Introduction: Make an Attiny13 Based IR Proximity Sensor for $2.42
This is an extremely cheap IR proximity sensor you can make with a few cheap parts and an AVR programmer. I use an Arduino as my programmer in this Instructable.
This sensor only has a range of about 3 inches. You can easily add more LEDs or brighter ones to extend the range. You can also easily re-arrange the LEDS to detect when a beam is broken as well.
The design takes advantage of a cheap AVR (computer on a chip). The computer pulses the IR LEDs off and on and compares the analog readings from the sensor in each state. When the reading with the lights on is above the reading with the lights off the sense pin goes high indicating the sensor is seeing it's own (reflected) light. There is an LED on the sense indicator in this design so you can see when the sensor engages. You can connect the signal right to a microcontroller like an Arduino or Picaxe.
This design moves some processing out of your main robot brain and into it's own node. You may want to debounce the signal, but you don't have to flash the leds and take the readings. You can also use just one digital pin to take the reading. The sketch is around 700K out of 1024 available.
Why I built this
I'm way out of high school, but this is part of a series of designs related to bringing the robots from the game Robot Oddysey into the real world. I want to allow grade schoolers the same chance to learn robotics I had. So I am working on building really inexpensive robots that can move in 8 directions without turning. The "bumpers" are complete now.
Follow @dustin1970
Step 1: Gather Materials
Quantity |
Digikey Part Number |
Description |
Cost |
1 |
475-1439-ND |
PHOTOTRANSISTOR NPN W/FILTER 5MM |
$0.53 |
1 |
ATTINY13A-PU-ND |
IC MCU AVR 1K FLASH 20MHZ 8PDIP |
$0.95 |
3 |
CF14JT220RCT-ND |
RES 220 OHM 1/4W 5% CARBON FILM |
$0.24 |
1 |
CF14JT1M00CT-ND |
RES 1M OHM 1/4W 5% CARBON FILM |
$0.08 |
1 |
2N3904FS-ND |
IC TRANS NPN SS GP 200MA TO-92 |
$0.18 |
2 |
754-1241-ND |
EMITTER IR 3MM 940NM WATER CLEAR |
$0.44 |
TOTAL |
$2.42 |
You will also need
- Electrical tape
- plastic drinking straw
- scissors
- Wire snippers
- breadboard and/or soldering iron
- Jumper wires
- An AVR programmer (any Ardunio compatible will do)
- Ardunio 1.0 software with modifications to program Attiny and the Atiny13 core
Step 2: Create a Sensor Shroud
You don't want IR light leaking in from the sides of the sensor. We will create a little tunnel from electrical tape and a straw. My tape is opaque to IR. Yours probably is too but you can line it with aluminun foil if you want to be sure. Make sure no metal is exposed because it will ruin the circuit.
Cut off about 1.5" (4cm) of the straw. Wrap it in electrical tape. Trim the ends and make it neat.
Cut about 1/2" (8mm) bit of tape and roll it up from the short edge. Fold the roll in half. It won't stay but put a good crease in it. This will plug the bottom of the sensor and keep stray light out that way.
Step 3: Prepare the Sensor
Cut the middle leg off your phototransistor if it has one. It's not needed in this design and can actually cause problems if you leave it on. You don't have to cut it all the way back, but don't leave very much.
Gently wiggle the sensor into the straw until the base is 1/4" (5mm) in or so. Push in the folded roll of tape behind it and seal the back with a bit of tape.
Step 4: Wire It Up
Follow the wiring diagram and keep some notes in mind.
- The chip, LEDs and transistors only work one way around.
- The short leg of the LEDS is connected to the 220 ohm resistors.
- The long leg of the phototransistor is connected to the 1meg resistor.
- You can add more IR emitter/resistor pairs in parallel with the existing pair. Get the basic circuit working first. You could also just use one IR emitter, but 2 seemed to make the readings a lot more stable.
Attachments
Step 5: Wire Up the AVR (Arduino) and Program the Chip
Install Attiny13 Core13 from sourceforge. Here is an Instructable by diy_bloke with directions and even a zip file to download.
Here is MIT's instructions for modifying your environment to burn Attiny.
This page might help if you have problems. Check the comments.
Wire in the Arduino. You can unwire it when the programming is done.
Load the INO file and burn it to the Attiny13 chip.
Alternately just use an AVR programmer to burn the hex file or Instructable author nikkipugh has a super strip board shield design for programming attiny13 chips.
You may need a 10uf capacitor or 120 ohm resistor from the Arduino's reset to ground.
code:
/* Attiny13 proximity sensor by Dustin Andrews 2012 License: Creative Commons Unported http://creativecommons.org/licenses/by/3.0/ */ //Do some low level AVR pin writing to save instructions. 1010 of 1024. Close! #define SetPin(Bit) PORTB |= (1 << Bit) #define ClearPin(Bit) PORTB &= ~(1 << Bit) //wire your IR led to this pin. (chip pin 5?); #define irOutPin 3 //This pin goes high when the sensor detects and obstacle. (chip pin 6) #define outPin 1 // wire this pin to your phototrans vcc-->phototrans-->pin4-->1M res-->gnd(chip pin3) #define sensorInPin 2 void setup() { pinMode(irOutPin, OUTPUT); pinMode(outPin, OUTPUT); //pinMode(2, OUTPUT); analogReference(0);//analogread won't work on Atiny13 w/o this line. Won't work on others WITH it prob'ly. } void loop() { static long difference = 0; unsigned static long lastTime = 0; SetPin(irOutPin); delay1(); unsigned int r1 = analogRead(sensorInPin); ClearPin(irOutPin); delay1(); unsigned int r2 = analogRead(sensorInPin); difference += r1 - r2; if(millis() - lastTime > 5)//might get a smoother response with bigger delays { if(difference > 0) { SetPin(outPin); } else { ClearPin(outPin); } difference = 0; lastTime = millis(); } } void delay1() { //delay(1) is too costly in memory. Why is this so much cheaper? :D long start = millis(); while(true) { if(millis() - start > 1){break;} } }
Step 6: Build Some Awesome Robot With the Sensor
Troubleshooting:
- Check all the wires.
- Wiggle the LEDs and make sure they align well with the barrel.
- Check the wires again.
- Have someone look over your shoulder while you explain where each wire goes. (This helps so often it's not even funny)
- Check the polarity of the LEDs and sensor and transistor.
- Check the polarity of the Attiny. If it's hot you put in in backwards.
- Use a phone or other digital camera to check that the LEDs are glowing.
- Turn off any flourescent lights and try the sensor away from sunlight.
- Take one the LEDs and attach it to long wires and put it right down the barrel of the sensor to see if it can see at all.
- Change out the 1M Resistor for smaller values to get less sensitivity.
- Tweak the code.
Step 7: Optional - the Circuit Board
This step has a PDF you can use on a laser printer with the toner transfer or photo methods of making boards. There's lots of instructions all over for how to do this. I did determine that adding a .1uf cap across VCC and GND on the chip helps make the board work more reliably.
Print the pattern on what will be the bottom of the board. Put in the components in from the blank side of the board according to the diagram and solder them. I bent the LED and sensor leads 90 degrees so the sensor would be looking "ahead".
The PDF and layout are slightly different than the picture. I added the 0.1uf cap explicitly and made the base leg of the transistor run keep away from the sensor line.
Attachments
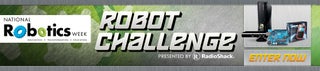
Participated in the
Robot Challenge
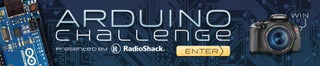
Participated in the
Arduino Challenge