Introduction: Low Cost Arduino Smart Doorbell
In this Instructables, I will show you how to create a low-cost smart doorbell for around $30 range. In this design, I use an Arduino Wemos with a built Wifi chip to so that the doorbell can communicate wirelessly with your smartphone. The following features are supported in this built. The doorbell current does not have live stream video.However, it capable of taking a picture when someone is at the door and send it to your phone with a datestamp. In addition, there is also motion detect feature in case there someone at the door but they did not press the doorbell.
Overall the following features are supported in this project:
- Alert when doorbell is pressed
- Motion detection
- Photo capturing
- Wireless Control
In the future, I hope to incorporate 10s video feature like Snapchat when someone is at the door or the doorbell is pressed.
Step 1: Materials
HC-SR505 Infrared PIR Motion Sensor $1
Push Button $2
SD Card Slot $2
3D Print Parts
Total Approximately : $34
Step 2: 3D Files
Download the files below and printed all of them out with around 30% thickness. Once you had all the files printed you can begin constructing the your very own smart doorbell.
Step 3: Download
The following files include .STL and .STP of all the mechanical part. Use winrar to unzip.
Attachments
Step 4:
- First, put the camera into the camera case
- Put the button into the button case
- The camera case should be a tight-fit for the camera and no screw is necessary
- For the button case, take a screw that fit the middle opening on the button module and screw it down to the case
- Make sure the the button is secure tightly on it's case
Step 5:
- Screw the both the two new module you just make (camera and button module) to the bottom side of the Top piece of the smart doorbell.
- The hole for the camera is the topmost hole and button is the bottom-most hole. Alternatively, you can just try each hole until they fit since each the camera can only fit one of the three holes.
- You probably need only around 3-4 screws for each module to make them secure.
- Make sure both modules is secure and move on to next step.
Step 6:
- This step we add the Motion Sensor into the back of the Top piece.
- The hole for the sensor is the middle hole. Simply push it in and make and make sure that it is secure. No need for a case for this module.
- You can also tape the board to the bottom of the camera module if you want to make it more secure.
Step 7:
Step 8:
- Attached the SD card slot to the Bottom piece as shown above.
- Make sure that the side with the pin is facing toward the wall with an opening.
- Secure the SD card slot with 2 screws.
Step 9:
- Now put the Arduino into the Bottom piece as shown above.
- Make sure that you connected the micro USB cable to the Arduino in this step.
- The micro USB cables should be going out of the opening on the wall.
- Secure the Arduino with one or two screws and it should be good enough
Step 10:
- Now put the Top piece on top of the Bottom piece.
- Secure it down with 4 screws on each corner as shown in the picture above.
Step 11:
- Now put on the 2 cover pieces
- The white pieces is on top .( top is the wall with opening)
- The black pieces go on bottom
- These pieces should be a tight fit and no screws is necessary.
Step 12: Software : Android App
This is an custom built android app that communicate directly with the webserver that the Arduino Wemost put out . The app simply pull data from that webserver whenever there something new is being update. In the app, you can see doorbell notification and log with date stamp. In you click on one of these log you can see the picture that being taken and some more information such as whether the door bell was pressed or not .
Step 13: Wiring
Arduino Wemos to Camera
- D1 -------- TX
- D0 -------- RX
- 5V -------- VCC
- GND------ GND
Note : Only use 4 pin, leave unsued pin alone
Arduino Wemos to SD Card Slot
- D13----------SCK
- D12----------MISO
- D11----------MOSI
- D10----------SS
- 5V -----------VCC
- GND--------GND
Arduino Wemos to Motion Sensor
- D2------------Out
- 5V--------------5V
- GND-----------GND
Arduino Wemos to Digital Button
- D12-----------Out
- 5V-------------5V
- GND----------GND
Step 14: Software : Arduino
In this section, I am just going briefly on how to interface the Arduino Wemos with the button, camera/sd card slot, and the motion sensor. This is simply a short tutorial that shown you how these components. In addition, I will also show you how to how to access wifi and transmit data to your android app using the Arduino Wemos.
Motion Sensor
The below is the code for motion sensor
/* * PIR sensor tester */ int inputPin = 16; // choose the input pin (for PIR sensor) int pirState = LOW; // we start, assuming no motion detected int val = 0; // variable for reading the pin status void setup() { pinMode(inputPin, INPUT); // declare sensor as input Serial.begin(9600); } void loop(){ val = digitalRead(inputPin); // read input value if (val == HIGH) { // check if the input is HIGH if (pirState == LOW) { // we have just turned on Serial.println("Motion detected!"); // We only want to print on the output change, not state pirState = HIGH; } } else { if (pirState == HIGH){ // we have just turned of Serial.println("Motion ended!"); // We only want to print on the output change, not state pirState = LOW; } } }
This Motion Sensor had a delay of 8 seconds so it will not be instantaneous when there is is not motion when a motion is just detected.
Step 15:
Below is simple code to interface with the button
/* *Button tester */ int inputPin = 12; // choose the input pin (for PIR sensor) int pirState = LOW; // we start, assuming no motion detected int val = 0; // variable for reading the pin status void setup() { pinMode(inputPin, INPUT); // declare sensor as input Serial.begin(9600); } void loop(){ val = digitalRead(inputPin); // read input value if (val == HIGH) { // check if the input is HIGH Serial.println("Button Pressed"); } }
This code simply printed out button pressed on the serial monitor when you pressed the button.
Step 16:
The code below interface with the camera , sd card slot and button. When the button is pressed the camera take a picture and save it onto the sd card slot.
#include <camera_VC0706.h> #include <SD.h> #include <SoftwareSerial.h> #define chipSelect 10 #if ARDUINO >= 100 SoftwareSerial cameraconnection = SoftwareSerial(2, 3); #else NewSoftSerial cameraconnection = NewSoftSerial(2, 3); #endif camera_VC0706 cam = camera_VC0706(&cameraconnection); void setup() { #if !defined(SOFTWARE_SPI) #if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__) if(chipSelect != 53) pinMode(53, OUTPUT); // SS on Mega #else if(chipSelect != 10) pinMode(10, OUTPUT); // SS on Uno, etc. #endif #endif pinMode(7,INPUT_PULLUP); Serial.begin(9600); Serial.println("VC0706 Camera test"); if (!SD.begin(chipSelect)) { Serial.println("Card failed, or not present"); return; } if (cam.begin()) { Serial.println("Camera Found:"); } else { Serial.println("No camera found?"); return; } char *reply = cam.getVersion(); if (reply == 0) { Serial.print("Failed to get version"); } else { Serial.println("-----------------"); Serial.print(reply); Serial.println("-----------------"); } cam.setImageSize(VC0706_640x480); uint8_t imgsize = cam.getImageSize(); Serial.print("Image size: "); if (imgsize == VC0706_640x480) Serial.println("640x480"); if (imgsize == VC0706_320x240) Serial.println("320x240"); if (imgsize == VC0706_160x120) Serial.println("160x120"); Serial.println("Get ready !"); } void loop() { if(digitalRead(7)== 0) { delay(10); if(digitalRead(7)== 0) { if (! cam.takePicture()) Serial.println("Failed to snap!"); else Serial.println("Picture taken!"); char filename[13]; strcpy(filename, "IMAGE00.JPG"); for (int i = 0; i < 100; i++) { filename[5] = '0' + i/10; filename[6] = '0' + i%10; // create if does not exist, do not open existing, write, sync after write if (! SD.exists(filename)) { break; } } File imgFile = SD.open(filename, FILE_WRITE); uint16_t jpglen = cam.frameLength(); Serial.print(jpglen, DEC); Serial.println(" byte image"); Serial.print("Writing image to "); Serial.print(filename); while (jpglen > 0) { uint8_t *buffer; uint8_t bytesToRead = min(32, jpglen); buffer = cam.readPicture(bytesToRead); imgFile.write(buffer, bytesToRead); jpglen -= bytesToRead; } imgFile.close(); Serial.println("...Done!"); cam.resumeVideo(); } } }
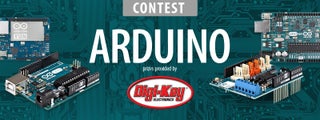
Participated in the
Arduino Contest 2016