Introduction: Email With Intel Edison (Intruder Alarm)
If you have a web-enabled device, it needs to do web enabled tricks! One of many things we do online frequently is use our email. Be it at work, on a tablet at the coffee shop, or straight to our smartphones, we almost always have access to email nowadays. Whether you need to send email to yourself, create an evil email-bot, or want to send reminders to a loved one, this instructable is for you.
In this tutorial, we are going to use node.js. Node.js is a scripting language based off of JavaScript and can do a lot of fun things fairly easily. There are a lot of resources online to help you learn the language if you aren't familiar, so I am not going to go too far in depth.
Prerequisites:
You've got yourself an Intel Edison, you've flashed it with the new firmware, and have set up the WiFi. Right? If not, you will need to do that first!!! https://communities.intel.com/docs/DOC-23147
Step 1: Edison Software Setup
While Intel provides an IDE called "Intel XDK IoT Edition" just for the Edison/Galileo, there is something to be said for doing things from scratch on a terminal. Of course, you can download and install the IDE, but it definitely isn't necessary.
To send emails, we need to install a package called NodeMailer. It has all the fun goodies we need to send emails. Open your Edison in a terminal like putty (Windows) or open a terminal and SSH into your Edison (Mac/Linux). You should have been walked through how to do that when you initially flashed and configured your Edison. Here's the link if you already forgot: https://communities.intel.com/docs/DOC-23147
A bit of a tip, whenever I tell you to type something into a terminal and press enter, you can also copy the text I have (minus the ">> " shown here and the LEFT CLICK in your terminal to paste it (then press enter because pressing enter is awesome).
To install NodeMailer, type the following into the terminal and press enter:
>> npm install nodemailer
If it turns out you don't have NPM installed (you'll get an error instead of amazing happiness of things working), you can install it by typing the following into the terminal and pressing enter. The go back, and try to install the NodeMailer packages again.
>> curl https://www.npmjs.org/install.sh | sh
Cool beans. You now have NodeMailer. Now we need forever.js. What forever.js will allow us to do is run our program even after we close the terminal. Otherwise, our program stop the second you close your terminal. The "-g" is VERY important as you cannot use forever as we intend to later if you don't.
>> npm install forever -g
Now, we want to install a program called Nano. Nano is a text editor that is extremely simple. If you know how to, you can also use Vi/Vim which is already a part of the Edison's Yocto image. While I LOVE Vi/Vim, it for some reason decided to stop accepting input on my Edison. To install Nano, type the following into your terminal and press enter. You will need to restart your Edison to complete the install.
>> wget http://www.nano-editor.org/dist/v2.2/nano-2.2.6.tar.gz && tar xvf nano-2.2.6.tar.gz && cd nano-2.2.6 && ./configure && make && make install >> make clean >> rm -r nano*
We want to make a directory for all our projects and then this project to stay organized. Type the following and press enter:
>> makedir mySandbox >> makedir mySandbox/intruderAlert
After installing our packages and software, we need to restart the Edison. Our little board tends to run out of RAM and restarting clears it out and cleans up. You might get lucky and find that you don't need to reboot, but I had to. Type the following and press enter:
>> reboot
Cool, now we have the general setup out of the way. Now we can write some code and add some circuits! Woot!
Step 2: Code
Now that you have everything setup, we can write some code. Let's jump into our sandbox folder and then our project folder and get started!
>> cd mySandbox/intruderAlert
>> nano -$ -i intruderAlert.js
Cool beans, now we write our code! When you are done, press control+o to save and then control+x to exit Nano. The text editor on instructables is buggy. If you are looking at the code and see a <br>, delete it and replace it with a newline (ie press enter or return).
//Set up our GPIO input for pin 5 on the Arduino breakout board var mraa = require('mraa'); var motionSensor = new mraa.Gpio(5); motionSensor.dir(mraa.DIR_IN); //Set up our node mailer var nodemailer = require("nodemailer"); var smtpTransport = nodemailer.createTransport{ service: "Gmail", auth: {
user: "example@gmail.com",
pass: "password"
} }); //Start sensing! periodicActivity(); //Here is our sensing function: function periodicActivity() { //Read our sensor var motionSensorTriggered = motionSensor.read(); //Do stuff if our sensor is read HIGH if(motionSensorTriggered){ //Send our email message smtpTransport.sendMail({ from: "John Doe <example@gmail.com>", to: "John Doe <example@gmail.com>", subject: "Possible Intruder Alert",
text: "Hey! Something activated your motion sensor!"
}, function(error, response){ //Send a report of the message to the console
if(error){
console.log(error);
}else{
console.log("Message sent: " + response.message); } smtpTransport.close();
});
//We don't want 5 million emails, so we want to wait a few seconds
//(in this case, 30 seconds) before sending another email. The timeout //is in milliseconds. So, for 1 minute, you would use 60000.
setTimeout(periodicActivity, 30000); }else{ //Our motion sensor wasn't triggered, so we don't need to wait as long. // 1/10 of a second seems about right and allows our Edison to do other // things in the background.
setTimeout(periodicActivity, 100);
} }
Step 3: Circuits
I am using a little PIR sensor I picked up at Radioshack a few years ago. You can also find them on Sparkfun, Adafruit, Amazon, and many other places for around $10. The way they work is they monitor the ambient infrared light in the room. When it abruptly changes, it sets an output on the sensor (which goes to an input on your Edison) high. Note that if you are not using an Edison Arduino break out board, you will likely need to level shift the output down as not to fry your Edison.
In a nutshell, you connect 5V on the board to to the positive terminal (+, VCC, PWR, or "5V") of the sensor, ground on the board (GND) to the negative terminal (-, VSS, GND, "0V"), and pin 5 on the board to the output of the sensor (OUT). Depending on which PIR sensor you are using, there may be colored wires that are presoldered or an unmarked circuit board - you will have to refer to your datasheet to find out where things go in that case.
You don't have to use a PIR sensor - you could make a laser tripwire, a weight sensor under a doormat, or buy IR tripwires (like what is connected to your garage door) as your sensor.
Step 4: Run the Code, Trip the Sensor, and Check Your Email!
To run the code, you have two options. The first is to run the program directly from the terminal. The disadvantage to this would be that once the terminal closes, your program stops running. This is also useful for testing purposes as you can quickly stop the program by pressing control+c should things get out of hand.
>> node intruderAlert.js
The better way to do this is to use the forever.js program we installed in the first step
>> forever start intruderAlert.js
Now your program is running into infinity. Great. Now EVERY TIME you walk in your house, you are going to get an email every 30 seconds. What are you going to do? There are a couple ways to address this. The first is to add an if statement to your code to only send emails during the day when no one is home. Another way to do this is to add a keypad to your Edison and have a passcode you enter to arm/disarm the system. The last and final way is to use your smartphone to log into your Edison and turn off the "alarm".
There are a multitude of terminal apps available for iOS and Android, so take your pick. You will need to find the wifi IP address of your Edison to set up your app. To do this, type the following into your computer's terminal (connected to the Edison) and press connect to the same wifi your Edison is on.
>> configure_edison -showWiFiIP
It should show you something like "192.168.0.30". This is the IP address you want to put into your smartphone's terminal app.
Now that you've logged into your Edison via wifi on your smartphone, type the following and press enter:
>> forever list
This will show you a list of all the node.js programs that forever is running. Look for the one called "intruderAlert.js" and take note of the job number (it will often be "0"). Now take that number (I'm using "0" in this case) and type the following and press enter:
>> forever stop 0
Step 5: Troubleshooting
So, you aren't getting any email when you were testing. Crap. We just went through ALL that for nothing. Well, let's see what we can do about that.
1) Are your credentials correct? Did you correctly type your email address and password? Did you use the same email in the "To:" section as you did in your credentials?
2) Are you sending to the right person's email address?
3) Is your wifi configured?
4) Are you using a Gmail account? You probably got an email telling you that a sign-in attempt was stopped. Don't fret, that was YOU trying to access your account! In the email, you will find the link https://www.google.com/settings/security/lesssecureapps. Go there and click "Enable". It may take several hours to overnight for this change to take place (it did in my case).
5) Did you type in the code right? caPitalizatiOn, spleling, [brackets], and pare{ntheses) matter. Make sure things are in the right order and are all done right. Note that I didn't copy and paste my code, I retyped it. So, if I made a mistake and you find it, shoot me a message and I'll fix it up for you :)
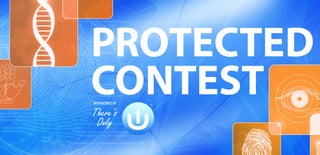
Participated in the
Protected Contest
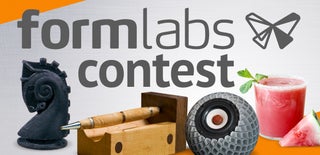
Participated in the
Formlabs Contest