Introduction: Digital Notice Board Using Raspberry Pi and MQTT Protocol
Notice Boards are almost used everywhere, such as office, schools, hospitals, and hotels. They can be used over and over again to display important notices or advertise forthcoming events or meetings. But the notice or advertisements have to be printed on a paper and pinned on the notice boards.
In this Instructable let's learn to build our Digital Notice Board with Raspberry Pi to save lots of papers and toner!
Step 1: How Does Digital Notice Board Works?
- A Raspberry Pi connected to an HDMI Display which is our Digital Notice Board.
- A Windows PC is used to Publish the notice on the Digital Notice Board through the internet.
- The notice published by the Windows PC is received by the Digital Notice Board through a CloudMQTT broker.
- The communication between the Windows PC and the Digital Notice Board is achieved by the MQTT protocol.
Step 2: Things Required:
- Raspberry Pi with Rasbian OS
- Display with HDMI port
- Windows PC
- Internet Connection
- CloudMQTT account
Step 3: GUI Design for Displaying Notices:
We have to design 2 GUIs, one for Raspberry Pi to display the notice on the HDMI Display and another for Windows PC to publish the notice to Raspberry Pi via CloudMQTT broker.
The GUI design depends upon the place where you are going to place the Digital Notice Board. For example, let me design a GUI for Instructables Office to display forthcoming events and meetings so that the employees can be updated with the latest information.
It is easy to design a GUI in a Windows PC, so let us design the Digital Notice Board GUI in the Windows PC and copy the code to the Raspberry Pi.
Software required:
Anaconda (which includes python 2.7, Qt Designer package and Spyder IDE).
Qt Designer is the tool used to design GUIs. The output of the Qt Designer will be a .ui file, later it can be converted to .py for further process.
What is happening in the video?:
- Download Anaconda Windows Installer for python 2.7 and install it in a Windows PC(normal installation process).
- After installation, you can find the Qt Designer tool in "installation_directory\Library\bin\designer.exe"(for me it is "C:\Anaconda2\Library\bin\designer.exe")
- Create a shortcut for "designer.exe" and place it on the desktop.
- open "designer.exe".
- Create a new Main Window.
- Pick and place the layouts and the required views(text view, label view, etc).
- Save as Rpi_UI.ui file.
- To convert it into .py file open cmd prompt in the current folder where the Rpi_UI.ui file exists and type the following command
installation_directory\Library\bin\pyuic5.bat -x RPi_UI.ui -o RPi_UI.py
for me it is,
C:\Anaconda2\Library\bin\pyuic5.bat -x RPi_UI.ui -o RPi_UI.py
this command will convert the Rpi_UI.ui file toRpi_UI.py file and place it in the same directory.
- Open the Rpi_UI.py file with Spyder IDE which is included in Anaconda.
- Running the script will display the GUI we designed earlier.
Next, let us set up the CloudMQTT account.
Step 4: Set Up a CloudMQTT Account:
- Visit this link .
- Create an account with E-mail and log in your account.
- Create new instance (I named it as TEST_1).
- Open the Instance info.
- Make note of the Server, User, Password, and Port.
- Refer CloudMQTT Python Documentation and save the script as CloudMQTT.py.
- The example code in the documentation requires paho library, Install Paho Python Client using pip tool, open cmd prompt as administrator and enter the following command.
pip install paho-mqtt
Step 5: Raspberry Pi Final Code Explained:
Here, let me explain the way I combined RPi_UI.py file with the CloudMQTT.py and saved it as RPi_UI.py.
- Import libraries, if it is not installed just install it.
import paho.mqtt.client as mqtt import urlparse from PyQt5 import QtGui, QtWidgets, QtCore from PyQt5.QtCore import QTimer, QTime from threading import Thread import sys import re from google_drive_downloader import GoogleDriveDownloader as gdd import os
- To install googledrivedownloader, use the command
pip install googledrivedownloader
- Initializing variables,
icon = "instructables-logo@2x.png" contestImg = "black" meeting1 = "Meeting1:" venue1 = "Time and venue1." meeting2 = "Meeting2:" venue2 = "Time and venue2." meeting3 = "Meeting3:" venue3 = "Time and venue3."
- Followed by the class Ui_MainWindow
class Ui_MainWindow(object): def setupUi(self, MainWindow): ... def retranslateUi(self, MainWindow): ... def _update(self): ...<br>
- The following lines in the function setupUi update the GUI every 3 seconds by calling the _update function
self.retranslateUi(MainWindow) QtCore.QMetaObject.connectSlotsByName(MainWindow) self.timer = QTimer() self.timer.timeout.connect(self._update) self.timer.start(3000)
- The on_message function waits for the message from the broker, once the message is received it downloads the image from the google drive using the google drive sharable link ID and also changes the values of the global variables.
def on_message(client, obj, msg): print(str(msg.payload) ) if(str(msg.payload) ): noticeReceived = str(msg.payload) result = re.search('%1(.*)%2(.*)%3(.*)%4(.*)%5(.*)%6(.*)%7(.*)%8', noticeReceived) global contestImg global meeting1 global venue1 global meeting2 global venue2 global meeting3 global venue3 fileId = ""+result.group(1)+"" path = "/home/pi/Desktop/Instructables/RPi UI/ContestImages/"+result.group(1)+".jpg" gdd.download_file_from_google_drive(file_id = fileId, dest_path= path) contestImg = result.group(1) meeting1 = result.group(2) venue1 = result.group(3) meeting2 = result.group(4) venue2 = result.group(5) meeting3 = result.group(6) venue3 = result.group(7)
- The code consists of 2 infinite loops,
rc = mqttc.loop()
and
sys.exit(app.exec_())
In order to run these loops simultaneously, I have used the Threading concept
def sqImport(tId):<br> if tId == 0: while 1: rc = 0 while rc == 0: rc = mqttc.loop() print("rc: " + str(rc))if tId == 1: while 1: app = QtWidgets.QApplication(sys.argv) MainWindow = QtWidgets.QMainWindow() ui = Ui_MainWindow() ui.setupUi(MainWindow) MainWindow.show() sys.exit(app.exec_()) threadA = Thread(target = sqImport, args=[0]) threadB = Thread(target = sqImport, args=[1]) threadA.start() threadB.start() threadA.join() threadB.join()
Cool, we have completed the Raspberry Pi set up, next let us design GUI for Windows PC to publish the message to the Raspberry Pi.
Attachments
Step 6: Windows PC GUI:
- Design a GUI for windows and save it as Windows_UI.ui.
- Convert it into a python file.
- Combine it with the CloudMQTT.py file and save it as Windows_UI.py.
- The function of Windows_UI.py file is similar to the RPi_UI.py file, the only difference is the Windows_UI.py file publishes the message whereas the RPi_UI.py receives the message.
Step 7: Windows PC Final Code Explained:
- The Windows_UI.py file has all the classes and functions of RPi_UI.ui except a few.
- Instead of the on_message function, it has an on_publish function to publish the message.
- The following code within the retranslateUi function calls the publish function once the PUBLISH button is clicked.
self.pushButton.clicked.connect(self.publish)
- The publish function concatenates the google drive sharable link ID and the meeting information and publish it under the topic "notice".
- This message will be received by the Raspberry Pi.
Attachments
Step 8: Set Up the Digital Notice Board:
- Connect the Raspberry Pi to an HDMI display, I have used my Sony TV as the Digital Notice Board Display.
- Run the RPi_UI.py file in the Raspberry Pi.
- Run the Windows_UI.py file in the Windows PC.
- Enter the Google drive link ID of a contest image and the meeting announcement.
- Click the PUBLISH button.
- Now you can see the updated Digital Notice Board within a few seconds.
Hints:
- You can create the desired number of Digital Notice Boards and the Boards can be subscribed to different Topics.
- To make the Windows_UI.py file portable, you can convert the file into an executable file using pyinstaller, so that you can run the executable file in any Windows PC without installing the required libraries in the PC.
Thank you
SABARI KANNAN M
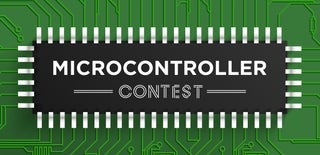
Participated in the
Microcontroller Contest