Introduction: How to Connect Arduino to a PC Through the Serial Port
To do that I have used a computer with Ubuntu 12.04 and the program language C++, but if you want to used Windows the code works too, only have to change the port used to conect with arduino, but this is explain in the next step.
This program is only the first version so it must be improved.
Arduino use the usb port to simulate a serial port so we have to use a usb cable to connect the arduino usb port to computer usb port.
Step 1: Program in C++
This is the code of C++, I have created a main class and a Arduino class, so this is object oriented.
#ifndef ARDUINO_H
#define ARDUINO_H
#include <SerialStream.h>
#include <SerialStreamBuf.h>
#include <SerialPort.h>
#include <string>
class Arduino{
public:
Arduino();
int open);
DataBuffer read();
void close();
private:
string dev = "/dev/ACM0";
SerialPort serial;
};
#endif // ARDUINO_H
This is the header of Arduino class.
There are three functions open, read and close.
Open: Open the conection bewteen arduino and the computer.
Read: Read the bufer where is all dates that arduino has send to the computer.
Close: Close the conection bewteen arduino and the computer.
To connect with arduino I have used the port of my computer "/dev/ACM0", if you use Windows instead of Linux you have to use the port "COM1" or "COM2". But to see what port is using arduino you have to use the JDK of arduino and select a port in "Tools -> Serial Port".
# include <Arduino.h>
Arduino::Arduino(){
serial(dev);
}
int Arduino::abrir(){
int estado = 0;
serial.Open(SerialPort::BAUD_9600,
SerialPort::CHAR_SIZE_8,
SerialPort::PARITY_NONE,
SerialPort::STOP_BITS_1,
SerialPort::FLOW_CONTROL_NONE);
if (serial.IsOpen() == false)
estado = -1;
return estado;
}
void Arduino::cerrar(){
serial.Close();
}
DataBuffer Arduino::leer(){
SerialPort::DataBuffer buffer;
serial.Read(buffer, 10, 1000);
return buffer;
}
This is the code of Arduino class.
#include <iostream>
#include <SerialStream.h>
#include <SerialStreamBuf.h>
#include <SerialPort.h>
#include <string>
using namespace std;
using namespace LibSerial;
int main(int argc, char **argv)
{
Arduino arduino();
return 0;
}
And finally this is the main class.
Step 2: Program in Arduino
void setup(){
Serial.begin(9600);
}
void loop(){
Serial.println("Hello world");
delay(1000);
}
This is the code that you have to do in arduino.
This code is very simple.
"Serial.begin(9600)" : Sets the velocity of dates in bits per second (baud).
Serial.println("Hello world") : Send a message through the serial port.
delay(1000) : Stop by 1000 miliseconds.
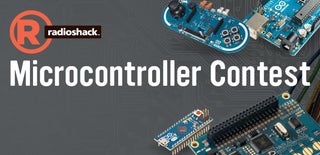
Participated in the
Microcontroller Contest