Introduction: Missed Call Notifier
One of the first projects I had created when I started my journey into electronics was a missed call notifier (http://dushyant.ahuja.ws/2013/11/physical-android-notifier/). Unfortunately it used bluetooth and a workaround using Tasker, S4LA and Python to work, which stopped working some time about a year ago. I think probably due to an android upgrade. Don't know, didn't really bother to check again. However, I still wanted one, just to ensure that I see if there's any missed call, even if my phone is either in my bag, or in the other room. Recently I had bought a couple of ESP8266 modules (ESP-01) and thought this might be a good first project. Didn't need many GPIOs, and wifi would be easier to work than bluetooth (as Tasker can directly access web pages).
Step 1: Setting Up the ESP8266 Tool Chain
There is a lot of information on the internet to setup the ESP8266, however it is extremely fragmented. Also, I wanted to use the Arduino IDE instead of Lua, and that was slightly more difficult to find information on. I have collated all the information required to make this project a success and to ensure that everything needed is in one place.
Download Arduino IDE
Download the arduino IDE (any version greater than 1.6.4):
https://www.arduino.cc/en/Main/Software
Install the ESP8266 Board
- Start Arduino and open Preferences window.
- Enter
http://arduino.esp8266.com/stable/package_esp8266com_index.json
into Additional Board Manager URLs field. You can add multiple URLs, separating them with commas. - Open Boards Manager from Tools > Board menu and install esp8266 platform (and don't forget to select your ESP8266 board from Tools > Board menu after installation).
(Reference: https://github.com/esp8266/arduino)
Install the ESP8266Wifi Arduino Library (if it wasn't installed in the earlier step)
Step 2: Programming Circuit
Reference: http://www.esp8266.com/wiki/doku.php?id=esp8266-m...
The ESP8266 needs to be put into the boot-load mode before it can be programmed. Very easy - once you know how. Just connect as per the circuit diagram above.
(Reference: http://iot-playground.com/2-uncategorised/38-esp82...)
Ensure that CH_PD is connected to +3.3V and GPIO0 is connected to GND
DO NOT TRY TO CONNECT TO WIFI WHEN THE MODULE IS CONNECTED THROUGH THE USB-UART. The ESP8266 can pull a lot of power (upto 800mA) and can destroy your USB-UART module / laptop's PC. It is recommended to power the module separately and connect the ground from your power supply to the USB-UART.
Step 3: Code
Based on Code from
https://github.com/iot-playground/Arduino/blob/mas...
and
https://www.arduino.cc/en/Tutorial/BlinkWithoutDel...
/*
* This sketch demonstrates how to set up a simple HTTP-like server.* The server will set a GPIO pin depending on the request
* http://server_ip/gpio/0 will set the GPIO2 low,
* http://server_ip/gpio/1 will set the GPIO2 high
* server_ip is the IP address of the ESP8266 module, will be
* printed to Serial when the module is connected.
*/
#include <ESP8266WiFi.h>
const char* ssid = "your wifi";
const char* password = "your password";
// Create an instance of the server
// specify the port to listen on as an argument
WiFiServer server(80);
const int ledPin = 2; // the number of the LED pin
int val =0;
// Variables will change:
int ledState = LOW; // ledState used to set the LED
long previousMillis = 0; // will store last time LED was updated
// the follow variables is a long because the time, measured in miliseconds,
// will quickly become a bigger number than can be stored in an int.
long interval = 250; // interval at which to blink (milliseconds)
void setup() {
digitalWrite(ledPin, LOW);
Serial.begin(115200);
delay(10);
// prepare GPIO2
pinMode(ledPin, OUTPUT);
digitalWrite(2, 0);
// Connect to WiFi network
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
// Start the server
server.begin();
Serial.println("Server started");
// Print the IP address
Serial.println(WiFi.localIP());
}
void loop() {
// Check if a client has connected
unsigned long currentMillis = millis();
if( val==1){
if(currentMillis - previousMillis > interval) {
// save the last time you blinked the LED
previousMillis = currentMillis;
// if the LED is off turn it on and vice-versa:
if (ledState == LOW)
ledState = HIGH;
else
ledState = LOW;
// set the LED with the ledState of the variable:
digitalWrite(ledPin, ledState);
}
}
if( val == 0) digitalWrite(ledPin, LOW);
WiFiClient client = server.available();
if (!client) {
return;
}
// Wait until the client sends some data
Serial.println("new client");
while(!client.available()){
delay(1);
}
// Read the first line of the request
String req = client.readStringUntil('\r');
Serial.println(req);
client.flush();
// Match the request
if (req.indexOf("/gpio/0") != -1)
val = 0;
else if (req.indexOf("/gpio/1") != -1)
val = 1;
else {
Serial.println("invalid request");
client.stop();
return;
}
client.flush();
// Prepare the response
String s = "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n\r\n \r\nGPIO is now ";
s += (val)?"high":"low";
s += "
\n";
// Send the response to the client
client.print(s);
delay(1);
Serial.println("Client disonnected");
// The client will actually be disconnected
// when the function returns and 'client' object is detroyed
}
Attachments
Step 4: Actual Circuit
Step 5: Setting Up the Android Phone
Finding the IP Address
You can find the IP address of the module by connecting to your wifi router and finding the new device.
Alternatively, you can connect the USB-UART to the actual circuit (ensure that you connect the grounds together).
DO NOT CONNECT the 3V3 from the USB-UART to the circuit.
Once connected, open the Arduino Serial Monitor and restart the ESP8266. The module will diplay it's IP address once it is connected to the serial monitor.
Configure Tasker
The android phone connects to the module using Tasker (as I already had it installed), however you should be able to do something similar using IFTTT.
- Add Event -> Phone -> Missed Call
- New Task -> Add Action -> Net -> HTTP Get ->
- Server: :80
- Path: gpio/1
- Add Event -> Application -> Phone
- New Task -> Add Action -> Net -> HTTP Get ->
- Server: :80
- Path: gpio/0
- Once configured, the notifier will blink whenever you get a missed call, and will stop blinking when you open the phone application (presumably to see your phone log)
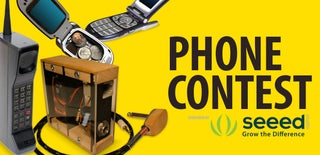
Participated in the
Phone Contest