 is not being used by the LCD so it is free for the relay. in my case I connect relay 4 of the shield to pin 7 and ground. I plug the relay board into a 9 volt battery with a clip. you should see the fan turn on then off then on then off. play with the code, change the delay near the bottom of the page. instead of 1000 milliseconds make it 5000 or 500. notice the first delay is 'on' time, the second is 'off' time. if it works, you are ready for programming.
Step 10:
the program will work as follows:
if the temperature of the sensor is hotter than a predetermined value,
turn on the fan to cool down the box.
if it is colder, turn off the fan.
all this time it will
a) update the lcd screen with current temperature and
b) send serial data to the computer, mirroring that of the lcd display.
i began the software bny using "analog read serial" as a base to add my own code to it.
in the lcd hello world example, you see "#include <LiquidCrystal.h>"
in the beginning. this opens the "liquid crystal" libraries. this means later you can reference back with lcd commands like "setCursor" or "print".
add this line of code somewhere near the top of "analog read serial".
there is a part of the code called the void setup. this is where you can put commands which run once, like setup information. there is one command we can add here. "lcd.begin(16, 2);"
this opens a new lcd screen with 16x2 characters. 2 tall by 16 wide.
look at my code for everything you need to add.
the secret is the if/else statement.
******************************
this says IF the temperature is (more than 4 degrees or whatever)
then do this.
other wise (else),
do this.
*******************************
this part of the code lets you specify various actions which occur based on varying input, in this case the temperature, but it could be speed of a car or brightness of a room or the time or some math computations, likewise it can control anything from the lights to switching the radio for you or turning on your coffee pot at the right time. the possibilities really are endless.
the image is not all the code, so dont bother transcribing it. find the code on the next page.
Step 11: All the Code, Copy It All. Read the //notes for Assistance and Explanation.
/ include the liquid crystal library
#include <LiquidCrystal.h>
// the setup routine runs once when you press reset:
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
// Pin 7 has our relay that trips the fan
// give it a name:
int fan = 7;
void setup() {
// initialize serial communication at 9600 bits per second:
Serial.begin(9600);
// start 16x2 character lcd display:
lcd.begin(16, 2);
// print a message to the lcd
lcd.print("hello, world!");
// initialize the fan (pin 7) as an output.
pinMode(fan, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
// read the input on analog pin 0:
int sensorValue = analogRead(A0);
lcd.setCursor(0, 1);
// print out the value you read to lcd then serial:
lcd.print(sensorValue);
Serial.println(sensorValue);
delay(1); // delay in between reads for stability
// if else statement. if temperature is hotter than 150, turn on fan. else keep fan off:
if (sensorValue > 160)
{
digitalWrite(fan, HIGH); // turn on or keep on fan relay
}
else
{
digitalWrite(fan, LOW); // turn off or keep off fan relay
}
} //end of loop. do it again.
Step 12: Thats a Wrap
good luck programming and automating things, ask questions in the comments.
as a newcomer to 3d printing, i appreciate a vote for the "UP" contest, it really is a beautiful printer and id love to call one mine.
NOTE: i got a little carried away and built a whole functioning computer with temperature regulated by the arduino, it can switch harddrives and screen modes and the arduino can control the computer itself. all in an ikea box of all things.
if you want pictures, ill get some.
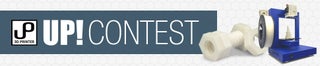
Participated in the
UP! Contest