Introduction: Make an Easy Button Tweet the Hard Way
- Connect it to a computer running a python script or similar.
- Connect it to a hacked router (nice - you know who you are).
- Using a third party proxy server e.g. ThingTweet, NeoCat's Arduino library, Arduino Tweet Library.
- It doesn't require an external computer/router.
- It doesn't have the security risks of method three. Passing security credentials over an unsecure transport is risky. If you are using a proxy server to generate your Tweets make sure you understand the risks.
The intention of this instructable is to give others a basic standalone framework for generating Tweets from a microcontroller.The application itself is pretty simple; press the button and it generates a semi random Tweet.
Step 1: Parts
Only a few parts are required for this project
- STM32 Discovery Board or similar
- WIZnet W5100 Network Module breakout - http://www.sparkfun.com/products/9473
- Nokia 5110 Graphic LCD breakout - http://www.sparkfun.com/products/10168
- Breadboard Power Supply 5V/3.3V - http://www.sparkfun.com/products/114
- Staples® Easy ButtonTM - http://www.staples.com/Staples-Easy-Button/product_606396?cmArea=class_box1
Step 2: Modifying the Easy Button
- Remove the four rubber feet and the four small screws under them.
- Unsolder the Vss and Vdd wires that connect to the battery terminals.
- Remove the battery terminals .
- Solder new wires to the Vss and Vdd pads.
- Solder a wire to the empty pad near the push button switch (see image).
- Drill a hole into the battery compartment and pass the three wires through it.
- Reassemble the button.
- Vss - ground
- Vdd - positive supply voltage (3V although it seems to handle 3.3V quite happily)
- Button sense - 0V rising to 3V when button is pressed.
Step 3: Hardware Schematic
The hardware is pretty basic. Time constraints have meant that it is still laid out on a breadboard but I plan to shortly make up a more permanent circuit with all the parts fitting into a small base under the button.
The power supply provides the Discovery Board with 5V and this is then stepped down to 3.3V by the onboard regulator to run all the peripherals.
The modified button is connected to PA1 of the Discovery Board and supplied with 3.3V which doesn't seem to effect its standard operation (the "that was easy" soundtrack).
The WIZnet W5100 Network module is connected to the SPI1 peripheral (pins PA5, PA6 and PA7) with additional connections for /Reset (pin PA2) and /Slave Select (pin PA4)
The Nokia 5110 Graphic LCD module is connected to the SPI2 peripheral (pins PB13 and PB15) with additional connections for /Reset (pin PB10), Data/Command (pin PB11) and /Slave Select (pin PB12).
There are a wide variety of general purpose IO pins and peripherals remaining in the Discovery Board for more advanced Twittering applications in the future.
Step 4: Workflow and Code Modules
The binary file currently stands at just under 35K in size. The code is not currently optimized so it should be possible to get the binary under 32K with a little work; of course that depends on the platform and the compiler.
The following gives an overview of the source code modules for the project. Time constraints mean the code is not as well commented as I would usually like but hopefully with the documentation in this instructable the modules should be fairly easy to follow.
Common
dictionary.c
- a useful helper class that controls a sorted linked list of name/value pairs.
sha1.c
hmacsha1.c
- Used to create a hash of the OAuth request and then encrypt it with the Consumer Secret and Access Token Secret.
- I can't take credit for these modules; they come largely unaltered from the AVR Crypto Library (http://www.das-labor.org/wiki/Crypto-avr-lib).
- Base64 encode and decode.
Drivers
easybutton.c
- Driver for the modified Staples Easy Button.
- Nokia 5100 Graphic LCD driver (SPI).
- WIZnet W5110 Network Module driver (SPI).
Protocols
Transport
udp.c
tcp.c
- The WIZnet module takes care of these two transport layer protocols so technically these also fall under the driver modules but they have been separated out for clarity.
Application
http.c
formdata.c
- HTTP client methods for making GET and POST requests to a web server.
- HTTP server methods for accepting incoming GET and POST calls.
- Get an IP address, subnet mask, gateway and DNS server IP from the network DHCP server.
- Resolve a given URL to a corresponding IP address.
- OAuth demands an accurate timestamp; this module requests the current time from a Simple Network Time Protocol server.
Web Server
webserver.c
- A listener for HTTP GET and POST requests.
- Allows for setting the OAuth Consumer Key, Consumer Secret, Access Token and Access Token Secret.
twitter.c
- Implementation of the Twitter API (currently only status update is used).
- Most of the heavy lifting is handled by the OAuth module.
- The core module of the Twitter framework.
- Generate a unique identifier for the request (nonce).
- Generate the timestamp for the request.
- Generate the signature base for the OAuth request.
- Calculate a hash of the signature base (SHA1).
- Sign the hash using the Consumer Secret and Access Token Secret (HMAC-SHA1).
- Send the signed request to the web server (HTTP GET or POST).
Attachments
Step 5: Create a New Twitter Application
- Consumer Key
- Consumer Secret
- Access Token
- Access Token Secret
The Token tells Twitter what user is Tweeting.
The Consumer Secret and Token Secret are not sent to the Twitter API but are used to calculate a cryptographic hash of the request which is used as a signature to prevent tampering. The request includes a unique identifier (nonce) and a timestamp that must be within five minute of the UTC time. Only one request will be accepted for a given nonce/timestamp pairing.
To get these key/secrets you need to create a new Twitter application.
Go to https://dev.twitter.com/apps - use your Twitter credentials to sign in.
Select "Register a new app".
Enter the application details
- Application name - anything you like
- Description - anything you like
- Application Website - (required) anything you like
- Organization - (optional) anything you like
- Application Type - Client
- Default Access Type - Read & Write
- Application Icon - use default or upload your own
Normally for applications you would request an Access Token and Access Token Secret for each user; in this case since there will only be a single user for the application there is a shortcut. From the application settings page select "My Access Token" to get an Access Token and an Access Token Secret.
Congratulations you are now ready to make your first tweet.
Step 6: Operation
- Connect the Ethernet jack to you network/router.
- Check the LCD to see what IP address has been assigned.
- In a web browser browse to the IP address (e.g. http://192.168.0.13).
- Enter the Consumer Key, Consumer Secret, Access Token and Access Token Secret you obtained and click Set.
- The LCD will now display "Ready" and the button will be armed.
- Tweet away until you are bored or your friends stop following you :)
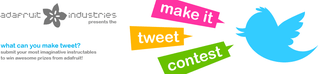
Participated in the
Adafruit Make It Tweet Challenge
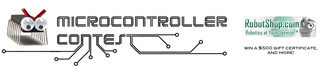
Participated in the
Microcontroller Contest