Introduction: Obstacle Avoiding Robot
I have seen many obstacle avoiding robot builds online including here at instructables, so I decided to do an instructable myself and provide as much visual and written detail as possible. Many of the obstacle avoiding robot projects that I have seen utilize a third servo upon which the PING))) sensor is mounted. This way, when the robot reaches its threshold distance to the obstacle in front of it, this third servo pans left and right then the robot turns to the route which has a “clearer” path and goes on. I on the other hand am just going to show you how to do it without the third panning servo. This is a better alternative for those on a tighter budget. Continue onto the next step to see the parts list.
Step 1: Gather the Parts
To build this robot you are going to need the following parts. All of these parts can be gotten separately or in a bundle (you won't need all of the parts in the bundle) at http://www.parallax.com/.
Hardware:
The following hardware you can get anywhere in various prices.
• Arduino Nano (any 5v Arduino of your choosing will work).
• Four AA batteries
• AA battery holder
• Breadboard (a mini breadboard was perfect in size).
• Wire
• PING))) Ultrasonic Distance Sensor
Hardware from Parallax:
The following hardware you can get anywhere in various prices.
Stock # Quantity Product Name
700-00009 1 Tail Wheel Ball
700-00022 1 Boe-Bot Aluminum Chassis
700-00023 1 Cotter Pin-1/16" Diameter
700-00025 1 Rubber Grommet-13/32" Hole Diameter
721-00001 2 Wheel, Plastic, 2.58 Dia, .3 W
721-00002 2 Rubber Band Tire for 721-00001
900-00008 2 Continuous Rotation Servo
Software:
• Arduino IDE (my version is 1.0.5).
Miscellaneous:
• Phillips head screw driver
• Computer
• Screws and nuts
Step 2: Building the Circuit
As you can see, the circuit is very simple. Just make sure that you have the signal wire of each of the servos going to the correct pins. They are not interchangeable in regards to the code that will be running the robot. The sensor that you see there in the schematic is NOT the PING))) sensor. The software that I used to create the schematic just doesn't have the PING))) sensor in its inventory, so I used the next closes one. The connections are the same (red=power, black=ground, yellow=SIG). I believe the circuit is self-explanatory, but if you have any questions, don't hesitate to ask. Continue onto the next step for the programming part.
Step 3: Programming
The basic concept of the code is for the robot to always be monitoring for objects in front of it while moving. This is done by the PING))) sensor where it sends out a 40kHz chirp which is not audible, and retrieves the echo. The program then gets the duration it takes for the pulse to get back and converts it into distance. Once the robot detects an object where the distance forward is greater than the danger threshold - the path is clear so our program tells the robot to move forward. Otherwise the robot will turn and proceed. The code just loops in this fashion.
THE CODE:
/*
> Design an obstacle avoiding robot using an Arduino NANO and Parallax PING))) Ultrasonic Distance Sensor
> Zoran M.
*/
#include //include Servo library
const int RForward = 0;
const int RBackward = 100;
const int LForward = RBackward;
const int LBackward = RForward;
const int pingPin = 7;
const int dangerThresh = 15; //threshold for obstacles (in cm)
Servo leftMotor;
Servo rightMotor; //declare motors
long duration; //time it takes to recieve PING))) signal
void setup()
{
rightMotor.attach(11);
leftMotor.attach(10);
}
void loop()
{
int distanceFwd = ping();
if (distanceFwd>dangerThresh) //if path is clear
{
leftMotor.write(LForward);
rightMotor.write(RForward); //move forward
}
else //if path is blocked
{
leftMotor.write(LBackward);
rightMotor.write(RForward);
delay(1000);
}
}
long ping()
{
// Send out PING))) signal pulse
pinMode(pingPin, OUTPUT);
digitalWrite(pingPin, LOW);
delayMicroseconds(2);
digitalWrite(pingPin, HIGH);
delayMicroseconds(5);
digitalWrite(pingPin, LOW);
//Get duration it takes to receive echo
pinMode(pingPin, INPUT);
duration = pulseIn(pingPin, HIGH);
//Convert duration into distance
return duration / 29 / 2;
}
Continue onto the last step for additional pictures and conclusion.
Step 4: Additional Pictures and Conclusion
Everything went pretty well while putting this project together. The program had no trouble whatsoever; the only thing I had some trouble with was the PING))) sensor. As I declared the threshold to be 15 cm it was picking up distances sometimes further than what I set it to be and sometimes closer than what I set it to be. A suggestion for future improvement would be to have a random method in the program where when the robot approached an object I would have no control whether it would turn left or right and by how many degrees. With this random method, both the the direction and degree of turn would be totally random; making it easier for the robot to get out of corners. Don't hesitate to post up your versions, even if you did it like I did. I would love to see them!
01001000 01100001 01110110 01100101 00100000 01100110 01110101 01101110 00100001
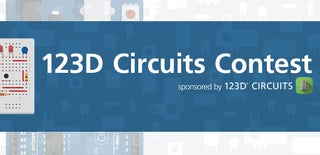
Participated in the
123D Circuits Contest
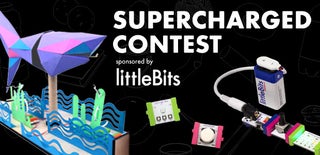
Participated in the
Supercharged Contest