Introduction: Arduino Obstacle Avoiding Toy Car
This instructable will show you how to change a simple toy car into one with obstacle avoidance capabilities.
Since every toy car is different you might encounter different problems but also different possibilities.I will post different toy cars in the coming weeks to show variations.
Supplies
What you need (Hard ware):
-Arduino Uno or Nano board
-motor driver,this can be the L298N,L293D motor shield,L9110 or another type.Here I use the L9110.
-HC-SR04 sensor
-DuPont jumper cables female-male
-2 x DC motors
-2 x battery holders 9V
What you need(Tools):
-screwdriver
-soldering iron
-hot glue gun
-nuts and bolts
Step 1: Prepare Your Car and Attach the Motors and the Sensor:the Chop Shop
It is time to look at your car and decide how you are going to place the motors .In my example I choose to have the motors in the rear.So the rear axle has to be removed .A Dremel rotary tool is a good thing to have for this job.I decided to reuse the wheels(cut away the axle and drill holes in the middle of the wheel),this is not always an option you have.
For motors I use two gear motors with long shafts which is a handy thing to have.Not so handy,however,is that these shafts can slide inside the motor(they won't come out all the way though).The motors are bolted on the chassis.
After this was done I had to cut away the seats from the body to make the car fit together again.
The sensor will go in the front (obviously).The best fit was with the bumper removed,then some part of the chassis had to be cut away .I Attached the jumper cables on the servo,then screwed the body on the chassis again.The sensor is hotglued on the front and you will see it is pointed slightly upward.
Step 2: Give Your Car a Brain and Some Food
For my motor driver I used a L9110 module,this is a very compact one.Useful to have when there is not much space in your car.For some reason I was not able to get this to work with an Arduino Nano board,that would have been better in this case.
In my example the connections are like this:
sensor:
Trig:Arduino pin A1
Echo:Arduino pin A2
VCC:Arduino 5V
GND:Arduino GND
L9110:
B-1A : Arduino pin 4
B-1B: Arduino pin 5
GND: Arduino GND
VCC: to 9V battery +
A-1A: Arduino pin 6
A-1B: Arduino pin 7
My car has 2 9V batteries.One for the Arduino(but it will work with less also),one for the motors .
The battery for the motors is connected with the negative to the Arduino GND and the positive to the L9110 VCC.It is housed in a holder with an off/on switch(recommended or use a separate switch instead).
Both the batteries are located on the back of the car attached to the roll bar with an elastic band.Part of the roll bar had to be cut away to make this work.
Step 3: The Code
This is the code I used(not written by me,just changed where necessary).I found it here :
/* Obstacle Avoiding Robot Using Ultrasonic Sensor and Arduino NANO * Circuit Digest(www.circuitdigest.com) */
int trigPin = A1; // trig pin of HC-SR04
int echoPin = A2; // Echo pin of HC-SR04
int revleft7 = 7; //REVerse motion of Left motor
int fwdleft6 = 6; //ForWarD motion of Left motor
int revright4 = 4; //REVerse motion of Right motor
int fwdright5 = 5; //ForWarD motion of Right motor
long duration, distance;
void setup() {
delay(random(500,2000)); // delay for random time
Serial.begin(9600);
pinMode(revleft7, OUTPUT); // set Motor pins as output
pinMode(fwdleft6, OUTPUT);
pinMode(revright4, OUTPUT);
pinMode(fwdright5, OUTPUT);
pinMode(trigPin, OUTPUT); // set trig pin as output
pinMode(echoPin, INPUT); //set echo pin as input to capture reflected waves
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH); // send waves for 10 us
delayMicroseconds(10);
duration = pulseIn(echoPin, HIGH); // receive reflected waves
distance = duration / 58.2; // convert to distance
delay(10); // If you dont get proper movements of your robot then alter the pin numbers
if (distance > 19)
{
digitalWrite(fwdright5, HIGH); // move forward
digitalWrite(revright4, LOW);
digitalWrite(fwdleft6, HIGH);
digitalWrite(revleft7, LOW);
}
if (distance < 30)
{
digitalWrite(fwdright5, LOW); //Stop
digitalWrite(revright4, LOW);
digitalWrite(fwdleft6, LOW);
digitalWrite(revleft7, LOW);
delay(500);
digitalWrite(fwdright5, LOW); //movebackward
digitalWrite(revright4, HIGH);
digitalWrite(fwdleft6, LOW);
digitalWrite(revleft7, HIGH);
delay(500);
digitalWrite(fwdright5, LOW); //Stop
digitalWrite(revright4, LOW);
digitalWrite(fwdleft6, LOW);
digitalWrite(revleft7, LOW);
delay(100);
digitalWrite(fwdright5, HIGH);
digitalWrite(revright4, LOW);
digitalWrite(revleft7, LOW);
digitalWrite(fwdleft6, LOW);
delay(500);
}
}
Step 4: Conclusion
This was a fun project to do and not difficult at all.Improvements would be to change the 9V battery for the motors for LiPo batteries.Also the sensor is a bit tilted too much upwards.Keeping the orginal wheels means that the car sits very low with hardly any space between the bottom and the floor.Definitely a car for hard floors.
I will post more instructables in the future with different toycars.Hope this one gave people some inspiration.
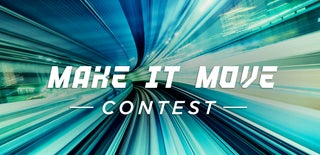
Participated in the
Make it Move