Introduction: Twinkle, Twinkle Little Star
However, I am more of a only software guy and don't usually have enclosures or any hardware.
I don't like art either (I can't seem to draw good people.)
So, when I have a project to do, I use the K.I.S.S. principle.
Step 1: Twinkling Materials.
Wire
Male headers
LEDs (Preferably Yellow however, when I ordered a pack of Yellow LEDs, they gave me orange. Therefore, I used warm white.)
Cardboard (I used corrugated but other types are fine .)
Paper+Design printed (on ible.)
Glue (Don't use hot glue or liquid glue because it makes bumps under the paper that are not very pleasant.)
Hot Glue (read the ible and you will comprehend.)
Yellow Marker (highlighters)
Step 2: Design.
Start by going to this link and printing the star at 200%.
Step 3: Assembly Part 1.
Now take the star and cut it out nicely. Then take the corrugated cardboard and glue the star to the cardboard. Now, cut out the star with the cardboard. attached. You may want to use scissors but it may not cut too well. An X-Acto Knife may do better but it is more dangerous.
If you are using scissors, cut out a general outline of what it will look like.
Then, clean out the extra by trimming it down to perfection.
Next use a yellow marker (or highlighter) to color it in.
WARNING: HIGHLIGHTERS AND MARKERS DON'T MIX. IT WILL TURN OUT WATERY IF YOU DO MIX SO DON'T.
Step 4: Program.
Program the code:
//feel free to make changes
//note: pin 5 and 6 act weird in the beginning - an arduino bug
byte led1 = 3;
byte led2 = 5;
byte led3 = 6;
byte led4 = 9;
byte led5 = 10;
byte led6 = 11;
int x = 0;
int y = 0;
int steps = 1; //change if needed, defines the steps between 0 and 255, a lower number is smoother
//make sure the variable "steps" is a factor of 255; any of the below numbers
//factors of 255 are : 1,3,5,15,17,51,85,255
//sorry for a lot of notes, but remember to change variable "delaytime" according to variable "steps"
//delay is in milliseconds for below
int delaytime = 10; //change if needed, delay between increments of PWM
//850 milliseconds is on-off/off-on time, in 17 step increments of brightness
int delaytime2 = 1000; //change if needed, delay between switching of leds
void setup (){
pinMode (led1, OUTPUT);
pinMode (led2, OUTPUT);
pinMode (led3, OUTPUT);
pinMode (led4, OUTPUT);
pinMode (led5, OUTPUT);
pinMode (led6, OUTPUT);
do {
x = x + steps;
analogWrite (led1, x);
analogWrite (led2, x);
delay (delaytime);
}
while (x != 255);
}
void loop (){
y = 0;
x = 255;
delay (delaytime);
do{
y = y + steps;
x = x - steps;
analogWrite (led3, y);
analogWrite (led1, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
y = 0;
x = 255;
do{
y = y + steps;
x = x - steps;
analogWrite (led4, y);
analogWrite (led2, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
y = 0;
x = 255;
do{
y = y + steps;
x = x - steps;
analogWrite (led5, y);
analogWrite (led3, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
y = 0;
x = 255;
do{
y = y + steps;
x = x - steps;
analogWrite (led6, y);
analogWrite (led4, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
y = 0;
x = 255;
do{
y = y + steps;
x = x - steps;
analogWrite (led1, y);
analogWrite (led5, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
y = 0;
x = 255;
do{
y = y + steps;
x = x - steps;
analogWrite (led2, y);
analogWrite (led6, x);
delay (delaytime);
}
while (y != 255 && x != 0);
delay (delaytime2);
}
Step 5: Assembly Part 2.
You are NOT done yet...The LEDs have not been wired! Now, this just like in my Random Arduino LED Fader
Follow the pictures to secure the LEDs.
Remember: Nobody (or anything) is perfect so it is okay if you have a few failures.
Now, you must tin the LED leads and solder some solid core wire to it.
Make sure you note which is positive and which is negative. I gave up on color coding but it still works fine.
Randomly pick a positive lead and connect it to a pin (3, 5, 6, 9, 10 ,11)
Then take all the negative pins on the LEDs and wire them to ground.
Step 6: Completion.
This is the step where you get to sit back, relax and enjoy the show.
Questions can be left in a comment below.
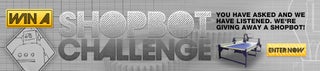
Participated in the
ShopBot Challenge
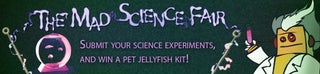
Participated in the
The Mad Science Fair
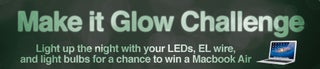
Participated in the
Make It Glow Challenge
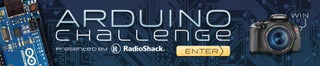
Participated in the
Arduino Challenge
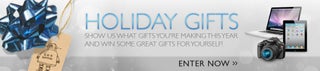
Participated in the
Holiday Gifts Challenge
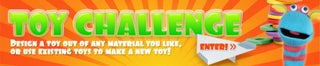
Participated in the
Toy Challenge 2