Introduction: Arduino Feeding Xively.com With Temperature From DS18B20
This instructable will explain how to:
* connect a DS18B20 temperature sensor to an arduino
* read from the sensor using the 1-wire interface
* send that data to xively.com directly from the arduino
* view the resulting graph on the internet
http://xively.com is an Internet of Things host that generates graphs based on data you provide. In this example I am going to use it to monitor the temperature in my room. It's not a hackerspace, my wife keeps it far too tidy for that.
Step 1: Parts List
* Arduino with ethernet shield
I have an arduino Uno clone and a wiznet 5100 shield.
* Power supply (it can be powered via the USB port)
I was fortunate that my router has USB ports that provide the 5v output meaning the arduino is powered whenever the router is and saves a plug, electricity, the environment and the future of the world.
* DS18B20 temperature sensor
* RJ45 network cable connected between the arduino and your router
Step 2: Schematic
* Arduino gnd to DS18B20 both outer legs
* Arduino 5v to 4.7k resistor leg 1
* 4.7k resistor leg 2 to DS18B20 middle leg
* Arduino digital pin 2 to DS18B20 middle leg
* RJ45 cable from Arduino to router
* Power to Arduino (via USB or barrel jack, I use USB from my router)
Step 3: Prerequisites
Three libraries are required for the arduino:
onewire library from http://www.pjrc.com/teensy/td_libs_OneWire.html (specifically http://www.pjrc.com/teensy/arduino_libraries/OneWire.zip)
httpclient library from https://github.com/amcewen/HttpClient (https://github.com/amcewen/HttpClient/archive/master.zip)
Xively library from https://github.com/xively/xively_arduino (https://github.com/xively/xively_arduino/archive/master.zip)
install these libraries in your arduino/library directory.
An account with http://xively.com (formerly pachube.com and cosm.com)
Sign up for a free developer account at https://xively.com/get_started/
Pick a username, password, set your address and timezone etc. You will receive a confirmation email, click the activation link to avtivate your account.
You can choose to take the test drive to learn about xively or skip it, this is completely up to you.
Doing the test drive? I'll wait
- \ | / - \ | /
Done? Let's continue
Step 4: Add a New Device to Xively
You should now be on the Development Devices page of xively. This is where the fun starts.
Click on +Add Device
Give the new device a name eg Arduino DS18B20 temperature logger
Fill in a description about the device, its location such as My Hackerspace, and yourself if you like. I tend to add a link to my web site here although there is a dedicated web address area later in the instructions.
Choose whether the data is public or private. Do you really want the world knowing how hot your hackerspace is? I choose public unless it is something like an alarm which I keep private.
Click on the Add Device button at the bottom of the page
Step 5: The Xively Interface for Your New Device, and All the Access Codes
You will now be on a page with a lot of information. Don't be overwhelmed – it is mainly set and forget stuff.
The page is broken up into 8 parts:
* product ID, secret, serial number, activation code
* status (Activated), feed ID, feed URL (this shows the data on a graph), API endpoint
* “Add channels to your device!” - the arduino will handle this in the sketch
* request log – this is for debugging and confirming everything is working from the arduino side
* location – the geographical location of the sensor (optional)
* api keys – we will need this later in the arduino sketch
* metadata – can be edited
* triggers – ping a web page when something happens (eg temperature drops below freezing)
Step 6: Arduino Sketch
<code>
#include <SPI.h>
#include <Ethernet.h>
#include <HttpClient.h>
#include <Xively.h>
#include <OneWire.h>
// MAC address for your Ethernet shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Your Xively key to let you upload data
char xivelyKey[] = ""; // enter the key, under API Keys
unsigned long feedId = 0; // enter your feed ID, under Activated
int frequency = 15000; // delay between updates (milliseconds)
// Analog pin which we're monitoring (0 and 1 are used by the Ethernet shield)
int sensorPin = 2;
// Define the strings for our datastream IDs
char sensorId[] = "temp";
XivelyDatastream datastreams[] = {
XivelyDatastream(sensorId, strlen(sensorId), DATASTREAM_FLOAT),
};
// Finally, wrap the datastreams into a feed
XivelyFeed feed(feedId, datastreams, 1 /* number of datastreams */);
EthernetClient client;
XivelyClient xivelyclient(client);
// initialize the one-wire interface
OneWire ds(sensorPin); // on pin 2 (a 4.7K resistor is necessary)
/*
5v - 4.7k resistor - 18B20 middle pin - D2
gnd - 18B20 both legs (joined together)
*/
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
Serial.println("Starting single datastream upload to Xively...");
Serial.println();
while (Ethernet.begin(mac) != 1)
{
Serial.println("Error getting IP address via DHCP, trying again...");
delay(5000);
}
Serial.print("IP address: ");
for (byte thisByte = 0; thisByte < 4; thisByte++)
{
// print the value of each byte of the IP address:
Serial.print(Ethernet.localIP()[thisByte], DEC);
Serial.print(".");
}
Serial.println();
}
void loop() {
byte present = 0;
byte type_s;
byte data[12];
byte addr[8];
float celsius;
int i;
if ( !ds.search(addr))
{
Serial.println("No more addresses.");
delay(250);
ds.reset_search();
return;
}
if (OneWire::crc8(addr, 7) != addr[7])
{
Serial.print("CRC is not valid!");
return;
}
// the first ROM byte indicates which chip
switch (addr[0])
{
case 0x10:
Serial.print(" Chip = DS18S20"); // or old DS1820
type_s = 1;
break;
case 0x28:
Serial.print(" Chip = DS18B20");
type_s = 0;
break;
case 0x22:
Serial.print(" Chip = DS1822");
type_s = 0;
break;
default:
Serial.println("Device is not a DS18x20 family device.");
return;
}
Serial.println();
ds.reset();
ds.select(addr);
ds.write(0x44, 1); // start conversion, with parasite power on at the end
delay(1000); // maybe 750ms is enough, maybe not
// we might do a ds.depower() here, but the reset will take care of it.
present = ds.reset();
ds.select(addr);
ds.write(0xBE); // Read Scratchpad
for ( i = 0; i < 9; i++) { // we need 9 bytes
data[i] = ds.read();
}
int16_t raw = (data[1] << 8) | data[0];
if (type_s)
{
raw = raw << 3; // 9 bit resolution default
if (data[7] == 0x10)
{
// "count remain" gives full 12 bit resolution
raw = (raw & 0xFFF0) + 12 - data[6];
}
}
else
{
byte cfg = (data[4] & 0x60);
// at lower res, the low bits are undefined, so let's zero them
if (cfg == 0x00)
raw = raw & ~7; // 9 bit resolution, 93.75 ms
else if (cfg == 0x20)
raw = raw & ~3; // 10 bit res, 187.5 ms
else if (cfg == 0x40)
raw = raw & ~1; // 11 bit res, 375 ms
//// default is 12 bit resolution, 750 ms conversion time
}
celsius = (float)raw / 16.0;
datastreams[0].setFloat(celsius);
Serial.print("Current temperature: ");
Serial.print(datastreams[0].getFloat());
Serial.println(" celsius");
Serial.println("Uploading it to Xively");
int ret = xivelyclient.put(feed, xivelyKey);
Serial.print("xivelyclient.put returned ");
Serial.println(ret);
Serial.println();
delay(frequency);
}
</code>
Step 7: Welcome to the Internet of Things!
Once you have uploaded the code to the Arduino you should start to see information appear in the Request Log section and datapoints appear on the graph.
Congratulations, you are now updating the world with the temperature in your hackerspace :)
What's next? That's up to you.
How many times of day does your front door open?
What is the water level of your begonias?
Is your washing machine finished?
Is the coffee machine empty?
How loud are the kids playing music while you are out?
There are millions of possibilities for monitoring, just take a look around you. Does it do something? Then there must be a way to monitor it.
Here for the world to see is the temperature in my hackerspace<bs><bs><bs><bs><bs><bs><bs><bs><bs><bs><bs> living room:
https://xively.com/feeds/1238358657
Currently it is 26.5 degrees C, and it is supposed to be autumn.
Step 8:
I have not tested this code yet (but it does compile at least!)
This version should create temp_nnnnnnnnnnnn for each individual DS sensor and upload the data to xively.
Hopefully it will make the code multiple sensor friendly.
Greg
Attachments
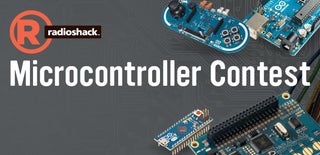
Participated in the
Microcontroller Contest
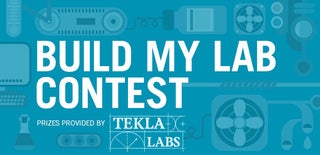
Participated in the
Build My Lab Contest