Introduction: Arduino Based Twitter Feed Bracelet
The idea was to create a messaging system that was both cheap and worked independently. The system had to be able to send short alphanumeric messages one way. I selected the arduino systems as the base as i was already familiar with it.
The project uses temboo, signing up for the account was both quick and easy, as were obtaining the correct tokens. The project can be easily modified to take input from other sources.
The project is two parts, the first was the base station, this has to be connected to the internet directly (i didn't want to have to have a laptop in the middle relaying twitter feeds). The second part is the wrist band with an OLED display.
Components and Cost
Base:
Arduino Yun - 55.05
NRF24L01 - 1.00
Strap/Band:
OLED Screen - 10.00
NRF24L01 - 1.00
Arduino Nano Clone - 5.00
Strap - 5.00
Total = 77.05
All prices approx and in GBP
Project should take about 4 hours
Step 1: Making the Base
The base is the easiest part to build, but was a pain to wire..
You may notice that I've used a base for the NRF24L01 in the photo, this was used to increase the stability of the board. They are very sensitive to small voltage variations, this was just a precaution added during a test.
To wire the NRF24L01 to the YUN you have to make use of three of the ICSP male pins shown in the diagram, use the NRF24L01 pin digram and the table below to wire :)
NRF24L01: Function: YUN Pin
1: GND: GND
2: VCC: 3.5v
3: CE: D9
4: CSN: D10
5: SCK: SCK
6: MOSI: MOSI
7: MISO: MISO
8: IRQ:
please find below the code used for the transmitter. There are three files needed, once you have generated the arduino .ino file, locate it and save the others in the same folder.
****Arduino Code****
#include
#include
#include
#include
#include
#include
#include "TembooAccount.h" // contains Temboo account information
/*** SUBSTITUTE YOUR VALUES BELOW: ***/
// Note that for additional security and reusability, you could
// use #define statements to specify these values in a .h file.
const String TWITTER_ACCESS_TOKEN = "Insert Your Token";
const String TWITTER_ACCESS_TOKEN_SECRET = "Insert Your Token";
const String TWITTER_API_KEY = "Insert Your Token";
const String TWITTER_API_SECRET = "Insert Your Token";
int numRuns = 1; // execution count, so this doesn't run forever
int maxRuns = 10; // the max number of times the Twitter HomeTimeline Choreo should run
int msg[1];
RF24 radio(9,10);
String theMessage = " ";
char incomingByte = 0;
const uint64_t pipe = 0xE8E8F0F0E1LL;
void setup() {
Serial.begin(9600);
// For debugging, wait until a serial console is connected.
delay(4000);
while(!Serial);
Bridge.begin();
radio.begin();
radio.openWritingPipe(pipe);
}
void loop()
{
// while we haven't reached the max number of runs...
if (numRuns <= maxRuns) {
Serial.println("Running ReadATweet - Run #" + String(numRuns++));
TembooChoreo HomeTimelineChoreo;
// invoke the Temboo client.
// NOTE that the client must be reinvoked, and repopulated with
// appropriate arguments, each time its run() method is called.
HomeTimelineChoreo.begin();
// set Temboo account credentials
HomeTimelineChoreo.setAccountName(TEMBOO_ACCOUNT);
HomeTimelineChoreo.setAppKeyName(TEMBOO_APP_KEY_NAME);
HomeTimelineChoreo.setAppKey(TEMBOO_APP_KEY);
// tell the Temboo client which Choreo to run (Twitter > Timelines > HomeTimeline)
HomeTimelineChoreo.setChoreo("/Library/Twitter/Timelines/HomeTimeline");
// set the required choreo inputs
// see https://www.temboo.com/library/Library/Twitter/Ti...
// for complete details about the inputs for this Choreo
HomeTimelineChoreo.addInput("Count", "1"); // the max number of Tweets to return from each request
HomeTimelineChoreo.addInput("AccessToken", TWITTER_ACCESS_TOKEN);
HomeTimelineChoreo.addInput("AccessTokenSecret", TWITTER_ACCESS_TOKEN_SECRET);
HomeTimelineChoreo.addInput("ConsumerKey", TWITTER_API_KEY);
HomeTimelineChoreo.addInput("ConsumerSecret", TWITTER_API_SECRET);
// next, we'll define two output filters that let us specify the
// elements of the response from Twitter that we want to receive.
// see the examples at http://www.temboo.com/arduino
// for more on using output filters
// we want the text of the tweet
HomeTimelineChoreo.addOutputFilter("tweet", "/[1]/text", "Response");
// and the name of the author
HomeTimelineChoreo.addOutputFilter("author", "/[1]/user/screen_name", "Response");
// tell the Process to run and wait for the results. The
// return code will tell us whether the Temboo client
// was able to send our request to the Temboo servers
unsigned int returnCode = HomeTimelineChoreo.run();
// a response code of 0 means success; print the API response
if(returnCode == 0) {
String author; // a String to hold the tweet author's name
String tweet; // a String to hold the text of the tweet
// choreo outputs are returned as key/value pairs, delimited with
// newlines and record/field terminator characters, for example:
// Name1\n\x1F
// Value1\n\x1E
// Name2\n\x1F
// Value2\n\x1E
// see the examples at http://www.temboo.com/arduino for more details
// we can read this format into separate variables, as follows:
while(HomeTimelineChoreo.available()) {
// read the name of the output item
String name = HomeTimelineChoreo.readStringUntil('\x1F');
name.trim();
// read the value of the output item
String data = HomeTimelineChoreo.readStringUntil('\x1E');
data.trim();
// assign the value to the appropriate String
if (name == "tweet") {
tweet = data;
} else if (name == "author") {
author = data;
}
}
Serial.println("@" + author + " - " + tweet);
String steve = ("@" + author + " - " + tweet);
int messageSize = steve.length();
for (int i = 0; i < messageSize; i++){
int charToSend[1];
charToSend[0] = steve.charAt(i);
radio.write(charToSend,1);
}
msg[0] = 2;
radio.write(msg,1);
} else {
// there was an error
// print the raw output from the choreo
while(HomeTimelineChoreo.available()) {
char c = HomeTimelineChoreo.read();
Serial.print(c);
}
}
HomeTimelineChoreo.close();
}
Serial.println("Waiting...");
delay(90000); // wait 90 seconds between HomeTimeline calls
}
****RF24_config.h****
/*
Copyright (C) 2011 J. Coliz
This program is free software; you can redistribute it and/or
modify it under the terms of the GNU General Public License
version 2 as published by the Free Software Foundation.
*/
#ifndef __RF24_CONFIG_H__
#define __RF24_CONFIG_H__
#if ARDUINO < 100
#include
#else
#include
#endif
#include
// Stuff that is normally provided by Arduino
#ifdef ARDUINO
#include
#else
#include
#include
#include
extern HardwareSPI SPI;
#define _BV(x) (1<<(x))
#endif
#undef SERIAL_DEBUG
#ifdef SERIAL_DEBUG
#define IF_SERIAL_DEBUG(x) ({x;})
#else
#define IF_SERIAL_DEBUG(x)
#endif
// Avoid spurious warnings
#if 1
#if ! defined( NATIVE ) && defined( ARDUINO )
#undef PROGMEM
#define PROGMEM __attribute__(( section(".progmem.data") ))
#undef PSTR
#define PSTR(s) (__extension__({static const char __c[] PROGMEM = (s); &__c[0];}))
#endif
#endif
// Progmem is Arduino-specific
#ifdef ARDUINO
#include
#define PRIPSTR "%S"
#else
typedef char const char;
typedef uint16_t prog_uint16_t;
#define PSTR(x) (x)
#define printf_P printf
#define strlen_P strlen
#define PROGMEM
#define pgm_read_word(p) (*(p))
#define PRIPSTR "%s"
#endif
#endif // __RF24_CONFIG_H__
// vim:ai:cin:sts=2 sw=2 ft=cpp
****TembooAccount.h****
#define TEMBOO_ACCOUNT "" // your Temboo account name
#define TEMBOO_APP_KEY_NAME "" // your Temboo app key name
#define TEMBOO_APP_KEY "" // your Temboo app keyblink
****
Once all the code is loaded, connect the arduino YUN to a power supply and internet, open the serial monitor, give it a few moments to connect and the top tweet from the chosen feed should appear.
Step 2: Building the Reciever
Building the reciever is relatively easy as well, again, wiring the NRF24L01 is the tricky bit. Please follow the table below and the diagram of the NRF24L01 :)
NRF24L01: Function: Nano Pin
1: GND: GND
2: VCC: 3.5v
3: CE: D9
4: CSN: D10
5: SCK: D13
6: MOSI: D11
7: MISO: D12
8: IRQ:
please find the code below and in the attached file, just copy and paste into the arduino IDE:
#include
#include
#include
#include
#include
#include
#include
#include
#include
#define OLED_RESET 4
Adafruit_SSD1306 display(OLED_RESET);
#define NUMFLAKES 10
#define XPOS 0
#define YPOS 1
#define DELTAY 2
#define LOGO16_GLCD_HEIGHT 16
#define LOGO16_GLCD_WIDTH 16
#if (SSD1306_LCDHEIGHT != 64)
#error("Height incorrect, please fix Adafruit_SSD1306.h!");
#endif
int msg[1];
RF24 radio(9,10);
const uint64_t pipe = 0xE8E8F0F0E1LL;
int lastmsg = 1;
String theMessage;
//String theMessage1 = "";
void setup(void){
Serial.begin(9600);
radio.begin();
radio.openReadingPipe(1,pipe);
radio.startListening();
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3D (for the 128x64)
// init done
// Show image buffer on the display hardware.
// Since the buffer is intialized with an Adafruit splashscreen
// internally, this will display the splashscreen.
display.display();
delay(2000);
display.clearDisplay();
//testscrolltext(theMessage);
//delay(2000);
//display.clearDisplay();
}
void loop(void){
if (radio.available()){
bool done = false;
done = radio.read(msg, 1);
char theChar = msg[0];
if (msg[0] != 2){
theMessage.concat(theChar);
}
else {
int messageSize = theMessage.length();
Serial.println(messageSize);
Serial.println(theMessage);
//testscrolltext(theMessage);
//delay(5000);
display.display();
display.clearDisplay();
//theMessage= "";
//watchdogSetup();
String theMessage1 = theMessage.substring(0, (messageSize/4));
display.setTextSize(2);
display.setTextColor(WHITE);
display.setCursor(0,0);
display.println(theMessage1);
display.display();
delay(5000);
String theMessage2 = theMessage.substring((messageSize/4), (messageSize/2));
display.clearDisplay();
display.setCursor(0,0);
display.println(theMessage2);
display.display();
delay(5000);
String theMessage3 = theMessage.substring((messageSize/2), ((messageSize/4)*3));
display.clearDisplay();
display.setCursor(0,0);
display.println(theMessage3);
display.display();
delay(5000);
String theMessage4 = theMessage.substring(((messageSize/4)*3), messageSize);
display.clearDisplay();
display.setCursor(0,0);
display.println(theMessage4);
display.display();
delay(5000);
Serial.println(theMessage1);
Serial.println(theMessage2);
Serial.println(theMessage3);
Serial.println(theMessage4);
display.clearDisplay();
theMessage = "";
}
}
}
Once all connected and checked to be working the band was 3D printed on a DaVinci Jr using PLA. File attached. The components were held in place with hot glue.
Any questions, please ask :)
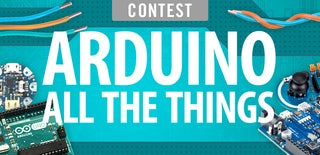
Participated in the
Arduino All The Things! Contest