Introduction: Holiday Dice Roller
This is a fun project, based on Arduino. I chose a holiday theme for the box, but you can, of course, use any style of box you like.
This device rolls two dice at random.
I also added a twist...there is a "secret" button that you can push for random doubles! Baffle your opponents by always rolling doubles!
Here's a video showing how it works:
Step 1: Hardware Assembly
I cut out a piece of wood and drilled holes in it to mount the LED's in. It looks better if they don't protrude above the surface of the outside face of the wood.
I used 14 typical LED's, each with a 100ohm resister in-line and connected to the Arduino digital pins as follows:
Left Die:
upper row, left to right: 10,9,8
middle: 7
lower row, l to r: 11,12,13
Right Die:
upper row, left to right: 3,2,1
middle: 0
lower row, l to r: 4,5,6
Note: I made one die green and the other red in the spirit of the holidays!
Then I connected an on/off switch (in-line with the 9V battery) and a "roll" button (a momentary switch connected to Arduino Analog A0 pin...which can be referenced as digital pin 14 in the code).
I also put a "secret" switch under the green ribbon (a momentary switch connected to Arduino Analog A1 pin...which can be referenced as digital pin 15 in the code). If you push this before your roll, you always get doubles!
Step 2: The Code (Arduino Sketch)
Here is the code. Download this into your Arduino. You can ignore the print lines because that was used for debugging.
const int rollButton = 14;
const int secretButton = 15;
const int diceOne = 0;
const int diceTwo = 6; //add 6 to adress pins of second die
int rollButtonState = HIGH; // the current reading from the ROLL pin. HIGH = not pressed
int lastRollButtonState = HIGH; // the previous reading from the ROLL pin
int secretButtonState = HIGH; // the current reading from the SECRET pin
int lastSecretButtonState = HIGH; // the previous reading from the SECRET pin
// the following variables are long's because the time, measured in miliseconds,
// will quickly become a bigger number than can be stored in an int.
long lastSecretDebounceTime = 0; // the last time the output pin was toggled
long lastRollDebounceTime = 0; // the last time the output pin was toggled
long debounceDelay = 55; // the debounce time; increase if the output flickers
void setup() {
pinMode(0, OUTPUT); // first die LED's
pinMode(1, OUTPUT);
pinMode(2, OUTPUT);
pinMode(3, OUTPUT);
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
pinMode(7, OUTPUT); // second die LED's
pinMode(8, OUTPUT);
pinMode(9, OUTPUT);
pinMode(10, OUTPUT);
pinMode(11, OUTPUT);
pinMode(12, OUTPUT);
pinMode(13, OUTPUT);
pinMode(rollButton, INPUT); // sets A0 pin as digital input; this is ROLL button
digitalWrite(rollButton, HIGH); // turn on pullup resistor
pinMode(secretButton, INPUT); // sets A1 as digital input; this is SECRET button
digitalWrite(secretButton, HIGH); // turn on pullup resistor
// Serial.begin(9600);
randomSeed(analogRead(3)); // if analog input pin 3 is unconnected, random analog
// noise will cause the call to randomSeed() to generate
// different seed numbers each time the sketch runs.
// randomSeed() will then shuffle the random function.
clearAll();
lightOne(0); // set both dice to lights ONES while waiting for ROLL switch to be pressed
lightOne(7);
}
void loop() {
Serial.print(rollButtonState);
Serial.println(" = Roll Button State");
Serial.println("MAIN LOOP");
checkRollButton();
checkSecretButton();
if (rollButtonState == LOW) // button pressed = LOW
{
blinkAll(300, 3); // blink of & all led's 3 times, 300 ms apart, and then roll
}
}
void checkSecretButton() {
// read the state of the switch into a local variable:
int reading = digitalRead(secretButton);
// check to see if you just pressed the button
// (i.e. the input went from HIGH to LOW), and you've waited
// long enough to ignore any noise:
// If the switch went low, due to noise or pressing:
if (reading == LOW) {
// wait the debouncing time
delay(debounceDelay);
reading = digitalRead(secretButton);
if (reading == LOW) //reading is still low after debounce delay
{
secretButtonState = LOW;
Serial.println("secretRoll routine set LOW");
lastSecretButtonState = LOW; //this remembers the button was pushed and can only be reset after a secret roll
}
else
{secretButtonState = HIGH;
}
}
else
{secretButtonState = HIGH;
}
}
void checkRollButton() {
// read the state of the switch into a local variable:
int reading = digitalRead(rollButton);
// check to see if you just pressed the button
// (i.e. the input went from HIGH to LOW), and you've waited
// long enough to ignore any noise:
// If the switch went low, due to noise or pressing:
if (reading == LOW) {
// wait the debouncing time
delay(debounceDelay);
reading = digitalRead(rollButton);
if (reading == LOW) //reading is still low after debounce delay
{rollButtonState = LOW;
Serial.println("CheckRoll routine set LOW");
}
else
{rollButtonState = HIGH;
}
}
else
{rollButtonState = HIGH;
}
}
void lightOne(int diceNumber) {
Serial.println("Rolled ONE");
digitalWrite(diceNumber, HIGH);
}
void lightTwo(int diceNumber) {
Serial.println("Rolled TWO");
digitalWrite(1+diceNumber, HIGH);
digitalWrite(4+diceNumber, HIGH);
}
void lightThree(int diceNumber) {
Serial.println("Rolled THREE");
digitalWrite(1+diceNumber, HIGH);
digitalWrite(4+diceNumber, HIGH);
digitalWrite(0+diceNumber, HIGH);
}
void lightFour(int diceNumber) {
Serial.println("Rolled FOUR");
digitalWrite(3+diceNumber, HIGH);
digitalWrite(1+diceNumber, HIGH);
digitalWrite(4+diceNumber, HIGH);
digitalWrite(6+diceNumber, HIGH);
}
void lightFive(int diceNumber) {
Serial.println("Rolled FIVE");
digitalWrite(1+diceNumber, HIGH);
digitalWrite(3+diceNumber, HIGH);
digitalWrite(4+diceNumber, HIGH);
digitalWrite(6+diceNumber, HIGH);
digitalWrite(0+diceNumber, HIGH);
}
void lightSix(int diceNumber) {
Serial.println("Rolled SIX");
digitalWrite(1+diceNumber, HIGH);
digitalWrite(2+diceNumber, HIGH);
digitalWrite(3+diceNumber, HIGH);
digitalWrite(4+diceNumber, HIGH);
digitalWrite(5+diceNumber, HIGH);
digitalWrite(6+diceNumber, HIGH);
}
void clearAll() {
digitalWrite(0, LOW);
digitalWrite(1, LOW);
digitalWrite(2, LOW);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW);
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
}
void blinkAll(int t, int n) {
clearAll();
for(int x=0; x < n; x++) { // Loop n times
digitalWrite(0, LOW);
digitalWrite(1, LOW);
digitalWrite(2, LOW);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
digitalWrite(7, HIGH);
digitalWrite(8, HIGH);
digitalWrite(9, HIGH);
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
delay(t);
digitalWrite(0, HIGH);
digitalWrite(1, HIGH);
digitalWrite(2, HIGH);
digitalWrite(3, HIGH);
digitalWrite(4, HIGH);
digitalWrite(5, HIGH);
digitalWrite(6, HIGH);
digitalWrite(7, LOW);
digitalWrite(8, LOW);
digitalWrite(9, LOW);
digitalWrite(10, LOW);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
digitalWrite(13, LOW);
delay(t);
}
clearAll();
//first check if secret button was previously pushed
if (lastSecretButtonState == LOW) // if secret button has been pressed, lastSecretButtonState will still be LOW
{
secretDiceRoll();
}
else
{
randomDiceRoll();
}
}
void randomDiceRoll() {
int randNumber=6;
/*
//test code
clearAll();
lightOne(diceOne); // set both dice to lights ONES while waiting for ROLL switch to be pressed
lightOne(diceTwo);
*/
Serial.println("entered Random number code");
Serial.print(rollButtonState);
Serial.println(" = Roll Button State");
for(int d=0; d<8; d=d+7) { // Do once for ea die
// get a random number from 1 to 6
randNumber = random(6)+1;
switch (randNumber) {
case 1:
lightOne(d);
break;
case 2:
lightTwo(d);
break;
case 3:
lightThree(d);
break;
case 4:
lightFour(d);
break;
case 5:
lightFive(d);
break;
case 6:
lightSix(d);
break;
default:
clearAll();
break;
}
}
rollButtonState = HIGH;
Serial.println("EXITed Random number code");
Serial.print(rollButtonState);
Serial.println(" = Roll Button State");
Serial.println("");
}
void secretDiceRoll() { //secret button causes random doubles
int secretRandNumber=6;
secretRandNumber = random(6)+1;
switch (secretRandNumber) {
case 1:
{lightOne(0); lightOne(7); break;}
case 2:
{lightTwo(0); lightTwo(7); break;}
case 3:
{lightThree(0); lightThree(7); break;}
case 4:
{lightFour(0); lightFour(7); break;}
case 5:
{lightFive(0); lightFive(7); break;}
case 6:
{lightSix(0); lightSix(7); break;}
default:
{clearAll(); break;}
}
secretButtonState = HIGH;
lastSecretButtonState = HIGH;
}
Step 3: Finish!
After you've downloaded the code, plug in the 9v battery, carefully route the wires in the box, close up the box, and enjoy!
Here's a video showing how it works:
http://www.youtube.com/watch?v=Brk-Qv1YEJ8
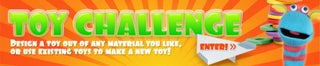
Participated in the
Toy Challenge 2
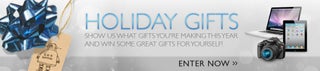
Participated in the
Holiday Gifts Challenge
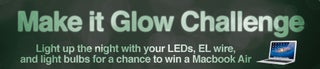
Participated in the
Make It Glow Challenge
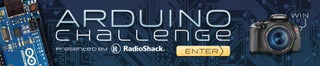
Participated in the
Arduino Challenge