Introduction: TTP224 Digital Touch Sensor Applications Using Arduino
TTP224 is a capacitive touch IC, you can convert your PCB into a touch Pad ! well this is useful if you want to replace a traditional Button , or you want to add a touch feature to your project.
TTP224 comes in a common breakout like in the picture, very cheap and so easy to integrate in your project.
with this breakout , you have 4 output pin (OUT1 - OUT4) , and normally the output is 0V "LOW" ,and once you touch the pad the signal rise to VCC "High".
The voltage supply VCC can be from 2.4V-5.5V so it's compatible with all Arduino boards 3.3V/5V .
In this tutorial I will show you how to use this breakout with some applications like :
- LCD I2C control panel
- Control Servo Motor
- Control Relay
Let's start with the components:
Step 1: Components
- Arduino Nano.
- LCD1602.
- I2C LCD Driver.TTP224 touch module.
- Servo motor SG90.
- Relay Module 2 Channel.
- Breadboard.
- Jumper Wires.
note : The LCD I2C library doesn't work on arduino 1.8.x for unknown reason , I tested the codes on arduino 1.5.5 , 1.6.1 and it works fine
Step 2: Simple Connection
First , I will connect the Arduino with TTP224 and read the touch pad status and print it on the LCD
this code is so simple and you can modify on it if you want to add other sensors.
the schematic in picture , I chose I2C lcd driver because it's easier to connect, you can use the LCD1602 without it but you need to change the connection.
the Arduino code :
<p>/*<br> * Simple TTP224 Touch pad reader. * //written by: Mohannad Rawashdeh-Raw Like and share this if you like it , and support me to make more if you have and suggestions , questions , follow me on: my website: <a href="https://mb-raw.blogspot.com"> https://mb-raw.blogspot.com </a> Facebook: <a href="https://www.facebook.com/Mohannad-Rawashdeh-Raw-774983565988641/"> https://mb-raw.blogspot.com </a> Twitter: <a href="https://twitter.com/MB_Rawashdeh"> https://mb-raw.blogspot.com </a> google+: <a href="https://plus.google.com/u/0/100553238092483773623"> https://mb-raw.blogspot.com </a> instructables:https://www.instructables.com/member/Mohannad%20Rawashdeh/?publicView=true * */ #include <wire.h> #include "LiquidCrystal_I2C.h"</wire.h></p><p>LiquidCrystal_I2C lcd(0x20,16,2); // set the LCD address to 0x20 for a 16 chars and 2 line display</p><p>const int TouchPin1=11; const int TouchPin2=10; const int TouchPin3=9 ; const int TouchPin4=8 ;</p><p>boolean TouchStatus1=false; boolean TouchStatus2=false; boolean TouchStatus3=false; boolean TouchStatus4=false; void setup() { lcd.init(); // initialize the lcd // Print a message to the LCD. pinMode(TouchPin1,INPUT); pinMode(TouchPin2,INPUT); pinMode(TouchPin3,INPUT); pinMode(TouchPin4,INPUT); lcd.backlight(); lcd.print("St 1 2 3 4"); }</p><p>void readTouch() { TouchStatus1=digitalRead(TouchPin1); TouchStatus2=digitalRead(TouchPin2); TouchStatus3=digitalRead(TouchPin3); TouchStatus4=digitalRead(TouchPin4); delay(10); } void printResult() { lcd.setCursor(3,1); lcd.print(TouchStatus1); lcd.setCursor(6,1); lcd.print(TouchStatus2); lcd.setCursor(9,1); lcd.print(TouchStatus3); lcd.setCursor(12,1); lcd.print(TouchStatus4); delay(50); } void loop() { readTouch(); printResult(); delay(100); }</p>
Attachments
Step 3: Control Servo Motor
In this code i will control servo motor and set the direction and the servo angle depend on the TTP224 status
If I touch:
1 - Move the servo CCW 2 degree , if the angle = 180 , do nothing
2- Move the servo CW 2 degree , if the angle = 0, do nothing
3 - Move the servo to 90 degree , if the angle >90 , do nothing
4 - Move the servo to 180 degree , if the angle >180, sure do nothing
You must take in consider the Power source issue here , the LCD need 160mA for the backlight , the servo need at lest 400mA so don't power them using arduino 5V pin , use power supply 5V -1A for better result , other wise the LCD will blinking , arduino will restart and it might cause a damage for the SMD fuse on arduino board.
code
<p>/*<br> * Control Servo motor using TTP224 * Touch pad and arduino. * //written by: Mohannad Rawashdeh-Raw Like and share this if you like it , and support me to make more if you have and suggestions , questions , follow me on: my website: <a href="https://mb-raw.blogspot.com"> https://mb-raw.blogspot.com </a> <p>Facebook: https://www.facebook.com/Mohannad-Rawashdeh-Raw-774983565988641/</p><p>Twitter: https://twitter.com/MB_Rawashdeh <br>google+: https://plus.google.com/u/0/100553238092483773623</p> instructables:https://www.instructables.com/member/Mohannad%20Rawashdeh/?publicView=true /* #include <Wire.h> #include "LiquidCrystal_I2C.h" #include <Servo.h> LiquidCrystal_I2C lcd(0x20,16,2); // set the LCD address to 0x20 for a 16 chars and 2 line display Servo SG90; // create servo object to control a servo </servo.h></wire.h></p><p>int degree=0;</p><p>const int TouchPin1=11; const int TouchPin2=10; const int TouchPin3=9 ; const int TouchPin4=8 ;</p><p>boolean TouchStatus1=false; boolean TouchStatus2=false; boolean TouchStatus3=false; boolean TouchStatus4=false; void setup() { lcd.init(); // initialize the lcd // Print a message to the LCD. lcd.backlight(); lcd.print("Servo Control"); SG90.attach(6); // attaches the servo on pin 9 to the servo object delay(2000); drawMainPanel(); SG90.write(degree); }</p><p>void readTouch() { TouchStatus1=digitalRead(TouchPin1); TouchStatus2=digitalRead(TouchPin2); TouchStatus3=digitalRead(TouchPin3); TouchStatus4=digitalRead(TouchPin4); delay(10); } void printResult() { lcd.setCursor(3,1); lcd.print(TouchStatus1); lcd.setCursor(6,1); lcd.print(TouchStatus2); lcd.setCursor(9,1); lcd.print(TouchStatus3); lcd.setCursor(12,1); lcd.print(TouchStatus4); delay(50); } void drawMainPanel() { lcd.clear(); lcd.print("1-Inc 2-Dec"); lcd.setCursor(0,1); lcd.print("3-90 4-180"); }</p><p>void controlServo() { if(TouchStatus1) { degree+=2; if(degree>=180){degree=180;} SG90.write(degree); } if(TouchStatus2) { degree-=2; if(degree<=0){degree=0;} SG90.write(degree); } if(TouchStatus3) { for(int i=degree;i<90;i++) { SG90.write(i); delay(40); } degree=90; } if(TouchStatus4) { for(int i=degree;i<180;i++) { SG90.write(i); delay(40); } degree=180; } delay(40); } void loop() { readTouch(); controlServo(); delay(100); }</p>
Attachments
Step 4: Control Relay
If you are looking for applications like control AC lamp or High voltage DC device you need a relay , so let's do something simple to control Relay 2 channel
Touch Pad 1 and 2 to control Relay #1
Touch Pad 3 and 4 to control Relay #2
<p>/*<br> * Control Servo motor using TTP224 * Touch pad and arduino. * //written by: Mohannad Rawashdeh-Raw Like and share this if you like it , and support me to make more if you have and suggestions , questions , follow me on: my website: https://mb-raw.blogspot.com Facebook: https://www.facebook.com/Mohannad-Rawashdeh-Raw-774983565988641/ Twitter: https://twitter.com/MB_Rawashdeh google+: https://plus.google.com/u/0/100553238092483773623 instructables:https://www.instructables.com/member/Mohannad%20Rawashdeh/?publicView=true * */</p><p>#include <wire.h> #include "LiquidCrystal_I2C.h" LiquidCrystal_I2C lcd(0x20,16,2); // set the LCD address to 0x20 for a 16 chars and 2 line display</wire.h></p><p>int degree=0;</p><p>const int TouchPin1=11; const int TouchPin2=10; const int TouchPin3=9 ; const int TouchPin4=8 ;</p><p>const int RelayPin1=4 ; const int RelayPin2=5 ;</p><p>boolean TouchStatus1=false; boolean TouchStatus2=false; boolean TouchStatus3=false; boolean TouchStatus4=false;</p><p>boolean RelayON=LOW; boolean RelayOFF=HIGH; byte Dot[8] = { 0b00000, 0b00100, 0b01110, 0b11111, 0b11111, 0b01110, 0b00100, 0b00000 }; byte Empty[8] = { 0b00000, 0b00100, 0b01010, 0b10001, 0b10001, 0b01010, 0b00100, 0b00000 };</p><p>void setup() { lcd.init(); // initialize the lcd // Print a message to the LCD. lcd.backlight(); lcd.print("Relay Control"); lcd.setCursor(3,1); lcd.print("M.Rawashdeh"); delay(4000); drawMainPanel(); lcd.createChar(0, Dot); lcd.createChar(1, Empty); pinMode(TouchPin1,INPUT); pinMode(TouchPin2,INPUT); pinMode(TouchPin3,INPUT); pinMode(TouchPin4,INPUT); pinMode(RelayPin1,OUTPUT); pinMode(RelayPin2,OUTPUT); digitalWrite(RelayPin1,RelayOFF); digitalWrite(RelayPin2,RelayOFF); }</p><p>void readTouch() { TouchStatus1=digitalRead(TouchPin1); TouchStatus2=digitalRead(TouchPin2); TouchStatus3=digitalRead(TouchPin3); TouchStatus4=digitalRead(TouchPin4); delay(10); } void printResult() { lcd.setCursor(3,1); lcd.print(TouchStatus1); lcd.setCursor(6,1); lcd.print(TouchStatus2); lcd.setCursor(9,1); lcd.print(TouchStatus3); lcd.setCursor(12,1); lcd.print(TouchStatus4); delay(50); } void drawMainPanel() { lcd.clear(); lcd.print("Relay1 Relay2"); // lcd.setCursor(0,1); }</p><p>void controlRelay() { if(TouchStatus1) { lcd.setCursor(0,1); lcd.print("OFF"); lcd.setCursor(3,1); lcd.write(1); digitalWrite(RelayPin1,RelayOFF); } if(TouchStatus2) { lcd.setCursor(0,1); lcd.print("ON "); lcd.setCursor(3,1); lcd.write(0); digitalWrite(RelayPin1,RelayON); } if(TouchStatus3) { lcd.setCursor(11,1); lcd.print("OFF"); lcd.setCursor(15,1); lcd.write(1); digitalWrite(RelayPin2,RelayOFF); } if(TouchStatus4) { lcd.setCursor(11,1); lcd.print("ON "); lcd.setCursor(15,1); lcd.write(0); digitalWrite(RelayPin2,RelayON); } delay(10); } void loop() { readTouch(); controlRelay(); delay(10); }</p>
Attachments
Step 5: Video
Here a video for the Servo and Relay code and simple test code.
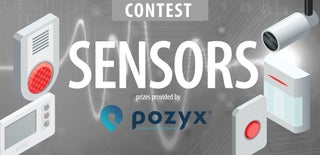
Participated in the
Sensors Contest 2017