Introduction: Metric Clock: Take 2
Some of you may remember my first instructable, the binary metric clock. It was published like a month ago, but I'm too lazy to actually check. After getting an LCD, I've decided to remake a better, easier to read, easier to set, useful version. For those who are not familiar with my first metric clock, I will include a small orientation:
It works like anything does in metric, with days as the base unit.
It can show decidays, centidays, millidays, and microdays.
For reference, 864 seconds are in a milliday.
Again for reference, six in the morning is 2:5:0:000, noon in 5:0:0:000, and 6 in the evening is 7:5:0:000.
I personally believe that a metric clock would simplify time-keeping, and should be use instead of the current system. A metric clock would make sense, as opposed to what is in place now.
Changes since the first version:
Not in binary
On an LCD
Can show microdays
Can be set without a computer
Step 1: Supplies
Here is the list of what you'll need:
1 LCD (must be compatible with Hitachi HD44780 driver)
1 10k potentiometer
2 buttons
2 10k resistors
1 Arduino (or clone)
Lots of wire
A breadboard
Step 2: Building It
The set up is pretty easy. If you take a look at the picture (created using Fritzing), the blue lines are wires, the big green thing is an LCD, and the resistors are 10,000 ohm resistors. It is remotely self-explanatory.
Step 3: Code & Explanation
Here is the code, commented for your convenience:
/*
Metric clock
By Alec Robinson, otherwise known as alecnotalex.
Licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 3.0 Unported License.
*/
#include <LiquidCrystal.h> //Used for writing for the LCD
int deci = 0, centi = 0, milli = 0, micro = 0; //Keep track of each of the units
int setmode = 0; //0 if user is not setting anything, 1 if user is setting decidays, 2 for centidays and so on.
int setbutton = 3, addone = 2; //Pins for buttons; setbutton changes setmode, addone changes the units the user is setting
LiquidCrystal lcd(7, 8, 9, 10, 11, 12); //Creates new lcd at pins 7-12
void setup ()
{
lcd.begin(16, 2); //Begins the 16x2 LCD
}
void loop ()
{
lcd.setCursor(0, 0);
lcd.print(deci); //Prints decidays
lcd.print(":");
lcd.print(centi); //Prints centidays
lcd.print(":");
lcd.print(milli); //Prints millidays
lcd.print(":");
lcd.print(micro); //Prints microdays
lcd.setCursor(0, 1);
if (digitalRead(setbutton) == HIGH) //If setbutton is pressed
{
setmode++; //Changes the mode
if (setmode == 4) //Resets it if the user has scrolled through all settings
{
setmode = 0;
lcd.print(" "); //Clears bottom row of screen
}
}
if (setmode > 0) //If the user is setting something
{
lcd.print("Setting: ");
delay(100); //To make sure the user doesn't accidently press a button more than once when they dont mean to
}
else
{
delay(86.4); //One microday
micro++;
if (micro == 1000) //One milliday
{
milli++;
micro = 0;
lcd.setCursor(7, 0);
lcd.print(" ");
lcd.setCursor(0, 1);
}
if (milli == 10) //One centiday
{
centi++;
milli = 0;
}
if (centi == 10) //One deciday
{
deci++;
centi = 0;
}
if (deci == 10) //One day
{
deci = 0;
}
}
if (setmode == 1) //When the user is setting the decidays
{
lcd.print("deci");
if (digitalRead(addone) == HIGH)
{
deci++;
if (deci == 10) deci = 0;
}
}
if (setmode == 2) //The user is setting centidays
{
lcd.print("centi");
if (digitalRead(addone) == HIGH)
{
centi++;
if (centi == 10) centi = 0;
}
}
if (setmode == 3) //The user is setting millidays
{
lcd.print("milli");
if (digitalRead(addone) == HIGH)
{
milli++;
if (milli == 10) milli = 0;
}
}
}
Attachments
Step 4: Determine Metric Time, From Reguar Time
I have changed my method since last time.
Let m = minutes in regular time
Let h = hours in regular time
Let mt = metric time
(h + m/60)/24 = mt
This will return a decimal. This decimal is the metric time.
Step 5: Your Finished Product
You're finished building your fancy new time keeping device! Your glorious new piece of time-telling equipment will greatly help you figure out how much of the day has gone by, and how much is left. Although currently no societies use a metric clock, one can still find many ways to use this device.
In the future, I have even more plans. Once I have the supplies, I plan on making it sync with the satellites, so it can be super precise. Of course, this will not be until a long time. Until then, I hope you enjoy your new clock!
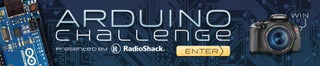
Participated in the
Arduino Challenge