Introduction: WS2812 RGB LEDs With Web Control and Arduino Yun
This instructable aims to help you get started with an Arduino YUN which is controlling a string of WS2812 RGB leds. The WS2812 is an individually addressable flexible LED strand. The strand can be cut, or put back together, and has a fairly high bending tolerance. The WS2812 is also completely digital, being driven by a single Data In pin rather than individual channels for red, green and blue.
In our demo we have an Arudino YUN running PHP and the PHP Bridge library which will allow us to send our color string to the Arduino and change the color of our LED strand. We also have a button to turn the strand on and off.
Step 1: Circuit Diagram
The basic diagram for our circuit looks like the above.
We have an Arduino YUN connected to our network via Ethernet. There is a common ground that ties the button and WS2812 strand together.
The button:
We are supplying 5v to one leg of the button and a 10KΩ resistor connects that 5v leg to ground. Our opposite leg is connected to pin 3 on the Arduino.
The WS2812 Strand:
The WS2812 data is transmitted via Pin 6 on our Arduino, and the line is protected by a 220Ω resistor. We also tie the grounds together from our strand and power supply. Because the WS2812 can have a rather large power budget you must drive it off an independent 5 volt power supply. You can use your 5 volt supply to the Vin pin on the Arduino if you want less power cables to manage, for this example I am using USB power from the computer to drive the Arduino.
Step 2: Parts
You will need:
- Arduino Yun
- 10K Ω resistor
- 220 Ω resistor
- 5 volt, 10 amp power supply
- Momentary button
- Some wires
- WS2812 LED Strip - I used sparkfun's 1m sealed strip for this demo as eventually this strip will go next to my sink.
- Breadboard
Some optional, but useful components - JST-SM male connector to attach to Sparkfun's pre-attached leads
- Barrel to terminal adapter to connect the Leds to your power supply.
- MicroSD Card for the Yun to store data
Step 3: Arduino Code
The arduino code is built to run as little as possible, continuously, inside the main loop. The main loop does two things in its 'rest' state. The first piece is to check and see if our button has been pressed, and what state we are moving through (on to off or off to on) and the loop also iterates through some debounce code for the button.
If the power state has changed to on the loop then works through the bridge parameters to get the colors, mainly to see if the colors have changed. This is triggered via a command variable, cmd, that is appended to the bridge data. If the colors have not changed then it will continue to work through the loop endless waiting for permission to continue.
If the cmd variable is set to go then the loop iterates through the process of changing the color. Once it is done setting the color of the leds it resets itself back into the stop mode, to avoid unnecessary iterations. Likewise if the power state changes to the off state the loop closes back down to simply listening for button presses.
Attachments
Step 4: PHP Code
The PHP file is split into two sections. The top half of the file does the heavy lifting. This file does require the PHP Bridge class which can be found here: GitHub - Bridge The php code does a handful of things. Firstly it instantiates the bridge client, it contains two functions for converting the decimal rgb colors into hex, and from converting hex html colors into RGB for the FastLED library. The last piece of the php code waits for a submission of the form and then builds and transmits the bridge string. I am using a single string of data to send all my commands to the Arduino, which is then disassembled on the other side. It helps reduce the number of calls that I needed to make.
The second half of the code contains a simple HTML form and an HTML5 color picker. The form action is self referential, once submit is pressed the data is passed into POST and then the PHP code trips. There is also a petty vanity here where in the PHP code gets from the Arduino the last set of colors and converts them back to HTML hex and then sets the color picker to the color stored on the Arduino. If no value is found it will default to black.
This code is small enough to fit on the Linio side of the Yun, but if you want to expand this any further it would be a good idea to get a microsd card to store your PHP files on.
Step 5: Configuring the Linino Linux Device
To configure the Linino Linux distribution to work with these sketches and PHP code there are a couple of small changes that need to happen. First you need to install php5, php5-cgi, php5-mod-json and php5-mod-sockets:
opkg install php5 php5-cgi php5-mod-json php5-mod-sockets
For my linino install I did not want to do away with the Luci install, but I did want my Lights code to be the default index for my Yun. So I created two instances of uhttpd. The first install, the Luci instance points to ports 8080, instead of port 80.
/etc/config/uhttpd
config uhttpd main<br> list listen_http 0.0.0.0:8080 option home /www option cgi_prefix /cgi-bin option rfc1918_filter 0 option max_requests 2 option cert /etc/uhttpd.crt option key /etc/uhttpd.key # Lua url prefix and handler script. # Lua support is disabled if no prefix given. # option lua_prefix /luci # option lua_handler /usr/lib/lua/luci/sgi/uhttpd.lua # list interpreter ".php=/usr/bin/php-cgi" # list interpreter ".cgi=/usr/bin/perl" option script_timeout 60 option network_timeout 30 option tcp_keepalive 1 option realm OpenWrt # option config /etc/httpd.conf
The second instance is 'my' instance, listening on port 80. I've also changed its root directory to my SD card and enabled PHP.
config uhttpd secondary<br> list listen_http 0.0.0.0:80 option home /mnt/sda1/lights option cgi_prefix /mnt/sda1/lights/cgi-bin option rfc1918_filter 0 option max_requests 5 option cert /etc/uhttpd.crt option key /etc/uhttpd.key list interpreter ".php=/usr/bin/php-cgi" list interpreter ".cgi=/usr/bin/perl" list interpreter ".py=/usr/bin/python" list interpreter ".php=/usr/bin/php-cgi" option script_timeout 60 option_network_timeout 30 option tcp_keepalive 1 option_realm Lights
Lastly I needed to edit PHP.ini and make sure that my PHP Bridge class was enabled. I also uncommented the mod_json and mod_sockets extensions.
/etc/php.ini
; Enable json extension module <br>extension=json.so ; Enable sockets extention module extension=sockets.so include_path /usr/lib/php/bridge
With those changes made I was ready to put it all together.
Attachments
Step 6: Putting It All Together.
Finally! We can wire everything up.
The steps that I did this in are as follows.
- Make sure the Yun is off.
- Connect the ground wires to the breadboard and to the ground on the WS2182 to a ground pin on the Yun.
- Connect Pin 6 of the Arduino to the DIN connector on the LED strand.
- I connected Pin 6 to one half of my breadboard
- I bridged the middle with my 220 Ω resistor.
- I connected the DIN pin to the other side of the board.
Make sure that you are connecting to the DIN and not the DOUT side of the strand, as they are directional.
- Connect your button
- I bridged my breadboad with my button
- I connected Pin 3 to one side of the button
- Opposite Pin 3 I bridged to ground with my 10KΩ pull down resistor.
- I connected the Arduino 5 volt leg to the same side of the button as our 10KΩ pull down.
- Connect the 5v, 50w power supply to the ground and power leads of your LED Strand
- Power back on the Yun
- Test it out
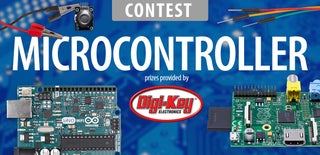
Participated in the
Microcontroller Contest 2017