Introduction: Android Controlled LED Strip (IOIO Powered)
or watch in HD
The Android phone is controlling an LED strip hidden in the vase using a IOIO board with Bluetooth. In this example, the Android phone is running an app which obtains color values from the onboard camera and then in turn matches those colors to the LED strip. We (myself and Ytai) sort of ran out of time on this project but with the sample code, it can easily be expanded to do other things. Here's a few ideas we didn't get to:
Using the phone accelerometer to change the color patterns, a shake event for example
Displaying a pattern when receiving a text
Displaying a pattern when receiving a phone call
Displaying a pattern when a friend if nearby via social networking
Displaying a pattern on specific Twitter keywords
IOIO is a physical computing platform for the Android platform, kind of like Arduino but dedicated to Android and features a small form factor with Bluetooth capability leveraging off the shelf & inexpensive Bluetooth dongles. IOIO was created by Ytai Ben-Tsvi who was my partner on this project, actually Ytai did all the work. I've done a few Arduino projects in the past that also required a PC for the graphical & rich internet application capabilities, it was nice see how one can get rid of the PC and yet still retain these rich capabilities leveraging the Android platform. This opens up many new application possibilities for hobbyists.
The LED strip is digitally addressable meaning each LED (32 in the strip) can be controlled individually. That combined with the Bluetooth IOIO and Android opens up endless interactive lighting schemes.
Materials
- IOIO Board - $50
- RGB LED Strip Addressable from Sparkfun - $45
- Bluetooth Dongle - $5
- Android Device *
- Hookup Wire
- Form factor of your choice for the LED strip(s), vase used in this example
- Android Holidays IOIO App (Android Market link) or here for users without Android Market - free
* Android 2.3.3 and above required for Bluetooth
Android 2.1 to 2.3.2 will support Bluetooth but you'll need to enter the Bluetooth pairing pin on every connection
Android 1.5 and above can use a USB cable connection as opposed to Bluetooth. For USB connections, the "USB debugging" setting (also known as ADB) must be turned on in your Android phone, you'll find this setting under "Settings", "Applications", and "Development".
Step 1: Wiring
The wiring is pretty easy. First and this is very important, look for the connector on the LED strip with an arrow (see the pictures), it should be the strip end with one connector as opposed to the strip end with two connectors. That is the end of the LED strip where you'll connect the 4 wires per the schematic below. The other end of the LED strip (with two connectors) also has a similar connector but you'd use that to daisy chain another LED strip. In this particular case, we're only using one LED strip so we don't need this connector. If this is the case for you, then you can save yourself some time by cutting off that connector (female) and then plugging it into the single connector end (male) and then wire to the IOIO board.
Outside of the DC power source which go to the VIN and GND pins on the IOIO (DC power between 5V and 15V), you'll only need to connect 4 wires on the LED strip to the IOIO.
LED Strip Blue Wire --> IOIO 5V
LED Strip Black Wire --> IOIO GND
LED Strip Green Wire --> IOIO Pin 3
LED Strip Red Wire --> IOIO Pin 4
Outside of the DC power source which go to the VIN and GND pins on the IOIO (DC power between 5V and 15V), you'll only need to connect 4 wires on the LED strip to the IOIO.
LED Strip Blue Wire --> IOIO 5V
LED Strip Black Wire --> IOIO GND
LED Strip Green Wire --> IOIO Pin 3
LED Strip Red Wire --> IOIO Pin 4
Step 2: Quick Test Before Installation
Do a quick test to ensure the LED strip can be controlled by the Android device. Connect the bluetooth dongle to the IOIO, power on, and then pair to your Android device. It will show up as "IOIO (address number)" in the bluetooth discovery screen. Select it and then enter "4545" for the pairing code.
Then install the Holidays IOIO app for this project and see if the LED strip lights up. Make sure the camera on your phone is not facing down so it's picking up some colors.
Then install the Holidays IOIO app for this project and see if the LED strip lights up. Make sure the camera on your phone is not facing down so it's picking up some colors.
Step 3: Installation
With the electronics working, now it's time to install the LED strip in your form factor of choice and also hide the IOIO board somewhere in there. If it's a permanent installation, then you'll want to solder the wires from the LED strip directly to the IOIO board or if it's temporary, then solder some header pins on your IOIO board and connect to the LED strip using jumper cables.
The LED strip has an adhesive backing so you can just stick it on.
The LED strip has an adhesive backing so you can just stick it on.
Step 4: The Fun Part
Now the fun part. Here's where you'll write the code to tell the Android phone how to control the LED strip. To make things easier, refer to the example code of this project and then just tweak for your needs, here's a direct link to the Android activity code (main body of code). The sky is the limit here in terms of interactive scenarios, anything you can get to with an Android phone becomes possible.
For the folks that know Arduino, coding Android is a little more difficult but not too bad, you'll get the hang of it pretty quick. Here's the traditional "hello world" application for IOIO and Android. Read through the code along with the comments in Bold Italics and you'll get the gist.
package ioio.examples.hello;
import ioio.examples.hello.R;
import ioio.lib.api.DigitalOutput;
import ioio.lib.api.exception.ConnectionLostException;
import ioio.lib.util.AbstractIOIOActivity;
import android.os.Bundle;
import android.widget.ToggleButton;
/**
* This is the main activity of the HelloIOIO example application.
*
* It displays a toggle button on the screen, which enables control of the
* on-board LED. This example shows a very simple usage of the IOIO, by using
* the {@link AbstractIOIOActivity} class. For a more advanced use case, see the
* HelloIOIOPower example.
*/
publicclass MainActivity extends AbstractIOIOActivity {
private ToggleButton button_;
/**
* Called when the activity is first created. Here we normally initialize
* our GUI.
*/
@Override
publicvoid onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);//all Android app have this line, just make sure it's there and don't worry about it
setContentView(R.layout.main); //in Android, the screen layout comes from an XML file you specificy, main.xml here
button_ = (ToggleButton) findViewById(R.id.button);
}
/**
* This is the thread on which all the IOIO activity happens. It will be run
* every time the application is resumed and aborted when it is paused. The
* method setup() will be called right after a connection with the IOIO has
* been established (which might happen several times!). Then, loop() will
* be called repetitively until the IOIO gets disconnected.
*/
class IOIOThread extends AbstractIOIOActivity.IOIOThread {
/** The on-board LED. */
private DigitalOutput led_;
/**
* Called every time a connection with IOIO has been established.
* Typically used to open pins.
*
* @throws ConnectionLostException
* When IOIO connection is lost.
*
* @see ioio.lib.util.AbstractIOIOActivity.IOIOThread#setup()
*/
@Override
protectedvoid setup() throws ConnectionLostException {
led_ = ioio_.openDigitalOutput(0, true);
}
/**
* Called repetitively while the IOIO is connected.
*
* @throws ConnectionLostException
* When IOIO connection is lost.
*
* @see ioio.lib.util.AbstractIOIOActivity.IOIOThread#loop()
*/
@Override
protectedvoid loop() throws ConnectionLostException {
led_.write(!button_.isChecked());
try {
sleep(100);
} catch (InterruptedException e) {
}
}
}
/**
* A method to create our IOIO thread.
*
* @see ioio.lib.util.AbstractIOIOActivity#createIOIOThread()
*/
@Override
protected AbstractIOIOActivity.IOIOThread createIOIOThread() {
returnnew IOIOThread();
}
}
For the folks that know Arduino, coding Android is a little more difficult but not too bad, you'll get the hang of it pretty quick. Here's the traditional "hello world" application for IOIO and Android. Read through the code along with the comments in Bold Italics and you'll get the gist.
package ioio.examples.hello;
import ioio.examples.hello.R;
import ioio.lib.api.DigitalOutput;
import ioio.lib.api.exception.ConnectionLostException;
import ioio.lib.util.AbstractIOIOActivity;
import android.os.Bundle;
import android.widget.ToggleButton;
/**
* This is the main activity of the HelloIOIO example application.
*
* It displays a toggle button on the screen, which enables control of the
* on-board LED. This example shows a very simple usage of the IOIO, by using
* the {@link AbstractIOIOActivity} class. For a more advanced use case, see the
* HelloIOIOPower example.
*/
publicclass MainActivity extends AbstractIOIOActivity {
private ToggleButton button_;
/**
* Called when the activity is first created. Here we normally initialize
* our GUI.
*/
@Override
publicvoid onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);//all Android app have this line, just make sure it's there and don't worry about it
setContentView(R.layout.main); //in Android, the screen layout comes from an XML file you specificy, main.xml here
button_ = (ToggleButton) findViewById(R.id.button);
}
/**
* This is the thread on which all the IOIO activity happens. It will be run
* every time the application is resumed and aborted when it is paused. The
* method setup() will be called right after a connection with the IOIO has
* been established (which might happen several times!). Then, loop() will
* be called repetitively until the IOIO gets disconnected.
*/
class IOIOThread extends AbstractIOIOActivity.IOIOThread {
/** The on-board LED. */
private DigitalOutput led_;
/**
* Called every time a connection with IOIO has been established.
* Typically used to open pins.
*
* @throws ConnectionLostException
* When IOIO connection is lost.
*
* @see ioio.lib.util.AbstractIOIOActivity.IOIOThread#setup()
*/
@Override
protectedvoid setup() throws ConnectionLostException {
led_ = ioio_.openDigitalOutput(0, true);
}
/**
* Called repetitively while the IOIO is connected.
*
* @throws ConnectionLostException
* When IOIO connection is lost.
*
* @see ioio.lib.util.AbstractIOIOActivity.IOIOThread#loop()
*/
@Override
protectedvoid loop() throws ConnectionLostException {
led_.write(!button_.isChecked());
try {
sleep(100);
} catch (InterruptedException e) {
}
}
}
/**
* A method to create our IOIO thread.
*
* @see ioio.lib.util.AbstractIOIOActivity#createIOIOThread()
*/
@Override
protected AbstractIOIOActivity.IOIOThread createIOIOThread() {
returnnew IOIOThread();
}
}
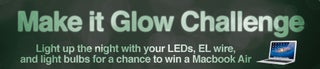
Participated in the
Make It Glow Challenge
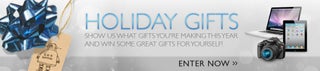
Participated in the
Holiday Gifts Challenge
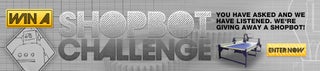
Participated in the
ShopBot Challenge