Introduction: The Weather Grid (Powered by Intel Edison)
The Intel Edison is a very interesting beast. It features both an Intel processor running Linux and has Arduino functionality (GPIO, SPI, etc.). This project is a little different in that it uses both of those features.
In this Instructable, we’re going to build the Weather Grid. The Weather Grid is a device that displays the current weather conditions and temperature for your current location without any interaction (once setup). The front of the Weather Grid is a piece of hardboard (approximately 9” x 7”) with carved icons which represent weather conditions and a cut-out for the LCD screen. The weather condition icons are illuminated using a series of LEDs. The whole thing is powered by an Intel Edison which uses its Arduino side to control the hardware and its Linux side to retrieve and format the data.
Step 1: The Weather Grid Overview
As I mentioned, there are a lot of interwoven parts in this project. To best explain how it works check out the above diagram.
Requirements:
- Intel® Edison Compute Module (IoT):
- http://www.intel.com/content/www/us/en/do-it-yours...
- Intel® Edison Kit for Arduino: http://www.intel.com/buy/us/en/product/emergingte...
- X-Carve CNC (or function equivalent): https://www.inventables.com/technologies/x-carve
- I meter APA102 LED strip (36 LEDs)
- Grove Base Shield: http://www.seeedstudio.com/depot/Grove-Base-Shiel...
- Grove LCD RGB Backlight: http://www.seeedstudio.com/depot/Grove-LCD-RGB-Ba...
- Craft Foam: http://www.walmart.com/ip/Make-It-Fun-Floracraft-...
- Masking tape
- Craft Knife
- Sanding block
- Computer/Laptop
Step 2: Setting Up the Intel Edison
We have to start up setting up the Intel Edison. This procedure is very well documented elsewhere so to keep this section brief, I’m just going to quickly summarize and link to the appropriate information.
Image the Edison
The first things you need to do it are assemble your Edison, image it and install and setup the drivers and Arduino IDE. Intel has a great “Getting Started” guide for this that you can find here: https://software.intel.com/en-us/iot/library/edison-getting-started . As you progress through the Getting Started guide, make sure to note of the IP address your Edison is assigned. You’ll need this later. If you need to re-setup the wifi at a later time you can do so with:
configure_edison --wifi
Once you have completed the “Getting Started” guide you should load up the “Blink” sketch in the Arduino IDE to make sure everything is working properly.
We’re now ready to start.
Step 3: Programming the Edison (Arduino IDE)
The LEDs
The first component we need to get working is the LED strip. In this case we are using a 1 meter strip of APA102 that has 36 individually addressable LEDs. We are using these instead of the more popular WS2811/WS2812 strips because they use separate wires for their clock and data signals. The different versions of the Intel Edison firmware have issues which make them difficult to interface with the 3 wire LED strips, so using the WS2811/WS2812 strips is problematic.
To control the LED strip we need four pins on the GPIO as shown here:
GND --> GND
DI --> PIN 11 (MOSI)
CI --> PIN 13 (SPI Clock)
5V --> 5V
The important issue here is identifying the proper pins on the Edison Arduino board. The Intel Edison Kit for Arduino Hardware Guide ( http://download.intel.com/support/edison/sb/ediso... ) identifies those pins as the ones needed for “SPI send data” (PIN 11) and “SPI clock control” (PIN 13).
Now that the proper pins are identified we have to write some code to push the LEDs. None of the existing libraries like FASTLED or NeoPixel work with the APA102s and the Edison so we’ll need some custom code. The best explanation I’ve found is in the Intel Community forums here: https://communities.intel.com/message/335874#3358... . Basically, we need to create a Buffer object and then assign the RGB color values by passing them through the Buffer. Once you have the APA102 strip connected to the pins as described, you can run the following code to test the system. Note that since we are only using a couple of LEDs at a time in the final project, we won’t be using an external power supply.
LCD
The LCD we’re using is the “Grove - LCD RGB Backlight.” It comes as part of the Grove Starter Kit Plus from SeeedStudio and is also available separately. To connect it to the Edison we’ll use the “Grove Base Shield” and one of the included four wire cables. Once it is connected you’ll need to download the “Grove_LCD_RGB_Backlight” Arduino Library from: https://github.com/Seeed-Studio/Grove_LCD_RGB_Bac... . You’ll then need to put a copy of the “libraries” directory for your Arduino IDE installation. Once you get the library files in place you should run the “fade” or “HelloWorld” example scripts as shown in the image.
Network Server
The Intel Edison comes with all of the features of an Arduino WIFI shield, so no additional hardware or shields are needed for the Arduino side of the Edison to access the WIFI systems. Now we just need to setup them up and test them. To do that, you’ll open the Arduin IDE and run the example sketch “SimpleWebServerWIFI.” If your Arduino IDE install didn’t come installed with the “wifi” sketches, you can download them from github ( https://github.com/codebendercc/arduino-library-f... ). Now you just need to customize the sketch with your network’s SSID (name) and password, and you should be good.
Putting it all together
Now we just need to write a sketch that will utilize all of the parts we’ve just setup. Here is the sketch you’ll need:
https://github.com/nam37/weathergrid/blob/master/W...
I’ve tried to comment out the sketch to answer any questions, but here is a quick overview of the sketch.
- The first 70 or so lines define the required constants, define some needed variables, and “include” the necessary libraries.
- The next 100 or so lines are the main “loop” of the sketch. Here the initialized webserver is waiting for incoming connections. When a connection is encountered, it tries to identify it as a weather code “WW” or as a temperature code “ZZ”. If either of those as found then the proper LED are lit and/or the proper message is displayed on the LCD.
- The rest of the sketch consists of a collection of function used to control the LEDs and the LCD.
A note on persistence...
Some Intel Edison firmware versions have a bug which breaks Arduino sketch persistence. If you find that your Arduino scripts are not automatically being reloaded on reboot, then you’ll need to address this. There is a great thread in the Intel Edison community that addresses this here: https://communities.intel.com/thread/77945
Step 4: Setting Up the Web Server (Apache2)
Setting up Apache2
As I described above, we are going to use a PHP script on the Linux side of the Edison to collect the weather data and pass it to the Arduino sketch. So the first thing we need to do is install Apache2 (a popular webserver) and then install PHP.
It install it, you’ll need to use “AlexT’s” software repository. You can find it here (http://alextgalileo.altervista.org/edison-package-repo-configuration-instructions.html ) along with all the needed documentation. Once the repo is setup you can run the following to install apache:
opkg install apache2
opkg install php-modphp
Once Apache2 is installed you’ll need to change the port that the Apache2 service will watch. You do that by editing the Apach2 config file: /etc/apache2/httpd.conf
There are two ways to edit the file. You can use an editor like VI to create and edit the file in place, or you can use WinSCP to remotely manipulate the file. WinSCP works over a SSH connection to allow you to manipulate the file system of a remove Linux box. For more information on WinSCP and the Intel Edison you should read over the following Instructable: https://www.instructables.com/id/Intel-Edison-Upda...
Once you’re ready to edit the file locate the “listen” line (around line 52) and update it to:
Listen 84
Now you’ll need to configure Apache2 to start on reboot (commonly refereed to as making it persistent) and you’ll also want to start the service. You can do those things with the following commandlines:
systemstl stop apache2
systemstl enable apache2
systemstl start apache2
Apache2 and PHP should now be running on your Edison. The default directory for the root of the webserver is at : /usr/share/apache2/htdocs.
It’s now time to test Apache2. Load a web browser on a computer on the same network, and access the Edison at:
http://[EDISONS-IP]:84/index.html
The rename “index.html” to “index.php” and used the other computer to load:
http://[EDISONS-IP]:84/index.php
Setting up the PHP script.
Now we’ll need to put the "weather-set-1.php" file (linked below) into the Edison’s web root (/usr/share/apache2/htdocs). You can use either WinSCP or create and edit the file with an editor like VI.
https://github.com/nam37/weathergrid/blob/master/w...
Once the file is created or copied over you can test the script using:
http://[EDISONS-IP]:84/weather-set-1.php/weather-s...
and
http://[EDISONS-IP]:84/weather-set-1.php/weather-s...
As before, it’s outside of the scope of this Instructable to fully explain every line of the script, but in general there are two important things going on here. First we are using ipinfo.io for geolocation. To do this we use “file_get_contents” to hit the ipinfo.io site and they reply back with our zip code. We then take this zip code and (using “file_get_contents” again) request our weather from Yahoo Weather using an edited version of a script from http://www.hastuts.com/display-weather-forecast-b... . Finally, we take the weather or temperature data and pass it to the Arduino via the web server running on the Arduino.
A more complete structure of the script:
- The beginning of the script sets the local time zone. You should set yours to the applicable time zone. For more information, look here: http://php.net/manual/en/function.date-default-ti...
- Next script determines if we’re attempting to set the temperature or the weather code based on the querystring.
- Finally the script ends with the functions the make it all work.
Step 5: Setting Up the Scheduler (cronie)
In order to automatically update the weather on reboot and refresh the weather on occasion, we are going to use cron jobs. To do that, we first have to install cronie (a crontab service) on the Edison. We’ll install cronie using the AlexT repo we used before to install Apache2 and php. The commandline to install cronie is:
opkg install cronie
Now that cronie is installed we’ll need to use VI to create two cron jobs. The first cron job will update the weather every 5 minutes and the second will update the temperature every 6 minutes. Feel free to adjust these values to whatever you want. The two cron jobs are:
*/5 * * * * curl --silent 'http://127.0.0.1:84/weather-set-1.php'
*/6 * * * * curl --silent 'http://127.0.0.1:84/weather-set-1.php?temp=Y'
You can set them up using the command:
crontab -e
Then verify they were successfully created using:
crontab -l
…which should show:
*/5 * * * * curl --silent 'http://127.0.0.1:84/weather-set-1.php'
*/6 * * * * curl --silent 'http://127.0.0.1:84/weather-set-1.php?temp=Y'
We are now setup to refresh the weather and temperature automatically.
Step 6: Assembling the Weather Grid
To make the physical Weather Grid itself, I used a X-Carve CNC from Inventables. I found a collection of weather icons in SVG format on the internet and imported them into Easel. Easel is Inventables online software they use to power their CNC. I’ve shared my project here:
http://easel.inventables.com/projects/iBYXHg6SuodOIH_XewO_GA
I then cut the file out of hardboard. Plywood, hardwood, or HDPE would also work great.
Once my carve was complete, I used sandpaper and a craft knife to clean it up and remove the tabs. If you choose to use a hardwood or HDPE this might not be as necessary, but generally hardboard needs to be cleaned up after a carve.
Next you’ll need to insert the LCD into the hardboard. It will be a tight fit, and it might require you to sand the hardboard around the opening a little.
Now we need to cut the craft foam that we are using to diffuse the light. I cut the rectangles about a 1/4 to a 1/2 inch thick. It’s OK if they are not the exact same size since the icons vary in size also. Just try to keep them all approximately the same thickness.
Now we need to use masking tape to attach the foam diffusers to the back of the hardboard behind the icons. Find pieces that match the size of the icons and try to keep them from touching. The more space between the diffusers the less bleeding that you’ll have with adjacent icons.
Now we need to attach the LEDs. The LED spacing on my strip allowed for two LEDs per icon. Use masking tape to attach the LED strips to the back. Take care to keep to the pattern described in the “SetWeather” function in the Arduino sketch. If your LEDs layout differently, make sure to note the numbers of the LEDs so that you can adjust the Arduino sketch. Once your LEDs are attached, fill in the rest of the back with additional strips of masking tape.
Step 7: You’re Done!
Good job! We’re done. All you need to do now is plug the
Edison in to a USB changer or a computer’s USB port and wait for the cron jobs to run and you should have a fully functional Weather Grid. Note that this Instructable has a lot of moving parts. From basic Linux to Arduino sketches to PHP scripting, there’s a lot going on here. If something doesn’t work right give it a second look. If you’re still having issues ask Google or leave a comment here and I’ll try to get you working.
Thanks for reading and happy making.
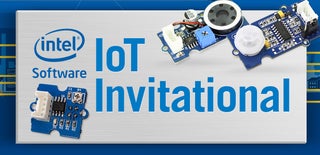
Runner Up in the
Intel® IoT Invitational