Introduction: Using an LCD Screen With an Arduino 101
This is the eighth module from the Arduino 101 Lesson series.
The LCD Screen is a useful interface to see the outputs of various sensors connected to the Arduino. It is more convenient to use the LCD screen as opposed to the serial monitor for logging since the Arduino would be able to function as a standalone device provided it has a power supply. It is also very easy to code as the Arduino library supports it, although it is relatively harder to wire.
Step 1: Tools and Materials
- Arduino 101 or Arduino Uno
- Breadboard
- LCD Display
- Potentiometer
- Jumper Cables
Step 2: Circuitry Wiring
Connecting the power from the Arduino to the breadboard
- Connect the 3.3V pin from the Arduino to the breadboard using a red jumper cable.
- Connect one of the GND pins from the Arduino to the breadboard using a black jumper cable.
Adding a potentiometer to the circuit to control the brightness of the display
- Connect one of the two outer pins to the red power rail of the breadboard using a red jumper cable.
- Connect the other outer pin tot he black rail of the breadboard with a black jumper cable.
Wiring the LCD Screen on the breadboard
- It is easiest to wire the LCD screen in order of the pins so I recommend that the edge pin of the LCD screen to be plugged in on the very bottom of the breadboard.
- It is simpler and more straightforward to follow the schematic above. First, connect all the red pins from the LCD screen to the red power rail using orange jumper cables
- Then, connect the ground pins to the black power rail using brown jumper cables.
- Connect the 4th pin to the potentiometer's middle pin.
- Finally, connect the last few pins to the Arduino in the same consecutive order as the LCD screen.
Step 3: Coding
Coding the LCD is quite simple as mentioned before, due to the built-in libraries created by Arduino.
// built in arduino library
#include
LiquidCrystal lcd(12,11,5,4,3,2);
void setup() { // The LiquidCrystal library can be used with many different // LCD sizes. We're using one that's 2 lines of 16 characters, // so we'll inform the library of that:
lcd.begin(16, 2);
// clear old screen data lcd.clear();
// text to be dispalyed on the screen lcd.print("hello, world!");
}
void loop() {
// Here we'll set the invisible cursor to the first column // (column 0) of the second line (line 1):
lcd.setCursor(0,1);
// Now we'll print the number of seconds (millis() / 1000) // since the Arduino last reset:
lcd.print(millis()/1000);
}
Step 4: Demo Program
The screen will output "Hello, world!" and on the line below it will start counting upwards incrementally.
You can vary the message by changing lcd.print("hello, world!");
For example, lcd.print(" Peel the Avocado ");
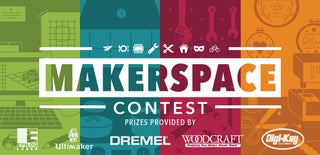
Participated in the
Makerspace Contest 2017