Introduction: UStepper Robot Arm 4
This is the 4th iteration of my Robotic arm, which I have developed as an application for our uStepper stepper control board. Since the robot has 3 stepper motors and a servo for actuation (in it's basic configuration) it is not limited to uStepper, but can be used with any stepper driver board.
The design is based on an industrial palletizer robot - and is relatively simple. With that said, I have spent countless hours coming up with the design and optimizing it both for ease of assembly, but also ease of printing the parts.
I did the design with ease of printing and simplicity of assembly in mind. Not that there isn't any way to improve on those two parameters, but I think I have come a long way. Furthermore, I would like to pull industrial robotics down to a level where hobbyist can follow it by showing that it can be made relatively simple - also the math to control it !
Feel free to leave a comment with constructive feedback on both the design but most of all on how I do on making it accessible to all (especially the math).
Step 1: Parts Required, 3D Printing and Assembly
Basically everything you need to know is in the assembly manual. There is a detailed BOM with both purchased and printed parts and a detailed assembly instruction.
3D printing is done on a reasonable quality 3D printer (FDM) with layer height of 0.2 mm and 30 % infill. You can find the latest iteration of parts and instructions here: https://github.com/uStepper/Robot-Arm-Rev-4
Attachments
Step 2: Kinematics
To make the arm move around in a foreseeable manner you need to do math :O I have looked a lot of places for a relatively simple description of the kinematics related to this type of robot, but I haven't found one that I believe was on a level were most people could understand it. I have done my own version of the kinematics based solely on trigonometry and not the matrix transforms that can seem quite scary if you have never worked on that stuff before - however, they are quite simple for this particular robot since it is only 3 DOF.
Never the less I think my approach in the attached document is written in a relatively easy to understand manner. But take a look and see if it makes sense to you !
Attachments
Step 3: Coding the Kinematics !
The kinematics can be hard to grasp even with the calculations I provided in the former. So here is first of all an Octave implementation - Octave is a free tool with many of the same features found in Matlab.
L1o = 40;
Zo = -70; L_2 = 73.0; Au = 188.0; Al = 182.0; Lo = 47.0; UPPERARMLEN = Au; LOWERARMLEN = Al; XOFFSET = Lo; ZOFFSET = L_2; AZOFFSET = Zo; AXOFFSET = L1o; disp('Code implementation') disp('Input angles:') rot = deg2rad(30); right = deg2rad(142.5); left = deg2rad(50); rad2deg(rot) rad2deg(right) rad2deg(left) T1 = rot;#base T2 = right;#shoulder T3 = left;#elbow #FW kinematics to get XYZ from angles: disp('Calculated X, Y, Z:') z = ZOFFSET + sin(right)*LOWERARMLEN - cos(left - (pi/2 - right))*UPPERARMLEN + AZOFFSET k1 = sin(left - (pi/2 - right ))*UPPERARMLEN + cos(right)*LOWERARMLEN + XOFFSET + AXOFFSET ; x = cos(rot)*k1 y = sin(rot)*k1 ##inverse kinematics to get angles from XYZ: rot = atan2(y,x); x = x - cos(rot)*AXOFFSET; y = y - sin(rot)*AXOFFSET; z = z - AZOFFSET-ZOFFSET; L1 = sqrt( x*x + y*y ) - XOFFSET; L2 = sqrt( (L1)*(L1) + (z)*(z) ); a = (z)/L2; b = (L2*L2 + LOWERARMLEN*LOWERARMLEN - UPPERARMLEN*UPPERARMLEN)/(2*L2*LOWERARMLEN); c = (LOWERARMLEN*LOWERARMLEN + UPPERARMLEN*UPPERARMLEN - L2*L2)/(2*LOWERARMLEN*UPPERARMLEN); right = ( atan2(a, sqrt(1-a*a) ) + atan2(sqrt(1-b*b), b) ); left = atan2(sqrt(1-c*c), c); ##output calculated angles disp('Output angles:') rot = rad2deg(rot) right = rad2deg(right) left = rad2deg(left)
With the above script you basically have the implementation ready code for forward and backward kinematics.
Forward Kinematics you use for calculating where you will end up with a given set of motor angles. Inverse kinematics will then (do the inverse) calculate what motor angles you need to end up at a desired x,y,z position. Constraints on motor movement then has to be inserted, like e.g. rotational base can only go from 0 to 359 degree. This way you ensure that you wont go to positions that are not feasible.
Step 4: Running the Thing !
We are not quite there with the kinematics library implementation, so that I can't provide yet. But I can show you a video of how it's running. It's quite stable and smooth because of the use of bearings and belt drive, besides reasonable quality of drives which is here the uStepper S boards.
Step 5: Additional End Effectors
I have designed 3 additional end effectors. One is quite simply a horizontal gripper, another one fits a regular European beer or soda can and lastly there is a vacuum gripper system that enables you to fit on a vacuum cup, pump and valve.
All will be or are available here (3D STL files and instructions): https://github.com/uStepper/Robot-Arm-Rev-4
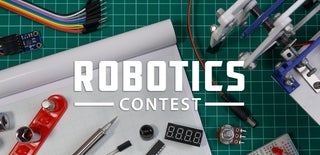
Participated in the
Robotics Contest