Introduction: Interfacing ADXL335 With ARDUINO
As the datasheet says, ADXL335 is a small, thin, low power, complete 3-axis accelero-meter with signal conditioned voltage outputs. The product measures acceleration with a minimum full-scale range of ±3 g. It can measure the static acceleration of gravity in tilt-sensing applications, as well as dynamic acceleration resulting from motion, shock, or vibration.
ADXL335 is 3v3 compatible device, it's powered by a 3.3v source and also generates 3.3v peak outputs. It has three outputs for each axis i.e. X, Y & Z. These are analog outputs and thus require an ADC in a micro-controller. Arduino solves this problem. We will be using the analog functions of Arduino.
Step 1: Parts Required
- Arduino-Any Arduino/clone will do but I have used Freeduino v1.16
- ADXL335 accelerometer
- Connecting wires(Relimates)
- USB cable for connecting Arduino with your Laptop
Step 2: The Circuit
- GND-To be connected to Arduino's GND
- VCC-To be connected to Arduino's 5V
- X-To be connected to Analog Pin A5
- Y-To be connected to Analog Pin A4
- Z-To be connected to Analog Pin A3
Use 2-pin relimate for connecting Vcc and GND.
Use a 3-pin relimate for connecting X, Y & Z outputs.
Also connect AREF pin to the 3.3v. This is done to set the reference voltage to 3.3v because the output of ADXL335 is 3.3v compatible.
Step 3: Code
//connect 3.3v to AREF
const int ap1 = A5;
const int ap2 = A4;
const int ap3 = A3;
int sv1 = 0;
int ov1 = 0;
int sv2 = 0;
int ov2= 0;
int sv3 = 0;
int ov3= 0;
void setup() {
// initialize serial communications at 9600 bps:
Serial.begin(9600);
}
void loop() {
analogReference(EXTERNAL); //connect 3.3v to AREF
// read the analog in value:
sv1 = analogRead(ap1);
// map it to the range of the analog out:
ov1 = map(sv1, 0, 1023, 0, 255);
// change the analog out value:
delay(2);
//
sv2 = analogRead(ap2);
ov2 = map(sv2, 0, 1023, 0, 255);
//
delay(2);
//
sv3 = analogRead(ap3);
ov3 = map(sv3, 0, 1023, 0, 255);
// print the results to the serial monitor:
Serial.print("Xsensor1 = " );
Serial.print(sv1);
Serial.print("\t output1 = ");
Serial.println(ov1);
Serial.print("Ysensor2 = " );
Serial.print(sv2);
Serial.print("\t output2 = ");
Serial.println(ov2);
Serial.print("Zsensor3 = " );
Serial.print(sv3);
Serial.print("\t output3 = ");
Serial.println(ov3);
delay(3000);
}
Step 4: Serial Monitor
We will be displaying two different values for one analog value read. The first value is the ADC converted value in 10-bit resolution(0 to 1023) while the second one is mapped for PWM and it is in 8-bit resolution(0 to 255).
Three values for X, Y & Z axes are displayed together are repeated after an interval of 3 seconds.
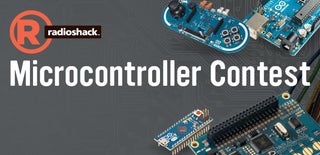
Participated in the
Microcontroller Contest