Introduction: Raspberry Pi Lightshow With Blynk Control
I was searching through the net for some DIY light decoration project ideas. I came across with Lightshow Pi and decided to build my own version of light show with 8 AC Outputs, adding some features to make the whole system more user friendly for my parents to use at home.
The whole system summed up in few words, a light show control box that runs light show in two mode: a normal sequence and music mode. The user control is done using Blynk App on smart devices. The music will be streamed via FM transmitter to house or car music system (I drew this idea from another LightshowPi project). You can also use a direct speaker instead of an FM transmitter.
Step 1: List of Materials
Raspberry Pi ( I used Model B which I bought back in 2013)
8GB SD/MicroSD card (Depends which model Pi you are using)
Wifi Dongle (Not Needed if you using Raspberry Pi 3)
8 Channels Solid State Relay (Model)
Extension Cord 4 outlets
8 Electrical Socket Outlets
3 core Electrical Power Cable
10 Female-Female Jumper Wires
Panel Box (I used a security alarm panel box here)
Car 3.5mm FM Transmitter (Model)
USB Outlet Power Adaptor
Wire connectors
As for the box internal wiring, mine is almost similar with this Instructable project. You can refer to the link there for instructions on how to wire the relay and power outlets for the lights.
Step 2: Raspberry Pi Preparation
Well I assume you have some basic knowledge on setting up a Raspberry Pi. To newbies, there are plenty of resources can be found online. Few things to take note for this project:-
- Get your Pi a static IP address
- Make sure Internet is working on the Pi as it needs to be online for Blynk to work
- Set up FTP Transfer via File Zilla
- If you are using the 1st generation of Pi Model A or B, I suggest you overclock it as you may experience audio laggy issues when running lightshowpi.
Download this latest Raspbian Jessie here. Burn the image in your SD/microSD Card.
I build this project on Raspbian Wheezy. I have a copy of the image here that you can use. If you choose to use this image, straight proceed to Step 6. The default IP for the Pi for this image is 192.168.1.107
Step 3: Lightshowpi Installation
I am not gonna elaborate much on this as there are plenty of projects already available out there explaining this. You can check their website here for the installation steps.
Step 4: Default Sequence Script
I have here wrote a simple python code that runs the lights in default pattern at boot. Of course you can modify the pattern as you like it to be.
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BOARD)
GPIO.setwarnings(False)
GPIO.setup(7,GPIO.OUT)
GPIO.setup(11,GPIO.OUT)
GPIO.setup(13,GPIO.OUT)
GPIO.setup(15,GPIO.OUT)
GPIO.setup(12,GPIO.OUT)
GPIO.setup(16,GPIO.OUT)
GPIO.setup(18,GPIO.OUT)
GPIO.setup(22,GPIO.OUT)
while True:
GPIO.output(7,GPIO.HIGH)
GPIO.output(11,GPIO.LOW)
GPIO.output(13,GPIO.LOW)
GPIO.output(15,GPIO.HIGH)
GPIO.output(12,GPIO.HIGH)
GPIO.output(16,GPIO.HIGH)
GPIO.output(18,GPIO.HIGH)
GPIO.output(22,GPIO.LOW)
time.sleep(1)
GPIO.output(7,GPIO.LOW)
GPIO.output(11,GPIO.HIGH)
GPIO.output(13,GPIO.HIGH)
GPIO.output(15,GPIO.LOW)
GPIO.output(12,GPIO.LOW)
GPIO.output(16,GPIO.LOW)
GPIO.output(18,GPIO.LOW)
GPIO.output(22,GPIO.HIGH)
time.sleep(1)
GPIO.output(7,GPIO.HIGH)
GPIO.output(11,GPIO.LOW)
GPIO.output(13,GPIO.LOW)
GPIO.output(15,GPIO.LOW)
GPIO.output(12,GPIO.HIGH)
GPIO.output(16,GPIO.LOW)
GPIO.output(18,GPIO.HIGH)
GPIO.output(22,GPIO.LOW)
time.sleep(1)
GPIO.output(7,GPIO.HIGH)
GPIO.output(11,GPIO.HIGH)
GPIO.output(13,GPIO.HIGH)
GPIO.output(15,GPIO.HIGH)
GPIO.output(12,GPIO.HIGH)
GPIO.output(16,GPIO.HIGH)
GPIO.output(18,GPIO.HIGH)
GPIO.output(22,GPIO.HIGH)
time.sleep(5)
Upload the code in /home/pi folder.
Attachments
Step 5: Blynk Installation on Pi
To get Blynk on Pi, you need to make sure wiringPi is installed first.
To install wiringPi, run the following command in Terminal :-
git clone git://git.drogon.net/wiringPi
Switch to the resulting wiringPi directory and run the build script:
cd wiringPi
./build
To make sure the library has been installed, run the gpio -v command, which should return the version information. To compile the Blynk library, use the following commands:
git clone https://github.com/blynkkk/blynk-library.git
cd blynk-library/linux
make clean all target=raspberry
Step 6: Blynk Configuration on Phone
Step 7: Blynk Configuration on Pi
1.Download this file. Edit the file by replacing the token with the token generated from the project you created in your Blynk app as below. Upload it to /home/pi folder.
/home/pi//Blynk/Blynk/linux/./blynk --token=<Your token here>
2. On Terminal, type "sudo nano /etc/rc.local" and add "/home/pi/autostartblynk.sh" before "exit 0" line.
3. On Terminal, type "cd /home/pi", press ENTER and followed by typing "chmod +x autostartblynk.sh" and press ENTER again.
4. Reboot Pi. Blynk shall autostart at boot.
Step 8: Upload Music
Upload your mp3s and the playlist file here to /home/pi/lightshowpi/music folder. I have created the playlist file for 15 mp3 songs. All you have to do is rename your MP3 from 1.mp3 to 15.mp3 and rename the playlist file to ".playlist".
Step 9: Play Music
The "start_music_and_lights" command to run all songs in playlist in sequence as per in lightshowpi official website (link) didn't work for me. So I wrote another python script to continuously play songs from my playlist as below:-
import time
import os
while True:
os.system("sudo python /home/pi/lightshowpi/py/synchronized_lights.py --playlist=/home/pi/lightshowpi/music/.playlist")
time.sleep(1)
os.system("sudo python /home/pi/lightshowpi/py/synchronized_lights.py --playlist=/home/pi/lightshowpi/music/.playlist")
time.sleep(1)
Upload this code musicloop.py in /home/pi folder.
*You can skip this step if you used my pre-configured image.
Attachments
Step 10: Blynk Control Script
I have prepared a python script which when executed, can start/stop the default/music mode and also to shutdown and restart the pi via Blynk app. You can also use physical buttons to the respective GPIO pins with this code to control the system.
import RPi.GPIO as GPIO
import time
import os
GPIO.setmode(GPIO.BCM)
GPIO.setup(7, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
GPIO.setup(8, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
GPIO.setup(9, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
GPIO.setup(10, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
GPIO.setup(11, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
GPIO.setup(15, GPIO.IN, pull_up_down = GPIO.PUD_DOWN)
def DefaultSequence(channel):
os.system("sudo python DefaultSequence.py")
def StopDefaultSequence(channel):
os.system("""sudo ps aux | grep "python All_Lights.py" | grep -v grep | awk '{print $2}' | xargs sudo kill -9""")
def MusicON(channel):
os.system("sudo python musicloop.py")
def MusicOFF(channel):
os.system("""sudo ps aux | grep "python /home/pi/lightshowpi/py/synchronized_lights.py" | grep -v grep | awk '{print $2}' | xargs sudo kill -9 && sudo ps aux | grep "python musicloop.py" | grep -v grep | awk '{print $2}' | xargs sudo kill -9 """)
def Reboot(channel):
os.system("sudo reboot")
def Shutdown(channel):
os.system("sudo shutdown now")
GPIO.add_event_detect(7, GPIO.FALLING, callback = MusicOFF, bouncetime = 2000) GPIO.add_event_detect(8, GPIO.FALLING, callback = Shutdown, bouncetime = 2000) GPIO.add_event_detect(9, GPIO.FALLING, callback = Reboot, bouncetime = 2000)
GPIO.add_event_detect(10, GPIO.FALLING, callback = DefaultSequence, bouncetime = 2000) GPIO.add_event_detect(11, GPIO.FALLING, callback = MusicON, bouncetime = 2000) GPIO.add_event_detect(15, GPIO.FALLING, callback = StopDefaultSequence, bouncetime = 2000)
while 1:
time.sleep(1)
Upload this code in /home/pi folder.
*You can skip this step if you used my pre-configured image.
Attachments
Step 11: Autorun All Scripts at Boot.
Upload these files to /home/pi/.config/autostart folder and reboot Pi. All the python scripts shall start at boot post rebooting.
*You can skip this step if you using my pre-configured image.
Step 12: Run
At boot, the system will run the default sequence. To switch to music mode, tap STOP DEFAULT SEQUENCE and then tap PLAY MUSIC. To switch back to default mode, tap STOP MUSIC and tap DEFAULT SEQUENCE.
Tap SHUTDOWN to shutdown the pi everytime before powering off.
Step 13: Extra Note: Light Controller Bypass
If you are using the typical flashing lights with 8 sequence like I did, best is to override the controller to stop flashing in order to achieve perfect sync with the music. I followed the guide here to override that.
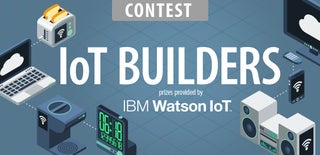
Participated in the
IoT Builders Contest
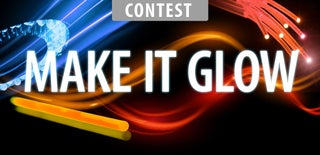
Participated in the
Make it Glow Contest 2016