Introduction: Card Game Simulator: WAR!
At the family gathering for Thanksgiving this year, I watched 2 of my nephews pull out a deck of cards and start playing “War”. The game went on and on, and they eventually abandoned the game when dessert time rolled around. I started wondering if anyone had ever finished a game of War. If the game actually produced a winner, how many turns would it take?
Could I create a program to simulate a game of War? Could I run that simulation 10,000 times and get some numbers about the average number of turns in a game? Could I do this in about an hour of coding? Let’s see!
Step 1: Game Play
How does the game work? The deck is divided evenly, with each player receiving 26 cards, dealt one at a time, face down. Each player places their stack of cards face down, in front of them. Each player turns up a card at the same time and the player with the higher card takes both cards and puts them, face down, on the bottom of his stack.
If the cards are the same rank, it is War. Each player turns up one card face down and one card face up. The player with the higher turned-up card takes both piles (6 cards). If the turned-up cards are again the same rank, each player places another card face down and turns another card face up. The player with the higher card takes all 10 cards, and so on.
The game ends when one player has won all the cards.
Step 2: The Hour of Coding
Let’s code it up! I used VB.net as it is the language I use for work.
The first requirement is a “deck” of “cards”. We don’t need to worry about the suit in this game, just the values of the cards. Since there are 13 values, we can represent the deck as a list of integers, from 1 to 13, with each value repeated 4 times. Run this list through a function to randomize the order of the cards and we have a shuffled deck.
The next requirement is to create 2 “hands” for the players, a container that we can add cards to the bottom and take cards from the top. The obvious answer is a queue. The hands are dealt by looping over the shuffled list and alternately adding a value to each player’s queue. Now we are ready to actually play a game of War.
Because I was interested in the number of turns it takes to complete a game, I created the game as a function that returns a value for the turns required. I created variables for Minimum Turns, Maximum Turns, and the number of games to play. I could run 1 game or 1 million games.
Every turn of the game, a card is popped from each player’s hand and the values are compared. The player with the highest card has the 2 cards pushed onto their queue at the bottom. WAR! If the values are equal, the 2 equal cards are added to a list that represents the pot. The pot also adds another card popped from each player’s hand. Then one more card is popped from each player and the values are compared. The player with the highest card wins the pot and adds the 4 cards in the pot and the last 2 cards. The war loop happens until a player wins the pot or a player is out of cards, then the game moves onto the next turn. The game loop continues until one player is out of cards, then returns the number of turns to the calling function.
Coding the game only took me about an hour of coding (yay!), but I took another hour to add a log to view how a game actually progressed.
Attachments
Step 3: Results
The program can run 10,000 games in about 3 seconds, and 100,000 games in a little over 30 seconds. Taking a couple of minutes and running a bunch of simulations gives us an answer to the original question:
Min = 19
Avg = 272.31329
Max = 2662
100000 games played in 36 sec
******************************************
Min = 18
Avg = 272.10613
Max = 2613
100000 games played in 36 sec
******************************************
Min = 19
Avg = 272.52653
Max = 2656
100000 games played in 36 sec
******************************************
The average game takes 272 turns to complete, but it could be done in as few as 18 or 19. A single game could take more than 2,600 turns! No wonder you rarely see a finished game of War.
The chart shows what an average length game looks like graphically. What does a short game of War look like, turn by turn?
Turn 0001 - A:02 - B:11 - B takes the cards - 25 to 27
Turn 0002 - A:09 - B:02 - A takes the cards - 26 to 26
Turn 0003 - A:12 - B:02 - A takes the cards - 27 to 25
Turn 0004 - A:12 - B:13 - B takes the cards - 26 to 26
Turn 0005 - A:12 - B:12 - time to go to war - 25 to 25
Turn 0005 - A:06 - B:08 - B wins the war and adds 6 - 23 to 29
Turn 0006 - A:05 - B:06 - B takes the cards - 22 to 30
Turn 0007 - A:05 - B:05 - time to go to war - 21 to 29
Turn 0007 - A:11 - B:11 - time to go to war - 19 to 27
Turn 0007 - A:08 - B:08 - time to go to war - 17 to 25
Turn 0007 - A:04 - B:09 - B wins the war and adds 14 - 15 to 37
Turn 0008 - A:09 - B:10 - B takes the cards - 14 to 38
Turn 0009 - A:10 - B:05 - A takes the cards - 15 to 37
Turn 0010 - A:07 - B:10 - B takes the cards - 14 to 38
Turn 0011 - A:01 - B:07 - B takes the cards - 13 to 39
Turn 0012 - A:04 - B:04 - time to go to war - 12 to 38
Turn 0012 - A:03 - B:07 - B wins the war and adds 6 - 10 to 42
Turn 0013 - A:11 - B:01 - A takes the cards - 11 to 41
Turn 0014 - A:01 - B:01 - time to go to war - 10 to 40
Turn 0014 - A:13 - B:13 - time to go to war - 8 to 38
Turn 0014 - A:02 - B:02 - time to go to war - 6 to 36
Turn 0014 - A:02 - B:12 - B wins the war and adds 14 - 4 to 48
Turn 0015 - A:10 - B:12 - B takes the cards - 3 to 49
Turn 0016 - A:05 - B:12 - B takes the cards - 2 to 50
Turn 0017 - A:11 - B:13 - B takes the cards - 1 to 51
Turn 0018 - A:01 - B:03 - B takes the cards - 0 to 52
B wins the game!
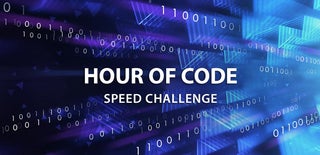
Participated in the
Hour of Code Speed Challenge