Introduction: Geiger Counter Triggered LED Decorations
Bored of blinky lights that blink in response to time?
Make them blink with space instead!
This instructable shows a quick method for connecting LED tree decorations to a Geiger counter via arduino, so that the lights blink between different strings each time radiation is detected.
It's nicer to think of this as "cosmic rays" than some of the other possibilities...
What you'll need:
- A Geiger counter with pulse output (I'm using the Gieger counter from MightyOhm)
- A few strings of battery-operated LED decorations (I'm using stars and snowflake decorations from Poundland, 'cos you gotta love dem luminous spheres of plasma and dem frozen supercooled cloud droplets)
- Arduino-based microcontroller (either a proper development board, or I'm using a stripboard bare bones version)
- Female header (two or three sockets' worth)
- Batteries/alternative power supply for your Geiger counter and Arduino
- Assortment of connecting wires (either jumper leads or multi-core stuff to solder)
- Soldering equipment, wire cutters, wire strippers etc.
- Maybe a spare LED for testing, insulation tape...
Step 1: The Geiger Counter
The PULSE connector (J6) has the following pinout:
1. VCC (nominally 3V)
2. pulse output – a short (100us) active high pulse every time the geiger tube fires
3. GND

We need to connect the GND pin (the one on the right) with a ground pin on our arduino and the pulse output (middle) with a pin on the arduino so we can detect the detections.
Cut 3 sockets' worth of female header. (You can get away with two if you have to.)
Cut yourself two lengths of wire long enough to reach between your Geiger counter and your arduino. It's helpful to have two different colours, but not essential. I'm something of a traditionalist and have used black for ground and red for the pulse output...
Solder the ground wire to one of the outer pins and the pulse wire to the middle pin.
This assembly should fit nicely onto the male pins on the Geiger counter:

Step 2: The Arduino
Now we need to connect the other end of those wires to the microcontroller.
I grabbed an old stripboard version I had lying around.
There are various instructions available online for making these, I remember referring to the Standalone Arduino / ATMega chip on breadboard instructable and the ITP Setting up an Arduino on a breadboard tutorial.
It was a lot of learning ago, so don't copy my wiring! If I were to start from scratch I'd do it like the schematic included in the images...
Connect the pulse output wire to pin 12 and ground to GND
Step 3: Test It
Now's a good time to check the arduino is reading the pulses from the Geiger counter...
I'm still learning the basics of coding. Mostly from Garry. He showed me how to do this bit!
Connect an LED between pin 13 and gnd of the microcontroller (if your board doesn't already have an integrated one).
Upload the following code to your microcontroller:
const int geigerPin = 12; // connected to pulse out of Geiger counter, grounds connected too
void setup() {
pinMode(geigerPin, INPUT);
}
int led = LOW;
void loop() {
int val = digitalRead(geigerPin);
while (val == LOW) {
// do what ever happens when there is no signal
val = digitalRead(geigerPin);
}
// got the start of a pulse, so do something appropriate
// toggle the LED so that you can see things working
digitalWrite(13, led);
led = !led;
while (val == HIGH) {
// wait for pulse to end, 100us is a long time for an Arduino
val = digitalRead(geigerPin);
}
// pulse finished
}
Power up the Geiger counter and the arduino, check they are connected properly, and you should see the LED toggle on or off each time the Geiger counter detects some radiation.
Assuming all is now working as it should, we're going to need MORE LEDS!
Step 4: The LEDs
I'm using three strings of cheap, battery-powered LED lights.
Chop the battery boxes off the ends of the strings and solder in any extension wire that may be necessary (have a think about where the Geiger counter and arduino will sit, and where you want your lights to be).
If you've added in extensions, make sure you insulate the joins with tape to prevent any short circuits later.
You won't need the battery box, so put it aside for when it Might Come In Useful.
Your LED strings will only light up if the ground and positive wires are connected the right way around. Do some quick tests with a battery if you need to and gather all the ground wires together. Attach them to a GND pin on your microcontroller. (I soldered them in one clump to a suitable track on the strip board.)
Connect one each of the positive wires to PWM pins 9, 10 and 11 of the arduino.
Here's the pin mapping if you're working with a stripboard version: http://www.arduino.cc/en/Hacking/PinMapping168
At this point you could try some test code to check the LED strings are all working ok...
Step 5: The Code
I attached the LEDs to PWM pins because I wanted to be able to control the brightness.
This code just does a straight cut between strings, but I'd like to add a fade or a cross-dissolve in later versions...
Upload the following code to your microcontroller:
const int geigerPin = 12; // connected to pulse out of Geiger counter, grounds connected too
int lights = 1; //counter for strings
int lights1 = 9; //define pins the LEDs are connected to
int lights2 = 10;
int lights3 = 11;
int bright1 = 200; //set brightness, 0-255
int bright2 = 200;
int bright3 = 200;
void setup() {
Serial.begin(9600); //Use serial for debugging
pinMode(geigerPin, INPUT); //set pins to inputs and outputs
pinMode(lights1, OUTPUT);
pinMode(lights2, OUTPUT);
pinMode(lights3, OUTPUT);
analogWrite(lights1, bright1); //switch on string 1
analogWrite(lights2, 0);
analogWrite(lights3, 0);
Serial.println("setup");
}
int led = LOW;
void loop() {
int val = digitalRead(geigerPin);
while (val == LOW) {
// do what ever happens when there is no signal
val = digitalRead(geigerPin);
}
// got the start of a pulse, so do something appropriate
// I'd toggle an LED so that you can see things working
digitalWrite(13, led);
led = !led;
Serial.println("LOW");
while (val == HIGH) {
// wait for pulse to end, 100us is a long time for an Arduino
val = digitalRead(geigerPin);
}
// pulse finished
lights ++; //increment the counter to move onto the next string
if (lights == 1){
analogWrite(lights1, bright1); //turn on string 1
analogWrite(lights2, 0);
analogWrite(lights3, 0);
Serial.println("lights1");
}
if (lights == 2){
analogWrite(lights1, 0);
analogWrite(lights2, bright2); //turn on string 2
analogWrite(lights3, 0);
Serial.println("lights2");
}
if (lights == 3){
analogWrite(lights1, 0);
analogWrite(lights2, 0);
analogWrite(lights3, bright3); //turn on string 3
Serial.println("lights3");
lights = 0; //reset the counter
}
Serial.println(lights);
}
Plug in, switch on, find a plant to decorate :)
Step 6: The Results
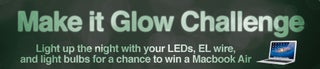
Runner Up in the
Make It Glow Challenge