Introduction: Simple Humanoid Walking and Dancing Robot (Arduino)
This instructable will be about making a humanoid walking robot that uses Arduino and can walk, jump and do some dances. The thing that is different in this robot compared to other humanoid robots is that while others use several high torque (expensive) servos, this robot only uses 2 (micro) servos per leg. This makes the robot easy to create, code and also cheap to make. This robot can be made as a beginners robot to introduce yourself to the field of robotics. Let's get into making the robot!!
Step 1: Tools and Material Required
The bill of materials is as follows:
- Micro Servo or any other (qty. 4) ($6 for four).
- Arduino UNO(you can use other models but keep in mind that using other models may affect stability). ($6.45)
- Wires. ($1.6 for 1m)
- Perfboard(not necessary, I did not use one). ($0.78 for one, sold as kit)
Thin sheet wood (2 pieces measuring 6.2 x 4.6 cm). This measurement can vary if you are using any other type of servos.
Cardboard (small pieces required).
Total:Around $16 including cardboard and sheet wood.
The required tools include:
- Soldering Iron (with solder).
- Hot Glue gun.
- Cutter.
- Hacksaw (to cut wood).
- Printer cable for Arduino.
Step 2: Preparing Servos
The first thing that you need to do is to attach the servo horns to the servos. Keep in mind that you can't just attach them to the servo, it has to be done properly or you will encounter problems later.This video explains how to attach the servo horns to the servos, click here to view it. (The video is not mine but is made by Karl Wendt).
Now that you have attached the horns to the servos, it is time to glue the servos together. Both the servo sets are attached in different ways so be careful while gluing them. I have attached pictures which you can use as reference to attach the servos together. Try to keep the horns are as close to 90 degrees as possible.
After that, take a piece of cardboard and glue both of the servos on it and cut extra cardboard out. One way to ensure that you do this properly is to make sure that the screw mounting part of both the servos is touching. Make sure to keep the wire part on the outside.
Step 3: Attaching the Arduino
Once that is done, take a rectangular piece of cardboard (mine measured 6.4 x 5.4 cm) and hot glue it to the backside of the servos as shown in the pictures. You can round the corners to make it look a bit neater.
After that, take the Arduino and hot glue the backside of the arduino (the white side) to the cardboard as shown in the pictures. This MAY damage your Arduino (highly unlikely) so if you do this, it will be at your own risk.
The only thing left to do now (in terms of hardware) is the wiring.
Step 4: Wiring the Servos!
The way I have done it, it looks a bit confusing but believe me, it isn't. The red wire of the servos are positive, the brown are negative and the orange wire is the signal wire. So, all you have to do is to solder all the red wires together and then solder a stiff piece of wire to that which connects to the 5v pin of the Arduino. Then, solder all the brown wires together and attach a solid piece of wire to them. This wire will connect to the GND pin which is below the 5v pin. (Wiring diagram attached)
After that, you will be left with four orange wires or signal wires. Before soldering them to a stiff wire for connection, you have to understand the naming for the servos. Looking from the pin side of the arduino, the servo on the top right is the right thigh servo. The one below it is the right foot servo. The servo at the top left is the left thigh servo and the one below it is the left foot servo. Connect the right thigh signal wire to pin 5, the left thigh signal wire to pin 11, the left foot signal wire to pin 3 and the right foot signal wire to pin 9. Make sure that all of the signal wires are attached to the pins with a squiggly line before them (PWM pins). (Wiring diagram attached)
Step 5: Choosing Power Source
Now comes the time to choose whether you will be powering the robot using USB power (which is inconvenient and causes occasional disturbances) or using six AA cells. I chose the latter, although it's entirely your choice what you want to do. The problem with AA cells is that they run out in a few hours. If you chose USB power, all you have to do is to connect a printer cable and power it with that (mine didn't work like that, it kept on falling), but if you chose AA cells then its a little bit more complicated. If you are using USB power (which I don't recommend), you can a power bank to make it more portable.
First of all, you have to create the 9v power supply which is just 3 3v AA battery packs soldered to each other in series. Then, you have to wire the 9v pack to the power jack on the Arduino (the wiring diagram is attached). The power jack has 3 pins one on top, one on bottom and one on the right (pic attached). The one at the top and the one at the right are the ground pins. The negative wire of your battery pack connects to one of them. The one at the bottom is the 9v pin, the positive wire of your battery pack gets soldered to that.
Once again, soldering wires directly to that is can damage your Arduino (although its unlikely), so do this at your own risk.
Step 6: Adding Feet
The next step is creating the robot is to attach feet to the base of the robot. For feet, I used a 6.2 x 4.6 cm piece of thin sheet wood for the feet. At first, I had used cardboard but that was very floppy and unsuitable so I opted for sheet wood. All you have to do now is to hot glue the wood onto the feet servos of the robot. Try to glue the servos at the exact middle of the feet.
Step 7: Programming
Now that we are done with the hardware part of the robot, it is time to work on the software. To upload the code to the robot, you need to download the Arduino IDE software. It can be downloaded from here. Once that is done installing, connect the Arduino to your PC via the USB printer cable. Then open the Arduino software and go to the 'Tools' from the toolbar and it should say Arduino/Genuino there. Click on ports and select the one with Arduino/Genuino next to it. Then, enter the code I have provided into the software and click on the right arrow sign at the top left. After some time, it should say Done Uploading and if you have done everything right, your robot should start to move!! If you are having any problems, mention them in the comments section and I will try to answer them ASAP. I have uploaded the code for walking, jumping and a few dances. Of course, once you are familiar with the software you can write your own code for the robot. If you made some mistakes while making the robot, you might have to tweak the code just a little bit. Once again, if you need help just ask for it in the comment section. (Note: Yes I know, the way I programmed this robot is very inefficient but this was my first micro-controller project and I did not know how to use functions so hopefully the coding will be better in the future contests!)
Step 8: Understanding the Code
In this step, I will try to explain the code the best I can. Lets do the dancing code.
-----------------------------------------------------------------------------------------------------------------------------------------------------
#include <><> This command is used for including the servo library.
Servo rightfoot; <><> This command creates a Servo object with the name 'rightfoot'. This will be used to address the servo later.
Servo rightthigh;<><> This command creates a Servo object with the name 'rightthigh'. This will be used to address the servo later.
Servo leftfoot;<><> This command creates a Servo object with the name 'leftfoot'. This will be used to address the servo later.
Servo leftthigh;<><> This command creates a Servo object with the name 'leftthigh'. This will be used to address the servo later.
void setup() <><> This includes the intial commands that the Arduino will go through once before going through the loops.
{ <><> Marks the beginning of the setup commands.
rightfoot.attach(9); <><> This command attached the Servo object 'rightfoot' to pin 9.
rightthigh.attach(5); <><> This command attached the Servo object 'rightthigh' to pin 9.
leftfoot.attach(3); <><> This command attached the Servo object 'leftfoot' to pin 9.
leftthigh.attach(11); <><> This command attached the Servo object 'leftthigh' to pin 9.
} <><> Marks the ending of the setup commands.
void loop() <><> This is the code that will be repeated multiple times to do the actions.
{ <><> Marks the beginning of the loop of commands.
leftfoot.write(10); <><> This command is used to make the Servo go to it's initial position after each loop.
leftthigh.write(90); <><> This command is used to make the Servo go to it's initial position after each loop.
rightthigh.write(105); <><> This command is used to make the Servo go to it's initial position after each loop.
rightfoot.write(180); <><> This command is used to make the Servo go to it's initial position after each loop.
delay(1000); <><> This creates a 1 sec delay before the next command is executed. The value is in milliseconds.
leftfoot.write(17); <><> This command is used to make the 'leftfoot' servo go to 17 degrees.
delay(25); <><> This command creates a delay of 25 ms before the next command is executed. It is added to make the actions look smoother. If you want to make the dance slower, you can increase the value of all the delay commands and vice versa.
leftthigh.write(95); <><> This command is used to make the 'leftfoot' servo go to 17 degrees.
delay(25);<><> This command creates a delay of 25 ms before the next command is executed. It is added to make the actions look smoother. If you want to make the dance slower, you can increase the value of all the delay commands and vice versa.
......... <><> I did not write all the commands because all the commands that follow are basically the same as these.
.........<><> I did not write all the commands because all the commands that follow are basically the same as these.
} <><> Marks the ending of the loop of commands.
(I have attached a picture which shows how the servo degrees work.)
-----------------------------------------------------------------------------------------------------------------------------------------------------
I used more or less the same commands in all of the programs I have coded so I don't think further explanation is needed. This could have been done in a much more shorter and efficient way but this was my first time working with a micro-controller so I used basic commands as they seemed to get the job done :). If you need further explanation of any part of the code, feel free to ask in the comments.
Step 9: Conclusion
Now that the robot is done, what next? Well, you should now be familiar with robotics and also with writing code for the Arduino. You can continue to code new functions for this robot or even make a new and bigger robot. This instructable was created with mainly the purpose of introducing people to the field of micro-controllers and robotics. I think that this is an excellent beginners robot as it is easy and cheap to make. I originally got this idea from this instructable here, but the person who made it made a mistake or something, I'm not sure, because my robot as well as many other people's robot in the comments section was not working so I though that I would re-write my own version of that instructable. Another thing that motivated me to write this instructable were the contests going on at Instructables at this time (such as the micro-controller contest) which had very good prizes. Winning those contests would equip me with gear which would be useful for future instructables. Thank you for viewing my instructable.
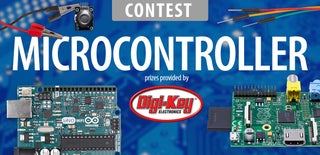
Participated in the
Microcontroller Contest 2017
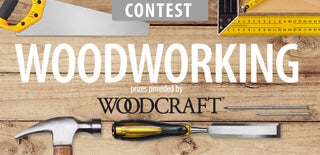
Participated in the
Woodworking Contest 2017
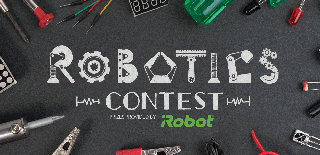
Participated in the
Robotics Contest 2017