Introduction: Robot "Cheaper"
The aim is to build cheapest possible Arduino-robot
Step 1: Components
- Arduino UNO R3 x1
- USB cable x1
- Mini solderless breadboard x1
- IR sensor x1
- Continuous rotation servo x2
- Battery holder x1
- AA battery x4
- Bunch of color wires
Step 2: Getting Started With Arduino IDE
First of all we need to install Arduino IDE. The Getting Started Guide from the official Arduino website will tell you everything about it. Once you have installed Arduino IDE you can start writing your own programs for Arduino (often called sketches). We'll use a language based on C to program our Arduino.
Let's start.
- Open Arduino IDE. By default it will show an empty sketch.
- Open example sketch via *File → Examples → 01.Basics → Blink* (or see [Appendix 1](#appendix-1-led-blinking-example)).
- Compile this example (*Sketch → Verify/Compile*). You'll see "Done compiling" and the size of the binary in bytes when the compilation is completed.
- Connect your Arduino board to your laptop using a USB cable. After that you will see some connected device in *Tools → Serial Port*. Select it. Now you are able to upload your sketch to the Arduino board.
- To start uploading simply press the Upload button on the toolbar or choose *File → Upload*. Arduino will start blinking its TX/RX LEDs and after a few seconds your sketch will have finished uploading indicated by "Done uploading" and the LEDs not flashing anymore.
Congratulations! You have just uploaded your program to the microcontroller.
Step 3: Connect IR Sensor
There is a wide range of different sensors that can be used with Arduino.
We will use a Sharp infrared distance sensor (GP2Y0A21YK0F) to measure distances of objects to your robot. Its range is restricted from 10cm to 80cm.
It's easy to connect this sensor to Arduino. Take a look at the wiring diagram.
Connect wires as shown: * + (red wire) to +5V pin * ground (black wire) to one of the GND pins * signal (yellow wire) to pin A0
We will use following sketch to calibrate your IR distance sensor (see attachment).
The sketch converts the voltage values read from the first analog pin (A0) to distances in centimeters. To achieve that, it uses a prefilled table (2-dimensional array) that maps the measured value to its corresponding length in centimeters. It's important to note that Arduino uses a 10-bit analog to digital converter. This means that the value read from the analog pins (0V to 5V) will be translated into integer values between 0 and 210 - 1 = 1023. If you open *Tools → Serial Monitor* after compiling and uploading the sketch, you will see the current analog value and the corresponding distance to the object in front of the sensor.
You can check the measurement by placing an object aligned to a ruler and matching the output from the Serial Monitor. If it's not enough precise, you should tune the values in the table until you reach the level of precision you need.
Congratulations! You just learned how to read the output of an IR distance sensor!
Attachments
Step 4: Connect Servo Motors
There are a wide range mechanisms that can help our robot to move. The most commonly used are DC motors, stepper motors and servos. You will use continuous rotation servos for that.
Servo motors typically have three wires: power (red), ground (black or brown) and signal (yellow, orange or white). Connect them as follows:
- power to the +5V pin on the Arduino board
- ground to a GND pin on the Arduino board
- signal to a digital pin on the Arduino board
If you want to learn how the work, you may be interested in this article.
At first you will calibrate the servos. Calibration can be performed in software and hardware. In the first case you'll have to do adjustments with the potentiometer. In the latter case you want to adjust pulse by changing the parameters passed to the `writeMilliseconds` function. Later you will control two servos, so we suggest that you calibrate the potentiometer to match the software, in order to have the same base value for both motors.
The following sketch will help us calibrating our servos. Change it to run the servo on zero speed and adjust the potentiometer position to ensure that at zero speed there's really no rotation.
Then you will connect two servos at once to control them simultaneously. Take a look at the wiring diagram.
You need to slightly change the sketch to control both servos simultaneously. Check out sketch for the complete code.
Attachments
Step 5: Power It Up
Until now we used power from USB. In order to let your robot drive around freely you will need some portable source of energy: Four AA batteries will be used for each robot.
Plug the positive (red) cable into VIN, and the negative (black) cable into one of the GND pins of your Arduino board. The Arduino board has a built-in voltage regulator and may work with 5V to 20V on VIN (though 7V through 12V are recommended).
Connect everything as shown in the wiring diagram.
Make sure you connect everything properly and then turn the battery switch to ON.
Note that servos consume a considerable amount of power, so if you need to drive more than one or two, you'll probably need to power them from a separate supply (not the +5V pin on your Arduino). Be sure to connect the grounds of the Arduino and the external power supply.
Step 6: Robot "body"
We'll have to prepare several partsto build your Robot's "body":
- Main parts of construction (cut from wood or plexiglass)
- Screws and nuts to fix servos, IR sensor and wheels (you'll probably need some to be bought in local store)
- Wheels (printed on 3D printer)
- Tires (rubber rings)
You can take models from attached files or repository and get them done in the nearest hacker space.
Then you'll need to glue all parts of robot together and make necessary holes.
As soon as all preparements are done fix servos to the side panels with screws (12mm M2). Pay attention that the servo shaft should align closer to the backside of the robot body. Then fix the IR sensor on the front panel also with screws (6mm M3). Then pull all the wires through holes in front and back panels so that all of them come out on the back. Then take mini breadboard and stick it with 2-sided adhesive tape on back panel.
Use three screws (12mm M2) to mount the Arduino board on top of the body. You can put small piece of material between panel and board to avoid contact between their surfaces (or just to fit it better). Small plastic spacers are also an option.
Fix together wheel and round fasteners with screws. Then put rubber ring on the wheel. Put wheel on the servo shaft and fix with screw.
Congratulations! It is almost done!
Step 7: Program Robot
In our first implementation we will try to achieve following:
- avoid obstacles
- avoid table edges
You are restricted to use only one sensor.
In the first picture you can see that in normal state when the robot moves along the surface, the distance to the nearest obstacle is equal to the distance of the surface itself.
If our robot will reach some object lying on the surface, the distance is much less than the default distance to the surface (Lmax).
So the easiest way to reach our first goal is to keep our robot moving within this distance range.
Similarly you can reach the second goal - avoid table edges. If the distance is more than Lmax it is more likely that the robot is directing to the table edge.
Let's define two basic rules:
- when distance to obstacle (D) within range Lmin and Lmax robot will move straight forward
- when D is greater than Lmax or less than Lmin robot will rotate until it finds direction with D lying within safe range
The robot has 2 wheels. It will drive under the most basic algorithm for a robot - differential drive:
- to drive straight both wheels move forward at equal speed
- to drive reverse both wheels move back at equal speed
- to turn left the left wheel moves in reverse and the right wheel moves forward
- to turn right the right wheel moves in reverse and the left wheel moves forward
In your case servos are placed in opposite directions so that for one of them robot forward moving means clockwise rotation, for another one - counter-clockwise. Try to keep this in mind.
Take a look at the example sketch, try it and start implementing custom behaviour!
Attachments
Step 8: Links
Following materials pushed me for writing this tutorial:
- SumoBot Kit
- How to Build Your First Robot Tutorial
- How to use sharp IR sensor with arduino
- Infrared IR ranger comparison
- Sharp GP2Y0A21YK datasheet
- Parallax Continuous Rotation Servo
- Pulse WIdth Modulation
- Operating Two Servos with the Arduino
- Arduino’s Servo Library: Angles, Microseconds, and “Optional” Command Parameters
- Powering Arduino with a Battery
- PID controller
- Sumo Rules
- Line Follower Rules
- Mini Sumo Robot with Proximity Sensors
- Unified Sumo Robot Rules
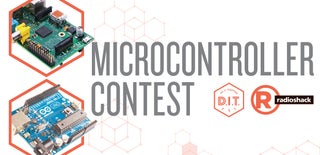
Participated in the
Microcontroller Contest
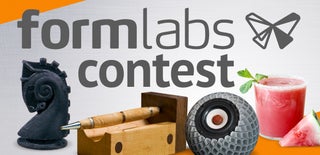
Participated in the
Formlabs Contest
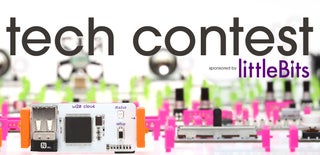
Participated in the
Tech Contest