Introduction: Touch LED Table
This instructable is step by step how to build a touch led table
Step 1: Step 1: Get a Square Table
I used a small bedside table purchased from walmart
Step 2: Step 2: Cut a Hole in the Table
The next step was to cut a square hole in the top of the table and then I spray painted the entire table flat black. I also drilled a hold in the side of the table for the power cord to go later.
Step 3: Step 3: Ardino Code and Breadboard
I started with the following code and a push button with the setup shown to power the led strip.
CODE:
// This is a demonstration on how to use an input device to trigger changes on your neo pixels.
// You should wire a momentary push button to connect from ground to a digital IO pin. When you
// press the button it will change to a new pixel animation. Note that you need to press the
// button once to start the first animation!
#include
#define BUTTON_PIN 2 // Digital IO pin connected to the button. This will be
// driven with a pull-up resistor so the switch should
// pull the pin to ground momentarily. On a high -> low
// transition the button press logic will execute.
#define PIXEL_PIN 6 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 30
// Parameter 1 = number of pixels in strip, neopixel stick has 8
// Parameter 2 = pin number (most are valid)
// Parameter 3 = pixel type flags, add together as needed:
// NEO_RGB Pixels are wired for RGB bitstream
// NEO_GRB Pixels are wired for GRB bitstream, correct for neopixel stick
// NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels)
// NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip), correct for neopixel stick
Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH;
int showType = 0;
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
}
void loop() {
// Get current button state.
bool newState = digitalRead(BUTTON_PIN);
// Check if state changed from high to low (button press).
if (newState == LOW && oldState == HIGH) {
// Short delay to debounce button.
delay(20);
// Check if button is still low after debounce.
newState = digitalRead(BUTTON_PIN);
if (newState == LOW) {
showType++;
if (showType > 10)
showType=0;
startShow(showType);
}
}
// Set the last button state to the old state.
oldState = newState;
}
void startShow(int i) {
switch(i){
case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/off
break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); // Red
break;
case 2: colorWipe(strip.Color(255, 96, 0), 50); // orange
break;
case 3: colorWipe(strip.Color(255, 255, 0), 50); // yellow needs help
break;
case 4: colorWipe(strip.Color(0, 255, 0), 50); // green
break;
case 5: colorWipe(strip.Color(0, 0, 255), 50); // blue
break;
case 6: colorWipe(strip.Color(38, 222, 220), 50); // Teal
break;
case 7: colorWipe(strip.Color(75, 0, 13), 50); // Purple
break;
case 8: colorWipe(strip.Color(255, 0, 255), 50); // Pink
break;
case 9: colorWipe(strip.Color(252, 253, 253), 50); // white
break;
}
}
// Fill the dots one after the other with a color
void colorWipe(uint32_t c, uint8_t wait) {
for(uint16_t i=0; i
strip.setPixelColor(i, c);
strip.show();
delay(wait);
}
}
void rainbow(uint8_t wait) {
uint16_t i, j;
for(j=0; j<256; j++) {
for(i=0; i
strip.setPixelColor(i, Wheel((i+j) & 255));
}
strip.show();
delay(wait);
}
}
// Input a value 0 to 255 to get a color value.
// The colours are a transition r - g - b - back to r.
uint32_t Wheel(byte WheelPos) {
WheelPos = 255 - WheelPos;
if(WheelPos < 85) {
return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3);
} else if(WheelPos < 170) {
WheelPos -= 85;
return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3);
} else {
WheelPos -= 170;
return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
}
Step 4: Step 4: Changing the Code
The next was modifying the code to account for the capacitive touch.
CODE:
#include #include
#define PIXEL_PIN 6 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 30 int showType; Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800); CapacitiveSensor tapper = CapacitiveSensor(2, 4);
void setup() { tapper.set_CS_AutocaL_Millis(0xFFFFFFFF); // turn off autocalibrate on channel 1 - just as an example Serial.begin(9600); strip.begin(); strip.show(); // Initialize all pixels to 'off
}
void loop() { long total1 = tapper.capacitiveSensor(30);
if (total1 >= 300) { if (showType > 9){ showType = 0; startShow(showType);} else { startShow(showType); showType++; } }
Serial.print("\t"); // tab character for debug window spacing Serial.print("\t"); Serial.print(total1); // print sensor output 1 Serial.print("\n");
}
void startShow(int i) {
switch (i) { case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/off break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); // Red break;
case 2: colorWipe(strip.Color(255, 96, 0), 50); // orange break;
case 3: colorWipe(strip.Color(255, 255, 0), 50); // yellow needs help break;
case 4: colorWipe(strip.Color(0, 255, 0), 50); // green break;
case 5: colorWipe(strip.Color(0, 0, 255), 50); // blue break;
case 6: colorWipe(strip.Color(38, 222, 220), 50); // Teal break;
case 7: colorWipe(strip.Color(75, 0, 13), 50); // Purple break;
case 8: colorWipe(strip.Color(255, 0, 255), 50); // Pink break;
case 9: colorWipe(strip.Color(252, 253, 253), 50); // white
} }
int lightCycle = 0;
// Fill the dots one after the other with a color void colorWipe(uint32_t c, uint8_t wait) { for (uint16_t i = 0; i < strip.numPixels(); i++) { strip.setPixelColor(i, c); strip.show(); delay(wait); } }
// Input a value 0 to 255 to get a color value. // The colours are a transition r - g - b - back to r. uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if (WheelPos < 85) { return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } else if (WheelPos < 170) { WheelPos -= 85; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } else { WheelPos -= 170; return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); } }
Step 5: Step 5: Putting the Table Together
The next thing I did was put aluminum inside the cut out in the table, and then put a piece of plexiglass underneath and attached the capacitive touch wire to the aluminum and super glued the led strip on the inside of the table.
Step 6: Step 6: Finalizing
The last step was to put a mirror underneath the plexiglass and the led strip to help shine the light upward and then mounted both boards to a piece of wood and screwed it onto the bottom of the table.
Step 7: LED Touch Table
Final Code:
#include <CapacitiveSensor.h>
#include <Adafruit_Neopixel.h>
#define PIXEL_PIN 6 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 28 int showType; Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800); CapacitiveSensor tapper = CapacitiveSensor(2, 4);
void setup() { tapper.set_CS_AutocaL_Millis(0xFFFFFFFF); // turn off autocalibrate on channel 1 - just as an example Serial.begin(9600); strip.begin(); strip.show(); // Initialize all pixels to 'off
}
void loop() { long total1 = tapper.capacitiveSensor(30);
if (total1 >= 2000) { if (showType > 9){ showType = 0; startShow(showType);} else { startShow(showType); showType++; } }
Serial.print("\t"); // tab character for debug window spacing Serial.print("\t"); Serial.print(total1); // print sensor output 1 Serial.print("\n");
}
void startShow(int i) {
switch (i) { case 0: colorWipe(strip.Color(0, 0, 0), 50); // Black/off break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); // Red break;
case 2: colorWipe(strip.Color(255, 96, 0), 50); // orange break;
case 3: colorWipe(strip.Color(255, 255, 0), 50); // yellow needs help break;
case 4: colorWipe(strip.Color(0, 255, 0), 50); // green break;
case 5: colorWipe(strip.Color(0, 0, 255), 50); // blue break;
case 6: colorWipe(strip.Color(38, 222, 220), 50); // Teal break;
case 7: colorWipe(strip.Color(75, 0, 13), 50); // Purple break;
case 8: colorWipe(strip.Color(255, 0, 255), 50); // Pink break;
case 9: colorWipe(strip.Color(252, 253, 253), 50); // white
} }
int lightCycle = 0;
// Fill the dots one after the other with a color void colorWipe(uint32_t c, uint8_t wait) { for (uint16_t i = 0; i < strip.numPixels(); i++) { strip.setPixelColor(i, c); strip.show(); delay(wait); } }
// Input a value 0 to 255 to get a color value. // The colours are a transition r - g - b - back to r. uint32_t Wheel(byte WheelPos) { WheelPos = 255 - WheelPos; if (WheelPos < 85) { return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } else if (WheelPos < 170) { WheelPos -= 85; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } else { WheelPos -= 170; return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); } }
Attachments
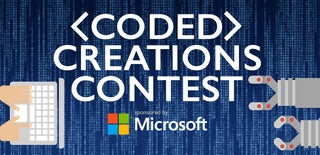
Participated in the
Coded Creations