Introduction: Backpack Anti-Theft Alarm
If you're a student then you have likely heard of someone's backpack being stolen on campus. To ease my mind I've designed a backpack ant-theft alarm. When activated, this device senses when a backpack is picked up and sounds an alarm to draw attention to the thief. The alarm is perfect for quiet places such as libraries or classrooms where a buzzer would be heard clearly (and cause enough of a scene to get you kicked out of the classroom/library too ;) ). It is a great project for beginners.
The backpack anti-theft alarm is powered by an ATtiny85 and functions using an ultrasonic range sensor (HC-SR04) to calculate distance from the backpack to the ground and a 5V buzzer to produce an alarm. Once the backpack is above a certain distance, the buzzer sounds and does not stop until the device is turned off. To provide the most security, you must enter a 5-bit binary code to turn the device off. This code is made using 5 SPDT switches, (by connecting them in a way that only one combination connects the circuit to ground). If mounted to a backpack correctly, this device can provide excellent security against backpack theft!
Throughout this instructable, you will learn a way of protecting your circuits using binary code, how to interact with the HC-SR04, how to use ATtiny85s, and you'll have a more secure anti-theft backpack! Let's get started with our parts list!
Step 1: Get Parts!
You'll need these parts:
-Project Box (3.34"L x 1.96"W x 0.83"H)
-10K Ohm Resistor
-330 Ohm Resistor
-Green 5mm LED
-HC-SR04
-Two CR2032s
-Coin-cell Battery Holder
-SPDT Slide Switches
-Perfboard
-ATtiny85 (with socket)
-5V Buzzer
-1N4007 Diode
-2N3904 Transistor (NPN general purpose)
-2.54mm Connectors
You'll need these tools:
-Computer
-Wire Stripper
-Dremel
-Soldering Iron (with rosin)
-Hot Glue Gun (with sticks)
-Multimeter (optional)
-Arduino (for programming ATtiny85)
Step 2: Solder Main Components
Let's begin by soldering our main components to our perfboard. Before you solder, make sure you map out which holes in your perfboard will line up correctly with the project box. Check that the HC-SR04 and buzzer are positioned correctly so that they will be able to protrude from the enclosure later on.
Now that you have an idea of what goes where, heat up your soldering iron and solder the HC-SR04, coin-cell holder, buzzer, female 2.54mm connector, and 8-pin DIP socket to the perfboard.
When all your major components are soldered in the correct positions on your perfboard, we can start making our 5-bit security switch!
Step 3: Make Security Switch
Our security switch is going to ensure that only the owner of the backpack alarm can turn it off. Using 5 SPDT switches, we can make a single 5-bit security switch which is off only when one combination of 0s and 1s are entered.
To start the switch, use your wire cutter (or metal cutter) to snip any excess metal on your switches (see pictures). Then hot glue each switch to the last. Make sure you hot glue both sides to make a firm bond. Continue these steps until all 5 switches are straightly and strongly bonded together.
To get our security switch working we will have to move on to the next step to wire our switches!
Step 4: Wire Security Switch
To wire the switch requires a little knowledge about SPDT switches. Single Pole Double Throw switches have two positions and connects the center terminal to either the upper or lower terminal. This switch is perfect for our purposes. We can treat the upper terminals as 1, and lower as 0s.
We will use two wires that we can name: GND and OUT. First, connect all the center terminals to OUT. To make the switches code, connect GND to either the upper or lower terminal on each switch and randomly alternate to create a pattern. Each terminal that is left with no connection will be the correct code to turn off our switch. This is because if any switch is not in the correct position, it will connect the circuit. You have to have all 5 switches in the correct position to turn the device off. Finally, connect our GND and OUT wires to a 2.54mm two pin male connector. To protect your switch, use hot glue to hold all loose wires in place.
When your security switch is connected properly, and you have wired your 2.54mm male connector, we can finish soldering the main perfboard.
Step 5: Solder Connections
Now that we have soldered the security switch and main components, we can finish the circuit by connecting our main components to the ATtiny85!
Using the attached schematic (see 5th picture) connect the HC-SR04, buzzer, indicator LED, power supply, and security switch to the appropriate arduino pins. In case you have difficulties reading a schematic, here are the connections that you will have to make:
-For the HC-SR04: VCC -> 5V, TRIG -> ATtiny pin 7. ECHO -> ATtiny pin 3, GND -> GND.
-For the indicator LED, connect a 330 ohm resistor to the positive lead on your 5mm LED then connect the negative lead to GND (all in parallel with the ATtiny85).
-For the "power supply" all we need to do is connect a 1N4007 from the 6V supply (coming from our two 2032s) to the ATtiny's VCC (pin 8). This is to drop the voltage down to a safe operating voltage for the ATtiny85 (it must be no higher than 6V).
-To get the buzzer working, we need a transistor. This is because we want the buzzer to be as loud as possible, and if we just supplied it with an ATtiny pin, it wouldn't have much current (or voltage). So connect ATtiny pin 5 to the base of a transistor via a 10k resistor. Next connect 6V from our batteries to the transistor's collector, and the buzzer to its emitter. Finally connect the other end of the buzzer to GND.
-To connect the security switch, connect GND to the previously named GND connection on the switch and finally, OUT to the GND of our ATtiny85 chip.
Now our circuit should be connected and ready for the code.If you're having trouble with the circuit, refer back to the schematic and don't hesitate to ask a question in the comments. Let's move onto uploading the code in the next step!
Step 6: Upload Code
The code is very simple, it should even be easy for all you beginners! If you're new to coding I suggest looking at the code and thinking of some lines as just some psudo-code. For example, check out this code:
//getDistance:
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration/2) / 29.1;
Looks confusing, right? Well actually it is! So it is best to just think of all those lines as one function that gets the distance. Interfacing with a complex module like the HC-SR04 can be confusing and that's why there are libraries written for it!
Be sure you look for some good documentation on how to program an ATtiny85! You will need another arduino (or programmer). Here is my favorite reference.
If my code confuses you or you are not quite code literate, please continue reading to learn about the basics of my code. (If this still doesn't answer your questions please comment below!). The first few lines of my code are just declarations and definitions of variables all of which are basic arduino syntax. In the setup() function I declare the pin modes to tell arduino what pins i'll be using for input or output. Also, I add a short delay for the user to get the device situated so that the alarm doesn't accidentally go off while setting it up. Finally, there is the loop() function. This function continuously checks the distance between the HC-SR04 and any object in front of it. If it ever exceeds the constant ALARM_DISTANCE, then the boolean variable "alarm" will become true. If that variable is true, the loop() executes a few lines to sound the alarm.
Finally our alarm should be beeping and working identically to the video shown in the introduction! However, it is still missing one thing: the case!
Step 7: Dremel Enclosure
To make the circuit fit in the enclosure, we need to dremel holes for the HC-SR04, buzzer, security switch and indicator LED. This should be a matter of guess and check for most of the way (as dremel work usually is), but there are some tips to help you out.
-Make sure you try to outline your cuts with ink before you begin so you know where and how large each cut should be.
-Be careful not to cut too much plastic out where you don't need to.
-Always cut from the inside so you don't accidentally scratch the outside of the case.
-Dremels are very dangerous. Wear gloves and stay away from the tip!
Once all the pieces of our circuit fit snug in their spaces, we can mount the security switch!
Step 8: Hot Glue Security Switch
Since our enclosure doesn't have room for screw mounting the switches, we have to mount them using either hot glue or epoxy. Make sure you place your security switch into your dremeled enclosure ensuring that it is straight and aligned in the correct direction.
Heat up your hot glue gun and use generous amounts of glue to mount the security switch to the project box. Use glue on both the outside and inside; however, be careful not to get glue inside of any of the switches since that will cause the switches to stop conducting.
Make sure your hot glue doesn't rise too far above the case since we will be closing the enclosure in our next step!
Step 9: Close Enclosure
Finally the circuit is complete, the case is all dremeled out, and the security switch is mounted! Now we can just snap on the back of the case and mount it right? Well most of the time it'll be that easy; however, we have to be careful that no components inhibit the back panel from snapping in,.
If you have trouble closing the case, check to see if any hot glue is blocking the case from closing and use a utility knife to cut it away. Also make sure no transistors, diodes, or leads are jutting out from the case that could prevent the enclosure from closing. Finally, check to see if the HC-SR04, buzzer, and LED protrude from the enclosure. If they aren't this could be stopping the case from closing. Use a dremel tool to enlarge the cuts you made before, so that the components slide through the holes and make room for the back panel.
Now that your backpack anti-theft alarm is all closed up and functional, we can mount it to your backpack!
Step 10: Mount It!
To use your new backpack anti-theft alarm, you will need to mount it to your backpack securely. In the event of a theft,,you don't want the thief to be able to simply remove the alarm. In the attached pictures, I show a simple way to quickly mount it to just about any backpack; however, it isn't the most secure option.
To make a more safe alarm you could mount using:
-Epoxy or hot glue
-Backpack clips
-Velcro
-Thread
If you find a secure way to mount your backpack anti-security alarm, please comment below with an attached picture!
Step 11: Secure Your Backpack!
Finally you can enjoy the security of having your own backpack anti-theft alarm for some ease of mind this school year! I hope you've learned a lot about HC-SR04s, ATtinys, buzzers, making a security switch, and using the dremel tool. If you enjoyed this article and would like to support me, please vote for this instructable in the DIY University, Tech, or Microcontroller contests! Click here for more fun and rewarding projects. Thanks for your interest and enjoy your new device!
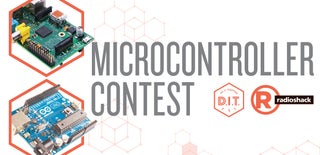
Runner Up in the
Microcontroller Contest
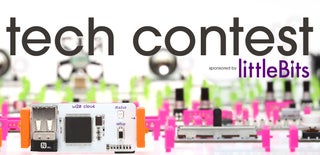
Participated in the
Tech Contest
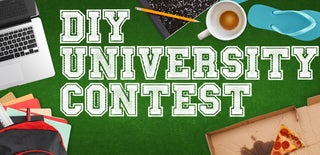
Participated in the
DIY University Contest