Introduction: Attiny Pocket Sequencer
Using an attiny85 I've developed this battery powered, pocket-sized sequencer which is fully programmable and usable in a studio setting, but in a smaller package. Here are some of the features:
-8 programmable steps
-8 LED display
-Potentiometer used for note frequency, tempo, and note length.
-Volume potentiometer.
-Onboard speaker.
-Mono audio-out 1/4 jack (selectable via a two-way switch)
-Two buttons, one for entering programming mode, one for selecting step or entering note length mode.
Besides making a pocket-sized sequencer, my goal of this project was to stretch the uses of the attiny chips to show how powerful they really are. This project is great for those interested in music and/or electronics, and by the end you will have one of the smallest, unique sequencers ever made!
Step 1: Parts
You will need these parts:
-Attiny 85 (with socket)
-Perfboard (I used 5 cm by 7 cm)
-Two 10k potentiometers
-Two tactile switch-buttons
-Two two-way switches
-A 7805 voltage regulator
-Two 10uF caps
-One 100uF cap
-One 2k resistor
-One 640 ohm resistor
-Eight LEDs
-74HC595 Shift Register
-1/4 inch audio female jack
-Speaker/buzzer
-9v Battery (with connector)
-(optional) 5cm by 7cm acrylic sheet
You will need these tools:
-Computer
-Another arduino (for programming the attiny85)
-Soldering Iron (with rosin)
-Hot Glue Gun
Step 2: Scheme! (The Schematic)
For simplicity, the schematic can be broken down into four parts: (I have attached pictures of each of the following)
1. Voltage Regulator
2. Switches and Potentiometers
3. 74HC595 and LEDs
4. Speaker/Audio out
1. The portion with the voltage regulator isn't all too complex. The positive terminal of our 9v battery will be connected to a switch, (to be used as an ON/OFF switch), then to a 100uF capacitor to ground, and finally into the voltage input (VI) pin on the 7805. Then the voltage output (VO) pin, we should connect a 10uF capacitor to ground and then connected to the attiny85's VCC pin. And of course, connect the ground of the battery to the 7805's ground pin.
2. The pins we are going to use for our switches and potentiometers will be pins 1 and 2. This will take a little explaining, as I had to cut corners to get maximum usability out of the attiny's pins. Pin 2 is the only pin that we have left that can be used and declared in the code, pin 1 is just the reset pin (so it is not usable unless you have a 12v ISP which we are not going to use). So lets talk about Pin 2. This pin will be connected, via a 640 Ohm resistor, to a 10k potentiometer's wiper pin (connect any other pin to ground). Then also connect a switch button which is connected directly to the pin (no resistor) and to ground.
OK so you may be thinking that is a little strange. Well it is but here's why. I found that I needed a button, and a potentiometer to make this sequencer, but I only had one pin. So after a bit of thought, I found that I could use a resistor on the potentiometer so that the analogRead(pot) would always return a value above about 30 (since there is always going to be some resistance on the potentiometer. Then I connected a button to ground so that when its pressed, analogRead(pot) would equal 0. (I also have the internal pull up resistor set ON). Now we can check the status of the button and potentiometer using only one pin.
As for pin 1, I have a switch connected to ground on it so that when it is pressed, the attiny resets and executes its "void setup()" function again. In the code I made it so that you must program the frequencies when the device starts. So this functions as a "program frequencies mode" button.
3. A 74HC595 is an 8-bit shift register. That means it can save up to one byte of information for us to use. Imagine 8 slots where you can either hold the value of HIGH or LOW (1 or 0). We can save data to this register to allow us to light eight LEDs with only three pins. (Data, latch, and clock). There's plenty more information on using shift registers with arduinos online. Here's a reference I find myself revisiting. Basically for the schematic, I just used the setup shown in the previous link. Just be sure you connect the data, clock, and latch to the correct pins since often times different models of shift registers can have weird names for these lines.
4. For our speaker/audio out, we are just going to have a 10k potentiometer, (set up as a variable resistor), connected to pin three and then to a 1uF capacitor which is connected to a switch that connects to either the on-board speaker, or a 1/4 audio out jack. The capacitor acts as a low pass filter, basically giving our sound a slight bass-boost.
Step 3: Breadboarding
Before we think about making our perfboard, lets put the circuit together on a breadboard just to make sure we've got a good understanding of how it works. I didn't add everything to my breadboard implementation, I left out the external speaker switch, the on/off switch, and other. Just do whatever you feel you need a better understanding on.
You can follow the schematic in the previous step to help make the circuit! Keep in mind you will have to know how to program an attiny85. Here is a great instructable that will tell you how.So leave some space on your breadboard for programming the chip!
Also, as always, I suggest keeping your breadboarded circuit for when you solder. I found that when something goes wrong I like to have a working circuit next to me to troubleshoot. When you are done making the circuit, check out the next step for the code.
Step 4: Using My Code
The code is actually rather simple, but since my readers come from many different backgrounds of knowledge I'll still go over the basics of my code.
As I mentioned before, I had to cut some corners to add extra buttons to the attiny so keep in mind that in my code whenever you see something like "if(analogRead(pot)<POT_THRESHOLD)" it means we are checking if the button was pressed.
So in my design of this sequencer, I wanted the user to program the steps right when the device is turned on. To do this I used the "setup()" function. That function is executed when the attiny is initially given power, or if its reset pin is set to LOW. I added a startup tone (which is a little arpeggio of a c major chord) to notify the user that they are in the frequency programming mode. To see the logic of programming the frequencies, see the setFrequencies() function. (Basically it goes through each step and sets the frequency equal to a value read by the potentiometer, and goes to the next step only when the button is pressed).
In the main loop ("loop()), the attiny is told to go through each step, and for each step, light the appropriate LED. Then play the note assigned to that step, at the specified note length. During this, the microcontroller is checking if the button (analogRead(pot)<30) is pressed. If it is, the program enters a function called "setSustain()". In this function, the user can select the notes length, (via the button and potentiometer).
That's all there is to the code, I hope it is straightforward enough for you. If it isn't I'd be glad to answer questions in the comments!
Attachments
Step 5: Planning the Perf
Before deciding on my final perfboard layout, I did lots of tinkering around with components to try to come up with a playable design. In the attached photos, you will see some basic designs that I tried before deciding on my current layout. Areas of concern while planning your perfboard are:
1. Placement of LEDs
2. Using space efficiently
3. Where/how to mount the 1/4 inch audio jack
3. Placement of potentiometers/buttons, etc...
If you come up with a sleek new design please share a picture in the comments, I'd love to see what you came up with!
Step 6: Soldering
Now lets solder this circuit together and get our pocket sequencer beeping.
While soldering this circuit I found that it was best to begin soldering the LEDs in place. After, cut the LED leads and you can use them for power/ground rails in your circuit. I connected all of the LEDs to ground using these and had some left over to help with the rest of the soldering.
Also, be sure that you have a bit of extra stranded wire since you'll be making lots of connections across the circuit.
Since this circuit is rather compact, there may be a few accidental shorts. To troubleshoot, I suggest sliding a utility knife between suspicious connections to check for these shorts.
When you're finished soldering, we can move on to making a case!
Step 7: Case
For my case, I went for simplicity. First, I cut a sheet of clear acrylic to 5cm x 7cm (the size of my perfboard). Next, using a dremel, I cut a square for the audio jack. The only two difficulties I came across were:
1. Accidentally cracking the acrylic (its rather fragile)
2. Ensuring that an 1/4 inch cable will fit in the jack (so that the cable does not hit the acrylic backing).
To make this device even more durable and sleek, you could make a case using a laser cutter or 3d printer. If you have these resources I'd love to see your results, since the size of this board makes it easy for anyone with a printer to print a case. Please share your results in the comments!
Step 8: Sequence! Beep Bop
Now you should have a working battery powered, pocket-sized sequencer! You can use it to make a retro 8-bit style tune, sound effects for games, to occupy you in a long car ride, or for some musical inspiration! I hope you have learned a bit about schematics, capacitors, using attinys, coding, shift registers, and how sequencers work. If you feel this instructable is deserving, give it a vote in either the RadioShack Battery Powered Contest, or the Epilog Contest... Or both! Thank you for your interest!
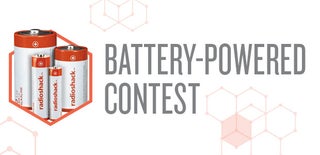
First Prize in the
Battery Powered Contest
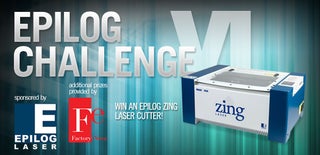
Participated in the
Epilog Challenge VI