Introduction: TinyDuino RC Telemetry (With GPS!)
RC telemetry is just really neat; if you fly or drive RC chances are you’ve wished at one time or another you had a way to track all the data from your model. Live GPS tracking can be helpful locating a model with a mind of its own. Off the shelf telemetry can be a little confusing and expensive to implement and not as fun or flexible as a DIY solution. The TinyDuino is a perfect platform to build an RC telemetry unit off for two reasons: the size is awesome for almost all RC scales but something to also consider is the wide range of equally ‘TinyShields’ that are available and make up the heart of this project.
This tutorial will cover the key points in creating and understanding an expandable RC telemetry device. It will function independently of the rest of your RC electronics so even the most catastrophic failure won't affect your ability to locate the smoking remains of your once beloved model.
Step 1: Dependencies and Libraries
With this project we require three large libraries, an unfortunate side effect of this is we won't have very much extra space for our own variables. With that in mind some care must be taken to not abuse the remaining memory. Static resource allocation is a must.
- TinyGPS++: http://arduiniana.org/libraries/tinygpsplus/
- Tinyscreen Lib: https://github.com/TinyCircuits/TinyCircuits-Tiny...
- RadioHead: https://github.com/TinyCircuits/TinyCircuits-Tiny...
Include only these header files:
- Air Unit: SPI.h, RH_RF22.h, TinyGPS++.h, SoftwareSerial.h
- Ground Unit: TinyScreen.h, Wire.h, RH_RF22.h, SPI.h
Step 2: Understanding Structures, Pointers, and Basic Memory Management.
To send large quantities of data over any form of communications link the most efficient way to go about it is send the raw bytes from the transmitter and read them back into memory at the receiver. This is not particularly difficult but it makes use of some unique low level code you may not have used before. Additionally since we will be tinkering with memory pointers and large data structures it's possible to crash the arduino very quickly.
Structures: A structure is somewhat like a more basic object. It contains a set of variables called properties that can be accessed with the dot operator. E.g:
//define data structure struct myStruct { int a = 0; int b = 0; int c = 0; }; // create instance of myStruct myStruct ms; // assign values to the new instance of myStruct ms.a = 10; ms.b = 11; ms.c = 21;
We use a data structure to represent this data because it will have a fixed size and structure, meaning that when we read our structure back into our receiver’s memory the code will automatically know what all the data means. You cannot use a String variable in a structure like this because the String variable takes a dynamic amount of memory and will cause unexpected errors. Rather if you want to store strings use an array of chars with a fixed size. Another thing to keep in mind is that data structures are the size of the sum of their parts. so they can get very large. Read more:Here
Pointers: A pointer in the context of a C language is a way of referencing an address in physical memory. This is useful for us because we cannot directly create a copy of a received structure. Rather we create a pointer to a nonexistent structure in memory then fill that memory with the stream of data we received from our transmitter. However because we are using a pointer to a structure rather than a ‘real’ structure we have to use the arrow operator (->) to address our properties rather than the dot operator. If this is confusing study the code in the next two steps and see how these concepts are used. Read More:Here
Memory Management: Because structures are large creating them at runtime is a great way to fill the arduino's memory and cause crashes. Instead we should statically allocate our structures and other static data by declaring them outside the loop() or start() function. to read more on the best practices for SRAM memory and variables Read More:Here
Step 3: Hardware Assembly
RF22 assembly:
For the health and longevity of the 433Mhz radio install the antenna before use.
Ground Unit:
- Tiny Screen
- 433Mhz Radio
- Tinyduino with Li-po support
Air Unit:
- GPS (Should be on top)
- Other sensors (Optional)
- 433Mhz radio
- Tinyduino with Li-po support
Notes:
- Tinyduino with Li-po or external battery required to allow for proper current flow to the screen, GPS, and radio
- GPS unit should be on top to allow for the best fix possible.
- use THIS graphic to ensure sensor compadability
Step 4: Code: Air Unit
The Air Unit code looks like this:
Step 5: Ground Unit Code
This is the code for the unit that displays the information:
Note: At the time of writing codebender does not support compiling this code. Please Download the .INO file and compile it on your computer.
Step 6: Using Your Telemetry Unit
Now for the fun part! Get the Li-po batteries charged with the USB and then take your model out to the field. Make sure to mount the air unit with the GPS facing the sky with as little between it and space as you can manage. Switch the telemetry system on and make sure you can get a fix. Have fun flying!
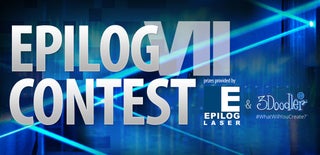
Participated in the
Epilog Contest VII