Introduction: DIY: Arduino Based Continuous Touch Piano
In this instructable, working and making of Arduino based piano is explained. This piano is able to play any frequency in-between two keys. Making of this would require basic knowledge of Arduino for debugging.
Have a view of video for working and making.
Features:
- A piano that takes input from the touch,
- Able to play any frequency in between two keys,
- Adjustable frequency range,
- The response of piano should fast enough.
Step 1: How It Works:
So, first we break our problem into multiple smaller problem and then try to solve them one by one
in our case to make piano major tasks are:
- Measuring/estimating positions of touch:
Here we do not need to have just one value of touch but we need to measure the position of multiple touches which also should be the analog value. so that we can take care of frequency which is in between to keys. this involves taking data from keys and also processing of that data.
- key and frequency calculation:
After taking data from all keys, we need to map that value for specific frequency. As we know increment of the frequency of piano is Geometric progression, which means you need to keep multiplying some factor to get the frequency of upcoming key.
- Tone generation and rejection of noise(in data):
After calculating frequency we need to generate the approximate waveform of that frequency. there is also some noise may present during no touch condition which needs to eliminated.
if you do not want to know how it works but just interested in making of it skip to step no:4
Step 2: Measuring Touch Position:
We are going to use capacitive touch for our purpose. here we will use an array of plates. we will use each of plate as the touch sensor.
The touch sensor is nothing but capacitor sensor, we are somehow measuring the change in capacitance (which is a result of touching) using Arduino. There are two pins are used both are connected with a high value of the resistor. We turn ON pin to high and start the timer and see how much time it takes for another pin to go at high state. This time which we call "rise time" depends upon capacitance and also resistance. Here resistance is constant but capacitance varies as per touch.
here in the image, we have taken around 12 keys for example. and touching somewhere between plate 6 and 7. here we need continuous data. we are not interested to know whether it is at 6th or 7th key. but we actually want to know the exact position of the touch in between 6-7. so we first detect key of max touch and then measure the position of the touch by using basic mathematics.
Image 1: http://playground.arduino.cc/Main/CapacitiveSensor...
Step 3: Determining Frequency & Tone Generation:
After calculating key number we need to calculate frequency associated with that key. The frequency of key is in geometric progression. The function of it is shown in the image.
In above function "n" is a number of keys. so adding a number of key we can calculate the frequency.
Here we map key 1-18 (a board with 18 touch plates) to any keys we want. and as our device is analog no need to take the range of only 18 key but any range.
for an example, if you want to play on 40th to 70th key on the keyboard that is mapped on 1-18. so left most part would play frequency of 40th key and right part would play frequency of 70th key.
Here we would use direct tone function for frequency generation. It generates the square wave of given frequency. for a better experience, we also can set a duty cycle for volume control as the pressure of touch.
for more reading related to piano key frequency:
https://en.wikipedia.org/wiki/Piano_key_frequencie...
Step 4: Making of Piano:
So now let's start making, you will need the following material/component :
- A wooden block: Length of around 20cm, 7-9cm width and 5-10mm thickness.
- Aluminum foil that sufficient to cover block,
- Resistors: 20x of 87k, 1x of 17k,
- Wires for connection,
- Breadboard,
- Speaker,
- Glue,
Except these, you will need tools like:
- Soldering iron,
- Glue gun,
- Stapler,
- Sand paper,
- Paper cutter,
- Ruler scale,
Step 5: Preparing Board:
First take a wooden block as given size and stick aluminum foil on it. Remove extra foil from it and cut it to 30% of width as shown in the 4th image.
Now do marking on it at 12mm apart along the length. you can even try the lower size of a key for the better result.
Now cut these foil as per marking so that we would have separate plates which we will use as touch key/plates.
Now cover Al foil with plastic tape. So that you don't need to touch plates directly.
Step 6: Making Electric Connection on It:
- Check that all plates are separated from each other, they must be electrically insulated. all plate must check after every step,
- Now connect the 87K resistor to all plates and solder it properly,
- connect another end of the resistor to common and connect to pin 4. and take a wire from every plate (another end of the resistor),
- also connect pin 12 to speaker.
now connect:
- KEY 1 TO A6
- KEY 2 TO 13
- KEY 3 TO A5
- KEY 4 TO A4
- KEY 5 TO A3
- KEY 6 TO A2
- KEY 7 TO A1
- KEY 8 TO A7
- KEY 9 TO 2
- KEY 10 TO 3
- KEY 11 TO 5
- KEY 12 TO 6
- KEY 13 TO 7
- KEY 14 TO 8
- KEY 15 TO 9
- KEY 16 TO 10
- KEY 17 TO 11
you can take you own combination but then arrange it such a way that value of 'i'th key in array x[i].
Step 7: Understanding Code:
- First, you need to install CapacitanceSensor library.
float N_min=48;
float N_max=70;
here the select range of key. for example in this code 48th to 70th is mapped on our board.
2.smoothing: this shows the value of smoothing. more value than higher lag would be there.
int smooth=2;
3.Specifying capacitance value:
CapacitiveSensor i20 = CapacitiveSensor(4,A6);
CapacitiveSensor i13 = CapacitiveSensor(4,13);
.
.
.
CapacitiveSensor i11 = CapacitiveSensor(4,11);
this section specify various pin as input where pin 4 in common transmitter pin.
4. Mapping values:
N_map();
map range of frequency as per N_min and N_max value on each plate.
5. Read value: Read_val() is function that run following code which take value from each plate.
x[1] = i20.capacitiveSensor(30);
x[2] = i13.capacitiveSensor(30);
.
.
x[18] = i11.capacitiveSensor(30);
6. key_calc(); =this function reads calculation of key as per min and max value of key.
for(int z=1;z<19;z++){ if(x[z]>t1){t1=x[z];t4=z;} : this would detect maximum value of touch.
kt=((x[t4-1]*N[t4-1])+(x[t4]*N[t4])+(x[t4+1]*N[t4+1]))/(x[t4-1]+x[t4]+x[t4+1]);
this would take 1 key forward and backward and take value from each and calculate position of touch as per intensity.
7. freq_calc(); Now we need to calculate the frequency of that key.
float n;
n=(key-49)/12;
freq=m*220*pow(2,n);
here, 'key' is the number of keys
m= it is the value which only becomes 1 when the touch input is high enough. so without any significant kind of touch frequency value would be equal to 0.
Attachments
Step 8: Uploading Code and Debugging:
after you upload code it may or may not work properly. So here are some of the problem that I observed during this project.
- One of the most common problems is " loose connection between Arduino and plate. you won't get continuous sound without proper connection.
- if two nearby plates are touching each other, it will have the same value of capacitance.
- the speaker you are using is not suitable with Arduino. so you need to use another speaker or an amplifier circuit.
May be, this piano won't work with battery due to lack of proper ground and work best if powered by use of PC as it has a good ground.
How to detect the problem:
- you can turn on print_raw() and then touch all plates together to see which plate is not responding properly.
- you can also check all plates one by one by uploading different code to it.
Step 9: Finally...
Limitations:
- the sound of this piano is not as good to hear as piano as it is a square wave wherein actual piano it as the combination of multiple harmonics.
- you can not play multiple keys at the time,
- the volume of piano is not controlled by touch but remain fixed.
set key range and smoothness number as per your style, and locate various notes on it so that you can play any song you like.
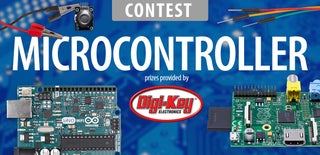
Participated in the
Microcontroller Contest 2017
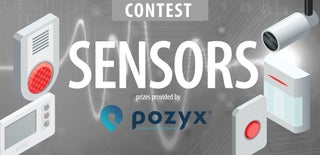
Participated in the
Sensors Contest 2017