Introduction: Raspberry Pi, Android, IoT, and Bluetooth Powered Drone
Using a Raspberry Pi for the on-board logic, this compact, mobile computer, will create a local port that streams a video in real-time while simultaneously creating Bluetooth sockets to read values sent by a custom android app. The app syncs with the drone and uses user input to send instructions to the drone instantly.
This project is fairly difficult to make from scratch. Hopefully, the following information will provide insight for multiple drone applications. All the software and circuit designs pertaining to this project is provided. Feel free to modify and share the code to your liking. Please use the information provided responsibly and feel free to leave a comment below.
Step 1: The Hardware
Below is the list of hardware that I used.
- Raspberry Pi 3 Model B
- Raspberry PI Camera Module
- L298N Motor Driver
- 2, DC Motors
- A Keyboard, Any Monitor, Mouse, Ethernet Cable, and HDMI Cable (for the pi)
- 8GB MicroSD Card
- Screws, Tape, ect.
- 2 Wheels
- An Android Bluetooth Device (My phone)
- 2, 18650 Cells
- A 5 Volt Regulator
- A computer to program the app on
A Raspberry Pi: For higher stream resolutions (or for beginners), I would recommend the newest version of the B model while more compact versions may use either the newest Model A+ or the Pi Zero (Camera Version). Remember that if you use a raspberry pi zero, you will need a Bluetooth and/or WiFi dongle for it. This tutorial will assume your using a Raspberry Pi 3 B.
A motor Driver: I used a L298N, although any motor driver should work. Just ensure that it can handle your motors voltage and current.
2 DC Motors: Make sure they can handle the weight of your drone.
A body: Used too hold all the components in. Usually hard plastic or aluminum is a durable, lightweight material to use. For optimal results, even a 3D Printer can be used.
A Power Source: Its almost always the toughest decision when choosing parts. The battery required depends on current draw. For low powered applications (like 1 or 2 amps), a 9v battery should suffice. For higher amperage, I recommend either a 18650 Lithium Ion cell or a Lithium Polymer battery since they are lightweight, can hold a lot of power for their size, and are rechargeable.
2 Wheels: Just ensure that your Wheels are the have the same hole size as the shaft of your motor. Also ensure that the diameters of the Wheels are big enough for your drone. Since their are multiple possible variations and designs for this project, their are multiple different wheel types and sizes to use.
Voltage regulators: A 5 volt regulator is required to power the pi via a battery. I used a LM2596 DC-DC buck converter.
Most of the products in the links were used in this project and were the best deals I could find at the time.
Now that you have everything you need, lets setup the pi.
Step 2: Setting Up the Raspberry Pi
This step will show you how to setup the Raspberry Pi so you can start coding. If you already have a pi setup with Raspbian, than feel free to move onto the next step.
First, you need to download an operating system for the raspberry. Get NOOBS if your a beginner. If you already have notable experience with a raspberry pi, then you may be interested in Raspbian. I will assume that you are using NOOBS for this tutorial.
While thats downloading, Format your SD card with SD Formatter.
Now Extract and copy the contents of the download into the root of your SD card. Root simply means that its not inside any folders. If a new folder was created to hold all the extracted files, copy across that file.
Next attach the SD card to the pi. While inserting, it should either "click" in or just sit inside the bottom of the pi.
Plug in your mouse and keyboard into the pi. Then plug in a HDMI cable to the pi from a monitor. Finally plug in a 3 amp micro USB wall socket adapter into the pi. Although a wall socket is recommended, I used my laptop as a power source (USB from my laptop to Micro USB in the pi).
Let the pi do its thing. If prompted, select Raspbian and let it install. Follow the instructions in the installer. It may take a while to complete. If everything goes right, you should see a desktop screen similar to the one above.
Plug in an Ethernet cable from your router into the pi. Then open the command line (The black "box" icon in the top of the screen). You will need to update the pi through a wired network to connect it to a wireless network.
Then click on the Cable icon on the top of the screen. Type in your network details where prompted.
Type the text in the next line exactly as shown and hit enter. This will update the pi. It may take awhile. Just let it do its thing.
sudo apt-get update
Then type in the code in the next line. This will also take some time. Dont do anything until its finished.
sudo apt-get dist-upgrade
Now you should be able to disconnect the Ethernet cable, click on the wireless icon at the top of the screen, enter your networks information, and finally connect to your network via wireless.
Now lets code the part of the drone that handles movements.
Step 3: Handling Movements (Bluetooth Server)
For handling movements, the programming language "Python 3" is used. The messages that tell the robot to move will be sent via Bluetooth from the app.
First, you need to install the Bluetooth library. Type in the following commands to do so.
sudo apt-get update
sudo apt-get install bluetooth
sudo apt-get install bluez
sudo apt-get install python-bluez
Now from the desktop, click on the start menu icon, then Programming, then Python 3. Then from the toolbar, click File, new. A new window should appear.
Copy the contents of the attachment "bluetoothpi.rtf" into the window.
On the toolbar, click on File, Save as. Save it in the /home/pi/Desktop/ directory as movements.py.
Now to test the code, click on the Bluetooth icon on the top-right corner of the screen. Pair the raspberry pi with your android device. Download an app called BlueTerm on your android device. Then in the command line, type in the following code in bold. This will execute the python code.
sudo python /home/pi/Desktop/movements.py
The text "Waiting for connections" should appear on the pi.
Start BlueTerm and click on the options icon and then the "look for connections" button.
On the app, your raspberry pi's name should appear. Click on the button with the pi's name. The text "Accepted connection from" should appear on the pi followed by its address. Now whatever you type in the phone, should be displayed in the pi.
You have successfully coded your own Bluetooth server socket!
Attachments
Step 4: The Android App (Bluetooth Client)
Download Android Studio here. Install it and follow the instructions given in the installer.
Start a new project. Create a blank activity called MainActivity.
Copy the contents of the attachment "Logic.txt" in the "MainActivity.java" file (tab). This contains all the logic behind the app. You may need to change the name of your device in the bottom of the file.
Then copy the contents of the file "GUI" in the "activity_main.xml" file (tab). This contains a very simple GUI for the app.
Now you will need to import the arrow buttons (pictures) into the app. Unzip the attachement Arrows.zip. On the left of android studio, open the file structure to see app, res, minimap. Copy the arrow pictures (PNG files), right click on the file minimap and paste the pictures into the file, keeping the names of the arrows the same. It should look like the picture when finished.
Finally copy the contents of the file "Manifest" to your "AndroidManifest.xml" file (tab).
To test the app, you will need to run it on your device. To do this, you will need to set the device to developer mode and enable USB debugging. For most devices, you will need to go to "Settings", "About Phone", scroll to the bottom and click on "Build Number" seven times until you see the message "You are now a developer!" Go back and you should now see a "Developer options" tab. Click on it and enable USB debugging.
Connect your android device to your computer via USB, click on the run icon on Android Studio, and select your device.
On the pi, start the python code created in step 3 by typing in the command in bold:
sudo python /home/pi/Desktop/movements.py.
Then click on the connect button in the app. When connected, some arrows should now appear on the app. Whenever you click on one, it will update the pi's "move" state.
Its alright if the background of the app gives an error. This will be fixed later.
You have just created you own app and Bluetooth Client.
Step 5: Connecting the Hardware
Solder the connections in the diagram above. Attach the camera module to the pi too.
Run the app and execute the the movements.py file. If the motors move correctly when using the app, feel free to put all the components into a finalized shell for the drone. You may need to modify the codes "HIGH" and "LOW" values so it moves correctly.
In the next step, we will add the streaming feature of the drone.
Step 6: Streaming
Their are multiple ways to stream a video using a Pi, but using uv4l is by far the easiest way with virtually no lag.
Just a side note, if you have already put your drone together and cant connect it to your monitor and keyboard, you will need to SSH into your drone. To do this, download Putty on your computer. Open it and type in your raspberry's IP address (find the IP address by typing the command ifconfig). It will prompt you for a username and password. The default username and password is pi and raspberry respectively. Now whatever you type in the command box will be like entering commands directly into the drone.
setting up uv4l
Type in this command:
sudo nano /etc/apt/sources.list
Add the following line in the in the last line of the file.
deb http://www.linux-projects.org/listing/uv4l_repo/raspbian/ jessie main
Exit and save the file by pressing Ctrl-X and then typing Yes.
Then type the following line and press enter.
sudo apt-get update
then this:
sudo apt-get install uv4l uv4l-raspicam
Type in the following commands line by line. Wait for it to finish what its doing before typing in the next line.
sudo apt-get install uv4l-raspicam-extras
sudo service uv4l_raspicam restart
sudo rpi-update
sudo apt-get install uv4l-server uv4l-uvc uv4l-xscreen uv4l-mjpegstream uv4l-dummy uv4l-raspidisp
sudo apt-get install uv4l-webrtc
sudo apt-get install uv4l-xmpp-bridge
sudo apt-get install uv4l-raspidisp-extras
After that, all you have to do is type in the following command to start streaming (replace "raspberrypi" with the raspberry's IP address/ Host name in your network)
cvlc http://raspberrypi:8080/stream/video.mjpeg
This will start streaming video in real time on port 8080. The stream should be seen in the background of your app now. To view the stream in any browser, type in this URL (where "raspberrypi" is your drones IP address).
http://raspberrypi:8080/stream/video.mjpeg
Congrats, you have completed your spy drone.
Step 7: Drive!
Flip the power switch and start exploring.
If you want to know how to make something else, ask me and I'll see what I can do.
If you have any questions, please comment below and I'll do my best to answer them.
Thanks for reading!
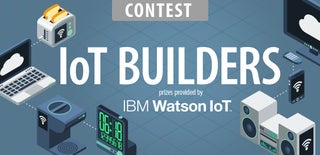
Participated in the
IoT Builders Contest