Introduction: The Reusable and Wireless Igniter
This project uses a simple RF link and two Arduinos to ignite something from up to 90 feet away! I always found it annoying to mess with a bunch of wire, so I felt like creating something to fix that problem. A wireless connection is not only safer, but also A LOT cooler. This wireless ignitor can be used to ignite fireworks, model rockets, and more!
This is PART ONE of a three part project! See the other parts by visiting my page!
Disclaimer: This is not intended to be used as anything but a safe way to ignite fireworks, a model rocket, etc. Be cautious whenever you are building this or using this device. I take no responsibility for any harm done due to any carless action or a failure to follow the proper safety measures.
Step 1: Materials
Sparkfun wish list with all of the parts here
Component | Supplier | Price |
---|---|---|
Arduino x2 | Sparkfun buying guide, Radioshack | $50-60 |
RF link modules | Sparkfun/Sparkfun | $9 |
Enclosure x2 | Sparkfun, Radioshack | $7-12 |
Toggle switch | Sparkfun, Radioshack | $3-5.50 |
On/off switch x2 | Sparkfun, Radioshack | $.75-4 |
Speaker(optional) | Sparkfun, Radioshack | $2-4 |
Glow plug | Amazon | $6 |
LEDs | Sparkfun, Radioshack | $1-5.50 |
5V Relay | Sparkfun, Radioshack | $2-5 |
Assorted resistors | Sparkfun, Radioshack | $8-$15 |
8-Pin Sockets | Sparkfun, Radioshack | $1-2 |
Total | ~$85-130 |
Tools:
--Wire (for antennae)
--Perfboard
--A few male headers
--Something to use as a cushion for the arduino, or some standoffs
--Drill
--File and/or sandpaper
--Soldering iron/solder
--Wire cutter/stripper
--Miscellaneous screws, nuts, etc.
--Big alligator clip
--Battery holder for 2 AA batteries (will be modified)
--9V Battery with connectors
--1.5V AA Batteries x2
Step 2: Getting VirtualWire
All of the code involved in this project is centered around an Arduino library called VirtualWire, by Mike McCauley. This library pushes the cheap RF link modules to their limits.
1.) This library can be downloaded directly from here, or on the website here.
2.) Find the location of your Arduino sketchbook by checking your Arduino preferences.
3.) Make a folder called "libraries" if it's not already there and place the VirtualWire folder in it.
4.) Open arduino and you're done!
Step 3: Creating the Virtual Wire
Step 4: Schematics
Follow the schematics shown in the pictures and build your circuit. I realize I forgot to draw the arrows on the LEDs, that's why they're marked.
This circuit is designed so when the switch is flicked on the transmitter, the signal is received and triggers a 5-second countdown before it ignites the fuse.
Note: I found that the wire size required for the glow plug to work properly is thicker than a normal jumper wire, or even the breadboard tracks. Therefore, it might be necessary to connect the glow plug leads directly with a few alligator clips.
Step 5: Setting Up the Igniter
The glow plug I'm using is designed for use in a RC car to ignite an air-fuel mixture. It runs perfectly off of 1.5V and burns red-hot on its inner coil. It might be necessary to provide a step-up material (something that ignites at a low temperature, but burns at a higher one), such as flash cotton, or stick a small piece of your fuse inside the plug's coil.
One AA battery proved not to supply enough current for the glow plug to run properly, so I decided to create a battery holder to make two AA batteries run in parallel. I then soldered the glow plug to an alligator clip:
Step 6: Putting It in the Enclosure
First, drill a few holes and file out the edges to fit the parts you are using. Make sure to drill an array of holes for the speaker if you decide to use one.
To neaten things up, I made a small shield to fit over the arduino. I also wanted to reuse the receiver and transmitter, so I put them on a split IC socket, as shown in the pictures.
I used some foam I cut to hold the arduino in place, but standoffs and screws would work too.
Step 7: Conclusion
I hope you enjoyed learning how to make a reusable wireless igniter!!
Here's a little clip showing it in action:
Be sure to leave feedback!
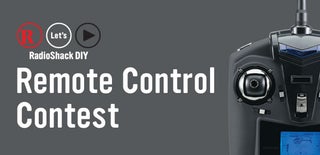
Second Prize in the
Remote Control Contest