Introduction: Tic-Tac-Toe Robot
I won't give detailed, step by step instructions for building the robot because it's physical design does not need to be exactly the same as mine. I used LOZ blocks. You can build the robot however you want using whatever blocks you have. It could also be made from Meccano or Lego.
The supplied Arduino code (see step 4) allows you to teach the robot arm where each position on the board is using the TV remote. The positions are stored in the controllers EEPROM memory. This means your robot can be any size or shape as long as the arm can reach all positions.
The program also displays the game on your PC via the serial monitor so you can play against the controller even without the robot. This is ideal for experimenting with the robot's A.I. My code is not very smart so even at the hardest level you can beat it.
Step 1: Servos and Gearboxes
My robot is made with LOZ blocks, these are a Chinese clone of K'nex but the quality seems to be just as good. Originally I converted 4 of the LOZ geared motors to work as servos but unfortunately the clutch built into the gear train introduced too much play. This reduced the accuracy of the arm too much to be useful.
If you do want to modify these motors they work much better as continuous rotation servos. You might need to change the motors as the original motors are designed for 3V and draw a lot of current. The motors are commonly found in many electronic stores and 6V versions are available.
I still used these for the gripper and raising the balls for collection where accuracy was not important but I found standard servos worked better for positioning the arm and could be integrated into the blocks quite easily.
Step 2: Robot Construction
The arm can be an X-Y axis system or it can be a polar arm like mine with a shoulder and elbow. The sample code uses a servo for each axis or joint.
The gripper can be any design you want but to use the sample code it must be driven by a servo and have 3 positions, closed, hold and open. The video below was taken when I tested my gripper. When the "fingers" are all the way down they are a tight fit around the ball which allows them to hold the ball when raised. When the fingers raise high enough, a "thumb" in the center pushes the ball out.
Step 3: Wiring the Micro Magician
For this robot I have use a Micro Magician V2. You could also use an original Micro Magician. The only difference is that the new version can work at lower voltages (3.6V instead of 4.5V) and has an additional 5V regulator. I am powering this robot with 4x AA NiMh rechargeable batteries (4.8V).
The nice thing about the Micro Magicians is that they make the wiring so easy. The IR receiver built into the board allows any Sony remote control or universal remote to be your keypad. The Micro Magicians come with an Arduino library that includes a Sony IR decoder. This makes reading the signals from your remote very easy. An indication LED on the controller lets you know if the receiver is detecting a signal. This is useful for debugging.
The only wiring you need to do is to plug in your 4 servos and the batteries. Even though the Micro Magician is a 3.3V controller it still allows you to power your servos directly from the batteries and has reverse polarity protection in case you accidentally connect the batteries the wrong way.
The [IOpins.h] tab is effectively your wiring diagram. It tells you where each servo must connect to. Note that the ground pin goes to the outside edge of the PCB.
Step 4: The Code
You will need a Micro Magician (does not matter which version) and the microM library which can be downloaded from here: https://sites.google.com/site/daguproducts/home/arduino-libraries
If you prefer you could use another Arduino board. You will probably need a breadboard and jumper wires to connect an IR receiver and your servos. You may prefer to use Ken Shirriff's multi-protocol IR library which can be found here: http://www.righto.com/2009/08/multi-protocol-infrared-remote-library.html
As you can see I have broken my code down into logical sections and put them in different tabs. This makes it much easier to edit your code.
[TicTacToe] this first tab is your main code. It has your Setup() function where all your initialization is done and the Loop() function where the game is played. The Loop() function consist of 3 sections.
- Mode Selection - the program looks for any new IR commands and changes modes if required.
- Players Move - when it is your turn this section allows you to make your move.
- Robots Move - depending on the level of difficulty, different strategies are selected here.
[IOpins.h] this tab is you wiring diagram. If you wish to change which pin a servo is connected to then just change the pin number here. As the IR receiver is hardwired to D4 it is not listed.
[Constants.h] this tab contains constants used within the program. IR receivers codes and preset values for the gripper and pins mechanism servos are defined here. As you robot will be different to mine you will need to change these values to suit. All values are in µS for use with the writeMicroseconds() command.
[Calibrate] this function is used to teach the robot where each position on the board is. Initially all positions are equal to both arm servos being in the center position. As you teach the arm, new positions are saved in the processors EEPROM so that even if power is turned off the robot will not forget what you taught it.
[eepromFunctions] when the program first runs the EEPROM is read so that the robot will know the positions of the board. When you calibrate the robot the new positions are written to the EEPROM so that when the power is turned off the robot will not forget the positions.
[DisplayGrid] the game is displayed on the serial monitor for debugging purposes and allows you to play Tic Tac Toe against the Micro Magician using just a TV remote. The robot is only needed to impress your friends and family and make the game more fun to play. 0 represents an empty position. The robots balls are represented with a 1. Your balls are represented by a 2.
[MoveArm] as the name suggest, this function moves the arm from one position on the board to another. my code breaks the move down into small steps so that the arm moves slowly and smoothly. Bigger moves are broken into more steps so that all moves happen at approximately the same speed.
[CheckForWin] after each move the board is checked to see if there is a winner. All rows, columns and diagonals are checked for 3 of the same.
[ClearBoard] at the end of the game to robot collects all the balls and puts them back in their chute. The robot relies on memory to determine if the ball belongs to the player or the robot and returns the ball to the correct chute. The pin mechanism raises the balls high enough for the gripper to collect them and then lowers the balls again so the arm can move freely.
[RandomMove] at the easiest level, all of the robots moves are random. This mode is intended for teaching children the game for the first time. A random move is also used by the robot when other strategies fail. To make game-play less predicable (more enjoyable), when it is the robots turn to start a game the robot will start with a random move.
[BlockPlayer] this function is used by the robot to look at your moves and determine if you are about to win. If the robot detects you have two balls in a line with the third position being free then it will place it's ball in the free position to block you. This function is not used in the easiest level which is intended for teaching children how to play.
[MakeLine] this function is similar to [BlockPlayer] except that it is used by the robot to get 3 in a row. This function is not used in the easiest levels.
[SecondMove] as a game of Tic Tac Toe does not have many moves, the second move is often where your strategy will come into play. The robot uses this in the hardest level to try and beat you. I admit, this part of the code could be improved. I am not good at strategy games.
Attachments
Step 5: Remote Control
If you are using a Micro Magician and the microM library then you can use any Sony compatible remote control. I am using a universal remote programmed to behave like a Sony TV remote control. If you modified my code and are using the multi-protocol IR library then it will be possible to use other remote controls as well.
I have shown a photo of the universal TV remote I used and how I organized my keys. You will need to adjust the code as different remotes will have different button configurations and may generate different codes for those buttons. These can be changed in the [Constants.h] tab.
If you use the microM library then the IR_receiver example code can be used to see what code is generated by your buttons. If you are using the IRremote library then use the IRrecvDemo example code instead.
Choose a layout that makes sense to you and draw a picture of it. Once you know what codes each button generates then you can write those codes down in your picture of the remote and use that to change the IR receiver values in my code.
Step 6: Calibration
When you first build your robot the code needs to be calibrated to work with it. With your computer connected to the robot via the USB cable, use the Arduino serial monitor to see what the program is doing and put the robot into calibration mode.
In calibration mode select the position you want to calibrate 1-9, player / robot pick up or drop off position. Then use your arrow keys to move the arm forward/backward and left/right. Once the arm is in the correct position press the update key and that position will be stored in the robots EEPROM memory.
You can decrease the amount the arm moves with each press of the button for fine adjustments or increase the amount to move the arm quicker.
Once you have saved a position in memory you can then select another position to calibrate. Calibration mode can be entered at any time during a game without the game needing to be reset. Once you are happy with all positions simply press the mode button again and the robot will return to game mode.
Calibration mode does not calibrate the gripper and ball raise / lower servos. As these are simple devices where the servo travels either full travel or half travel it is quite easy to adjust the values in the [Constants.h] tab.
Step 7: Play
Once you have your robot calibrated and working properly it is time to play but don't stop here. This is just the first step.
You may want to add an LCD display so you do not need the serial monitor.
Adjust the robots artificial intelligence to make it smarter but don't make it too smart. There is nothing more boring than a robot that wins or draws every time.
Increase the size of the playfield to 5 x 5. This will make the game much more challenging.
If Halloween is coming up then perhaps you should make the robot arm look like "Thing" from the "Adams Family".
Good luck and enjoy!
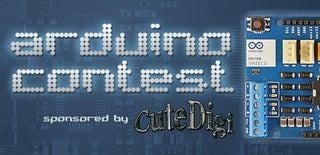
Participated in the
Arduino Contest
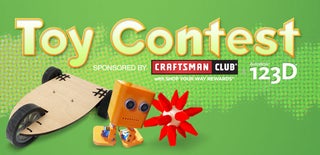
Participated in the
Toy Contest