Introduction: Telescope Motorization (focus + Orientation)
When you use your telescope to observe a planet, a galaxy, or a nebula, whether it be only for pleasure, or to take a picture, your telescope will shake every time you manipulate it (when you focus, when you track the object with the equatorial mount, etc).
A solution would be to tighten all the screws very hard, but it doesn't really work.
The real solution is motorization, or automation: just add motors so that you don't have to touch the telescope, just press buttons!
In this Instructable, I will teach you how to do it!
Let's learn!
Step 1: What You Need
For this project, you will need:
- an Arduino / Genuino board,
- a breadboard,
- 1 to 3 stepper motors(I use 28BYJ-48),
- a driver for each stepper (I use ULN2003),
- 2 to 4 push-buttons,
- 2 to 4 10 KΩ resistors,
- a bunch of jumper wires (male/male and male/female),
- 2 to 6 gears (Legos work well, but I use Buki),
- a soldering iron if you use a permanent breadboard,
- some hot glue.
The number of steppers, resistors, etc are variable because it depends if you want to fully automate the telescope or to automate only some parts...
Step 2: How It Works : Focus + Declination Axis
The circuit that controls the focus or the declination axis will feature a stepper motor and two push-buttons connected to the Arduino board.
For the focus, if you press a button, the stepper will rotate in a way and the lens will move in a direction. If you press the other button, the stepper will move the other way and the lens will move in the opposite direction.
For the declination axis, the mechanism will be the same but when the wheel will rotate, it will orientate the optical tube around the polar axis, instead of moving the lens.
This way you will be able to focus and orientate the optical tube around the declination axis without touching the wheel.
Step 3: Electronic Circuits : Focus / Declination Axis
Here is a Fritzing sketch of the system that controls the focus or the orientation around the declination axis.
The connections for the driver board are:
Connect the cables of the motors to the outputs of the board, and for the inputs, it's:
IN1 to Arduino pin 8,
IN2 to Arduino pin 10,
IN3 to Arduino pin 9,
IN4 to Arduino pin 11.
And don't forget to power the board (the pins next to the resistors must be connected to 5V and GND on the Arduino or on the breadboard).
Step 4: Code : Focus / Declination Axis
Here is the code for the focus or declination axis orientation that goes in the Arduino.
#include <Stepper.h> const int stepsPerRevolution = 200; const int button1 = 7; const int button2 = 4; Stepper myStepper(stepsPerRevolution, 8, 9, 10, 11); void setup() { pinMode(button1, INPUT); pinMode(button2, INPUT); myStepper.setSpeed(100); Serial.begin(9600); } void loop() { while(digitalRead(button2) == HIGH){ myStepper.step(-stepsPerRevolution); Serial.println("button2"); } while(digitalRead(button1) == HIGH){ myStepper.step(stepsPerRevolution); Serial.println("button1"); } while(digitalRead(button2) == LOW && digitalRead(button1) == LOW){ myStepper.step(0); Serial.println("none"); } }
Attachments
Step 5: How It Works: Polar Axis
The equatorial mount is a very cool system that enables you to track an object in the sky all along the night, following the rotation of the Earth, using only one wheel.
While automated, you won't have to touch this wheel.
The speed of the motor depends on the rotation speed of the Earth, so it is static.
It has to be calculated using the number of steps per rotation, the gears ratio, and the type of stepper and driver used.
Step 6: Electronic Circuit: Polar Axis
Here is a Fritzing sketch of the system that controls the orientation around the polar axis.
The connections for the driver board are:
Connect the cables of the motors to the outputs of the board, and for the inputs, it's:
IN1 to Arduino pin 8,
IN2 to Arduino pin 10,
IN3 to Arduino pin 9,
IN4 to Arduino pin 11.
And don't forget to power the board (the pins next to the resistors must be connected to 5V and GND on the Arduino or on the breadboard).
Step 7: Code : Polar Axis
Here is the code that goes in the Arduino to control the polar axis orientation:
#include <Stepper.h> const int stepsPerRevolution = 200; Stepper myStepper(stepsPerRevolution, 8, 9, 10, 11); void setup() {} void loop() { int motorSpeed = 10; //to be calculated, depending on your stepper and gear ratio if (motorSpeed > 0) { myStepper.setSpeed(motorSpeed); myStepper.step(stepsPerRevolution / 100); } }
Step 8: Build It, Use It !
Declination axis + polar axis (pictures on Step 5):
Now you will have to fix the gears on the steppers and on the axis wheels using hot glue.
Then you will have to solidly fix the stepper to the structure, using hot glue too.
(Note that on the pictures in Step 5, the blue gear replaces the control wheel. It is fixed on the wheel's shaft.)
Focus (pictures on Step 2):
You will have to fix the gears on the stepper and on the focus wheel using hot glue.
Then solidly hot-glue the stepper to the optical tube of your telescope.
And you are done!
If you want, you can combine all the different parts of this project, and even build a remote control !
Now you have a fully motorized telescope.
You can track objects automatically, focus and orientate the tube without touching the telescope!
Thanks for reading, you can check my other Instructables.
Feel free to post comments, I will enjoy answering them!
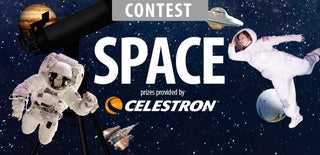
Participated in the
Space Contest 2016