Introduction: Wireless Nunchuk Controlled Animatronic Doll
This instructable will attempt to show you how to make an animatronic doll controlled by a wireless nunchuk. This doll can only move its head though. I'm sure there are plenty of people out there who can take this and expand on it and make it much better, and I encourage that completely. :)
This instructable will focus more on the coding and wiring than on the building. Since the doll you use for this project will play a major role in how you mount and house the components and the doll you use most likely will not be the same one I used, the exact way I mounted the components may not work for you.
Step 1: Main Materials
1. Arduino Duemilanove Board
NOTE: I origianlly tried to do this with an Arduino Uno but the code wouldn't work for the newer version. You also have to use the arduino 18 software to compile and load the code
2. Bread board for test wiring
3. universal blank circuit board
4. servo, this one is a pan/tilt
5. wireless Kama nunchuk
NOTE: I know that this code works for this brand of wireless chuk. I cannot say for sure if other brands will work. The code I used was based on code written specifically for this brand of chuk.
6. Nunchuk extender cable
NOTE: This is what i used to connect the nunchuk to the Arduino, there are adapters you can buy that will get the job done as well. I'm not sure if all the wiring will be the same though
7. soldering Iron and solder
8. doll
NOTE: I got mine from Hobby Lobby, the limbs and head are plastic and the body is cloth
9. power switch
10. momentary switch use for reset
11. small cardboard box to house Arduino and circuit board
12. 3" PVC pipe cut to fit in doll
13. battery, this one is 7.2 Volts
14. backpack
NOTE: not necessary, used to hold extra components and the nunchuk
15. small speaker
NOTE: used with the wireless microphone to provide a way to make the doll "talk"
16. wireless microphone
17. wall wort
NOTE: needed to cut off the end to attach to the battery to be able to power the arduino with it, must be center positive
18. RC 7.2V battery connector repair kit
NOTE: used to connect to the battery so that we can splice this cable instead of the actual battery cable
19. wire for soldering to circuit board
20. various tools including screw driver, screws, drill, small saw, wrie cutters, wire strippers, electrical tape, bracket, wire, needle nose pliers. etc.
21. needle, thread, scissors, cloth (I used an old pillow case)
22. length of chain, length of material that looks like back pack straps
NOTE: optional to make back pack and add chains to pants
Step 2: Nunchuk Extender Cable Connection
This section is basically a repeat of parts of another instructable, some photos have been taken from said instructable. See link below:
https://www.instructables.com/id/Wireless-Wii-Nunchuck-control-of-Arduino-projects/step2/How-to-connect-these-wires-to-board/
1. cut off the male plug end of the cable
2. strip the white wire insulation to reveal the inner wires
3. expose the inner wires: my cable only had 5 colored wires and some strands of exposed wire wrapped in some white paper
4. Now that you have access to all the wires, strip a portion of each one to expose the wires inside
5. Only 4 of the wires are used. Look in the female end of the cable. There are 6 contact points: 3 on top, 3 on bottom. The outside 4 are used while the 2 middle ones are not. See diagram above.
6. Now you need to find out which wire is which contact point. To do this, you can use a multimeter set to measure resistance. Use one lead of the multimeter to make contact with one of the points in the female socket of the cable and use the other lead to make contact with the exposed portion of a colored wire. When you find the wire that coresponds to the contact point in the socket, the multimeter will read 0 ohms. See the video if you are confused.
7. when you have discovered which wires are which, label them so you wont forget. I cut off the unused wire to keep it out of the way.
8. you may want to solder the ends of the wires a little to make a stronger solid wire that is easier to plug into the Arduino than the thin splayed wires the way they are. Here is a link to a video on how to solder for those of you who are like me before this project and have never used a soldering iron:
http://www.youtube.com/watch?v=BLfXXRfRIzY
Be sure not use too much solder or the wire will be too thick to plug into the arduino
Step 3: Setting Up the Bread Board for Testing Wiring
In this step we will wire everything up to the breadboard and the arduino
1. attach jumper cables to the servos. you will need one for the power, ground, and control for each servo.
2. use the bread board to power the servos and the nunchuk. You can wire your bread board how ever works best for you, but I have provided my wiring set up for your reference. Here is a video on bread board basics for those of you new to this:
http://www.youtube.com/watch?v=q_Q5s9AhCR0&feature=related
3. wire the Arduino: this will include power and ground from the breadboard, SDA and SCK of the extender cable from the bread board, and the control jumpers from the servo.
4. go ahead and plug the nunchuk receiver into the extender cable
5.Once everything is wired you are ready to load the code and test it out
Step 4: The Code
1. connect the arduino to the computer
2. open the Arduino 18 program. If you dont already have it, you can download it from the Arduino webpage:
http://arduino.cc/en/Main/Software
3. make sure you have the correct board type selected and the correct usb port selected before continuing
4. copy and paste the code found below. This code was written using code from user bradlight on this forum and code from my instructor but I'm not sure who wrote it: http://www.arduino.cc/cgi-bin/yabb2/YaBB.pl?num=1259091426
5. compile the code
6. If there are no errors (there shouldn't be), upload the code to the arduino
7. when the code loads you should now be able to control the servo by tilting the nunchuck left, right, up, or down. If the servo is not responding, hold the reset button, sync the nunchuk, then release the reset button. I have found that the nunchuk must be synced before the code starts running or the servo gets stuck. This is why my design includes a momentary switch for a reset button.
Step 5: Solder to Circuit Board
The next step after the set-up passes testing is to solder it to a circuit board.
1. First thing you need to do is design a soldering plan. My plan is provided for you, but you can approach it however works best for you
2. next cut lengths of wire to use to solder to the circuit board and strip a portion of both ends
3. follow your plan and solder the wires to the board. You should have 6 wires coming from the board that will plug into the Arduino. This will requiring bridging. On the bread board, the rows were connected like you saw in the video, but on this board they are not, so any components that need to have a connection need to be bridged. This means that you use a line of solder to connect those components.
The second picture shows where the solder bridges go and the forth picture shows the bridges on the actual board.
Step 6: Reset Switch
Now make connectors for our reset switch.
1. Break off a small piece of unused blank circuit board
2. insert the momentary switch into the board and solder
3. cut a lengths of wire: one for power and one for ground and strip a portion of both ends of each
4. solder wires for power and ground to the board and bridge them to the switch leads. The diagram shows the layout I used. It doesnt matter which lead you use for positive and which for negative.
Step 7: Power Switch
Now lets get our power switch wired up.
1. the repair kit comes with 2 connectors, use the one that connects to your battery for this part of the instructable.
2. there is a red wire(power) and a black wire(ground). Cut the black one about half way down and strip both ends
3. cut 2 lengths of wire for soldering and strip both ends of each
4. wrap one length of wire around each of the connector wires as seen in the photos
5. solder each one
6. wrap the exposed connections in electrical tape
7. solder the other end of the wire lengths to the leads on the power switch, one wire for each lead.
8. now you should have the connector back together with the power button now spliced into the ground wire
Step 8: Power Adapter
Now we need to be able to use our battery to power the arduino so we need to make an adapter that can plug into the arduino.
1. You can buy the connector or you can just take an old wall wort that has a center positive plug and cut off the plug. I chose the second option.
2. Now that you have the plug cut off from the wall wort, strip the end to expose the wires
3. insert the ground wire into the ground connector on the battery connector and insert the power into the power on the battery connector, then pinch the connections closed with a pair of pliers
4. wrap the connection in electrical tape
5. the final product should look like the photo above.
Step 9: Connect It All to the Arduino
Now we can connect everything to the arduino and test our work.
1. Plug everything into the arduino. I have labeled the wires on the photo since the photo looks confusing even to me.
2. connect the battery and test the power switch
3. test the reset switch
4. try to control the servos, If movement left and right of the nunchuk makes the servo move up and down, your control wires are in the wrong pins. Just switch them around and test it again.
5. You can choose to solder the wires to the Arduino or just leave them plugged in as is. I did not solder mine, but a few of my wires were loose and kept coming unplugged. To fix this just bend a little of the wire back into itself and pinch it down with some pliers to make the wire thicker so it will stay plugged in better.
6. If all is right, move on to the next step.
Step 10: Housing the Boards
Now that all the wiring is set and in working order, it is time to house it to keep it safe when we put it inside the doll
1. First, since our circuit board is on top of our Arduino, we need to insulate them to keep them from touching and shorting out. A piece of sturdy plastic will do just fine. I used a cheap plastic binder.
2. cut a rectangle out of the binder to fit between the boards. You can use zip ties to keep the wires more orderly if you'd like
3. Prepare the box: the box I used came from Hobby Lobby and it was a tad bit too small to sit the arduino in flat so it has to go in at an angle. When the board is in the box, we need access to the power port and a place for the servo wires to go in and the reset button and nunchuk cable to come out. I orginally cut a small rectangle out of the front and a circle out of the lid but later found that I needed more room in the front to fit the nunchuk cable and power cable through. I also found that the way I mounted the components wouldnt allow for the servos to come through the top of the box, so another piece was cut away from the back of the box to run the servo wires. The photos here are of the project in progress so they show the set up before attempting to mount it in the doll.
4. once you have your housing ready, put the boards in and run all the necessary wires out of box and put the lid on to test the fit.
Step 11: Placing Switches
With the boards now housed, we need to work on mounting them in the doll. I used a 3" diameter PVC pipe cut to a length that matched the length of the doll's body to mount all the components to. First step is to mount the switches
1. decide where you want your switches to be
2. drill a hole of appropriate size in the pipe for each switch
3. mount the switches in the holes. The switches I bought are easy to mount because they have a peice to screw it on with.
Step 12: Mount the Box and Battery
Time to mount the box housing to the pipe
1. remove the lid and empty the housing box
2. decide where you want to mount the housing. Make sure the battery adapter that plugs into the arduino is at the top and the battery connector that plugs into the battery is at the bottom
3. screw the box to the pipe and place a piece of electrical tape over the screw inside the box
4, put the boards back in and run the wires out as you had before and replace the lid. You may want to tape the lid down to keep it secure. This should leave just enough room for the battery to slide in.
5. The battery needs to be kept from sliding out the bottom but also needs to be able to be removed for charging so make a latch of sorts to remedy the problem. I used a piece of wire cut from a wire clothes hanger and fashioned a latch.
6. screw the latch to the pipe. It should be able to turn to release the battery or to hold it in place.
Step 13: Mount Servos in the Head
1. cut the dolls head off the body. Leave as much fabric as possible on the sides where the arms connect.
2. Use a saw to cut open the scalp
3. Here is where it gets a little vague. You need to attach the servo to the head and run the wires down through the neck. The servos also need to be mounted to the pipe. I mounted the servo with the bar of the top servo facing the back of the head.
4. First place the servo in the head and run the wires down through the neck
5. now we need to make the connections to later mount the servo to the pipe before we can close the head and attach the servo. I used a piece of wire clothes hanger to fashion an arm of sorts. This arm was then attached to one side of the servo, ran down through the neck and will then be attached to the side of the pipe opposite the attachment to the servo. I have made a diagram to illustrate what I am trying to describe.
6. Now screw the scalp to the servo. This is a little tricky to line up but it holds it well.
7. When the servo moves, the flap we cut in the head will lift. To fix this, just screw on a bracket to hold it down.
8. Now line up the head and screw the wire to the pipe. The wire will want to move when the servo does so use a washer to make the attachment firmer.
Step 14: Making It Look Like a Doll
So now we have it all mounted and working. Next we need to make it look like a doll so we really should give it arms and legs to start with.
1. we need to extend the fabric around the head so that it extends into the clothing when we dress him. Cut a strip of fabric (I used an old pillow case) and sew it onto the existing material
2. the pipe is slightly too wide to fit in the body conveniently and the ball joints of the legs are too close together so they prevent the pipe from sitting flat. So we need to widen the doll's body. First cut the body in half vertically.
3. now cut another strip of fabric and sew it onto one half of the body to add some width. I sewed the strip to the front of the body since I didnt want to bother with the velcro on the back. Leave the bottom open (the butt) to allow easy access to the battery
4. sew the two halves of the body back together, connecting the added fabric to the other half of the body.
5. wrap the body around the pipe and pull the arms up to the correct position. My body was still slightly too narrow to velcro so I just taped the ends together which also holds the body in place against the pipe.
6. dress the doll. All the clothes should fit around the pipe perfectly. I sewed chains to my doll's pants to deck him out but thats completely optional of course. It is a little difficult to get the pants up around the pipe but they will fit with no alterations.
Step 15: Backpack
I gave my doll a little back pack to hold the speaker and microphone receiver as well as the nunchuk when not in use.
1. Unfortunatly, I couldnt find a ready made back pack that would work. All were too big or too small for the doll so I bought a coin purse thing from Hot Topic.
2. Then I bought some material from Hobby Lobby that looked like back pack straps and sewed them on the purse to make it into a back pack.
3. put the back pack on the doll and place the components inside.
Step 16: Credits
My step-dad Roberto did the mounting. He handled all the tools and came up with the mounting layout. He fashioned all the peices of the clothes hanger...which took alot of time and trial and error,and mounted the switches, the box housing the boards, and the servos. THANK YOU for spending your whole weekend on this.
My friend from class, Willie for helping me with the code, wiring, solder planning, and majority of the soldering. He also gave me a great deal on the battery and charger. THANKS!
My friend Robert who spent a good deal of time helping me work the code to finally get it working. THANKS
My instructor, Thomas who assigned this project and helped me through working with the frustrating code problems and various other problems. THANKS for your time and knowledge
and to everyone who wrote the codes I used to make mine, wrote instructables that helped me with mine, made the videos on you tube I used, instructables members, mainly thegeeke who answered my questions and gave me suggestions in the begining and everyone who listened to my rants when it got frustrating.
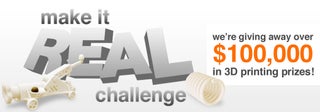
Participated in the
Make It Real Challenge
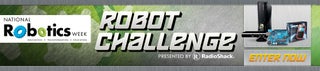
Participated in the
Robot Challenge
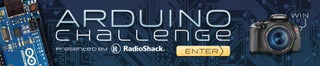
Participated in the
Arduino Challenge
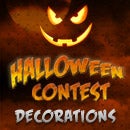
Participated in the
Halloween Decorations Contest