Introduction: ATX Home Security Robot Rover
Let's build an internet controlled security robot?
Everything that you need is:
- Arduino Duemilanove (heart of robot);
- Bluetooth HC-05 module (communicator);
- Serial LCD Module 20x4 Blue with White Backlight (display);
- Ultrasonic sensor HC-SR04 (distance sensor for blind driving);
- Bridge H L298N (to control de dc motors);
- Caster (every direction enabled);
- 2 x DC motors (to go everywhere with the robot);
- An old ATX power supply (will be the case of the robot);
- 12V Lipo Battery;
- And finally an old android cellphone (the brain of the robot).
What functions does it have?
- Rover Mode - will respect all commands sent by internet website.
- Fear Mode - will alert any kind of proximity and send pictures to an e-mail.
- Measuring Tape - will display the distance of an obstacle.
- Light Mode - will turn on the light to see in the dark.
Step 1: Prototype
Be free to prototype every step outside of the power supply.
It will help you to have more space to work till you don't know where to put the components.
Step 2: Cleaning the Power Supply and Installing the DC Motors
Cleaning
First thing that we must do is to take out all electrical components of the power supply.
If your power supply has an power button, keep that, it will be useful to power your Arduino board.
Be careful to remove the components and be sure that they are all uncharged.
Installing DC motors
Measure the distance of the holes that you will open and then use the drill to do that (I've used the reduction box because it comes with the holes to fasten it), then make two more holes to put the motor wires (I've used two holes each motor, but you can put both in only one hole).
Step 3: Putting the Caster and the Robot Floor
Caster
This caster in the example is a 4 holes based, then I've measured the distance of them and made all the holes in the center of the case.
Take sure if the caster is able to spin for all sides, if this cause a minimal problem you will have problem with the robot direction.
Floor
To make the floor I've used an old router case and then made 4 holes in the power supply case and fasten that with the box.
Step 4: Putting the Bridge and the Arduino Board
To put the Arduino board and the Bridge I've made the holes using the screw.
Check for more on this instructables.
Step 5: Phone Holder
To put the phone holder I just bended an small aluminium plate and fastened that with the power supply case.
Step 6: Android Programming
The Android application is responsible to get internet data and send it to ATX2 arduino board.
Bluetooth manager and sender:
package com.example.alisson.atx2;
import android.app.Activity; import android.bluetooth.BluetoothDevice; import android.bluetooth.BluetoothSocket; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.content.IntentFilter; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.bluetooth.BluetoothAdapter; import android.view.View; import android.widget.ArrayAdapter; import android.widget.EditText; import android.widget.ListAdapter; import android.widget.ListView; import android.widget.SimpleCursorAdapter; import android.widget.Toast;
import com.dropbox.sync.android.DbxAccountManager; import com.dropbox.sync.android.DbxFile; import com.dropbox.sync.android.DbxFileSystem; import com.dropbox.sync.android.DbxPath;
import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.util.ArrayList; import java.util.Set; import java.util.UUID;
public class MainActivity extends Activity { private BluetoothSocket mmSocket; private BluetoothDevice mmDevice; private InputStream mmInStream; private OutputStream mmOutStream;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); }
@Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; }
@Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId();
//noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; }
return super.onOptionsItemSelected(item); }
public void checkDevices(View view) {
final int REQUEST_ENABLE_BT = 0; BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); final ArrayList mArrayAdapter = new ArrayList();
if (mBluetoothAdapter == null) { // Device does not support Bluetooth } if (!mBluetoothAdapter.isEnabled()) { Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT); //Ativa Bluetooth }
Set pairedDevices = mBluetoothAdapter.getBondedDevices(); // If there are paired devices if (pairedDevices.size() > 0) { // Loop through paired devices for (BluetoothDevice device : pairedDevices) { // Add the name and address to an array adapter to show in a ListView mArrayAdapter.add(device.getName() + "\n" + device.getAddress());
Context context = getApplicationContext(); int duration = Toast.LENGTH_SHORT; //Toast toast = Toast.makeText(context, (device.getName() + "\n" + device.getAddress()), duration); if(device.getName().startsWith("HC")){ ConnectThread ct = new ConnectThread(device); ct.run(); } //toast.show(); } } }
private class ConnectThread extends Thread {
public ConnectThread(BluetoothDevice device) { // Use a temporary object that is later assigned to mmSocket, // because mmSocket is final BluetoothSocket tmp = null; mmDevice = device; UUID uuid = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"); // Get a BluetoothSocket to connect with the given BluetoothDevice try { // MY_UUID is the app's UUID string, also used by the server code tmp = device.createRfcommSocketToServiceRecord(uuid); } catch (IOException e) { } mmSocket = tmp; }
public void run() { try { // Connect the device through the socket. This will block // until it succeeds or throws an exception mmSocket.connect(); Context context = getApplicationContext(); int duration = Toast.LENGTH_SHORT; Toast toast = Toast.makeText(context, ("SUCESSO"), duration); toast.show(); ConnectedThread ct = new ConnectedThread(mmSocket); ct.write("5"); } catch (IOException connectException) { Context context = getApplicationContext(); int duration = Toast.LENGTH_SHORT; Toast toast = Toast.makeText(context, (connectException.toString()), duration); toast.show(); // Unable to connect; close the socket and get out try { mmSocket.close(); } catch (IOException closeException) { } return; }
}
/** Will cancel an in-progress connection, and close the socket */ public void cancel() { try { mmSocket.close(); } catch (IOException e) { } } }
private class ConnectedThread extends Thread {
public ConnectedThread(BluetoothSocket socket) { //mmSocket = socket; InputStream tmpIn = null; OutputStream tmpOut = null; try { tmpIn = socket.getInputStream(); tmpOut = socket.getOutputStream(); } catch (IOException e) { }
mmInStream = tmpIn; mmOutStream = tmpOut; }
public void run() { byte[] buffer = new byte[1024]; // buffer store for the stream int bytes; // bytes returned from read()
// Keep listening to the InputStream until an exception occurs while (true) { try { // Read from the InputStream bytes = mmInStream.read(buffer);
//mHandler.obtainMessage(MESSAGE_READ, bytes, -1, buffer) //.sendToTarget(); } catch (IOException e) { break; } } }
/* Call this from the main activity to send data to the remote device */ public void write(String message) {
byte[] msgBuffer = message.getBytes();
try { mmOutStream.write(msgBuffer); } catch (IOException e) { String msg = "In onResume() and an exception occurred during write: " + e.getMessage(); msg = msg + ".\n\nCheck that the SPP UUID: exists on server.\n\n"; } }
/* Call this from the main activity to shutdown the connection */ public void cancel() { try { mmSocket.close(); } catch (IOException e) { Context context = getApplicationContext(); int duration = Toast.LENGTH_SHORT; Toast toast = Toast.makeText(context, (e.toString()), duration); toast.show();} } }
}
Step 7: Html Page Programming
The easiest way that I've found to send those datas was an webpage, so I just created it http://www.atx2.esy.es/.
This page is responsible to record the commands on database.
This other page is the page that android application opens and read to check new commands http://www.atx2.esy.es/message.php
Step 8: Arduino Programming
This is the arduino code to read and execute the commands:
#define echoPin 10 // Echo Pin
#define trigPin 11 // Trigger Pin #include #include // Inicializa o display no endereco 0x27 LiquidCrystal_I2C lcd(0x27, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE);
long duration, distance; long randomPisca; int type; int IN1 = 4; int IN2 = 5; int IN3 = 6; int IN4 = 7; String mode = "rover";
void setup() { delay(650); Serial.begin(9600); lcd.begin (20, 4); pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); pinMode(IN1, OUTPUT); pinMode(IN2, OUTPUT); pinMode(IN3, OUTPUT); pinMode(IN4, OUTPUT); randomSeed(analogRead(A0)); engage(); }
void loop() { if (mode == "rover") { randomPisca = random(1000); if (randomPisca <= 12.0) { pisca(); } else if (randomPisca >= 13.0 && randomPisca <= 20.0) { olhaDireita(); delay(500); olhaEsquerda(); delay(500); } else olhaFrente(); } if (mode == "trena") { cls(); digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = duration / 58.2; Serial.print(distance); lcd.setCursor(0, 0); lcd.print(" ATX 2.0 "); lcd.setCursor(1, 1); lcd.print(distance); lcd.setCursor(1, 2); lcd.print("cm"); lcd.setCursor(0, 3); lcd.print(" Modo trena "); delay(400); } if (mode == "medo") { digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = duration / 58.2; Serial.print(distance); Serial.print("cm "); Serial.println(); if (distance <= 20) { olhaCima(); tras(); } else olhaFrente(); } if (Serial.available() > 0) { type = Serial.read(); if (mode == "rover") { if (type == '1') { digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = duration / 58.2; Serial.print(distance); } if (type == '2') { olhaBugado(); tras(); } if (type == '5') { olhaFrente(); frente(); } if (type == '6') { olhaEsquerda(); esquerda(); } if (type == '4') { olhaDireita(); direita(); } else { } } if (type == 'd') { lcd.setBacklight(LOW); } if (type == 'l') { lcd.setBacklight(HIGH); } if (type == 't') { mode = "trena"; } if (type == 'r') { mode = "rover"; } if (type == 'm') { mode = "medo"; } } } void tras() { //Tras digitalWrite(IN1, LOW); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, LOW); delay(200); digitalWrite(IN1, HIGH); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, HIGH); delay(10); } void frente() { //Frente digitalWrite(IN1, HIGH); digitalWrite(IN2, LOW); digitalWrite(IN3, LOW); digitalWrite(IN4, HIGH); delay(200); digitalWrite(IN1, HIGH); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, HIGH); delay(10); } void direita() { //Direita digitalWrite(IN1, HIGH); digitalWrite(IN2, LOW); digitalWrite(IN3, HIGH); digitalWrite(IN4, LOW); delay(200); digitalWrite(IN1, HIGH); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, HIGH); delay(10); } void esquerda() { //Esquerda digitalWrite(IN1, LOW); digitalWrite(IN2, HIGH); digitalWrite(IN3, LOW); digitalWrite(IN4, HIGH); delay(200); digitalWrite(IN1, HIGH); digitalWrite(IN2, HIGH); digitalWrite(IN3, HIGH); digitalWrite(IN4, HIGH); delay(10); } void engage() { lcd.setBacklight(HIGH); lcd.setCursor(0, 0); lcd.print("*------------------*"); lcd.setCursor(0, 1); lcd.print("| ATX 2.0 |"); lcd.setCursor(0, 2); lcd.print("| (ALS Robots) |"); lcd.setCursor(0, 3); lcd.print("*------------------*"); delay(3000); lcd.setCursor(0, 0); lcd.print(" ------------------ "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" (ALS Robots) "); lcd.setCursor(0, 3); lcd.print(" ------------------ "); delay(100); lcd.setCursor(0, 0); lcd.print(" ---------------- "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" (ALS Robots) "); lcd.setCursor(0, 3); lcd.print(" ---------------- "); delay(100); lcd.setCursor(0, 0); lcd.print(" -------------- "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" (ALS Robots) "); lcd.setCursor(0, 3); lcd.print(" -------------- "); delay(100); lcd.setCursor(0, 0); lcd.print(" ------------ "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" (ALS Robots) "); lcd.setCursor(0, 3); lcd.print(" ------------ "); delay(100); lcd.setCursor(0, 0); lcd.print(" ---------- "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" ALS Robots "); lcd.setCursor(0, 3); lcd.print(" ---------- "); delay(100); lcd.setCursor(0, 0); lcd.print(" -------- "); lcd.setCursor(0, 1); lcd.print(" ATX 2.0 "); lcd.setCursor(0, 2); lcd.print(" LS Robot "); lcd.setCursor(0, 3); lcd.print(" -------- "); delay(100); lcd.setCursor(0, 0); lcd.print(" ------ "); lcd.setCursor(0, 1); lcd.print(" TX 2.0 "); lcd.setCursor(0, 2); lcd.print(" S Robo "); lcd.setCursor(0, 3); lcd.print(" ------ "); delay(100); lcd.setCursor(0, 0); lcd.print(" ---- "); lcd.setCursor(0, 1); lcd.print(" X 2. "); lcd.setCursor(0, 2); lcd.print(" Rob "); lcd.setCursor(0, 3); lcd.print(" ---- "); delay(100); lcd.setCursor(0, 0); lcd.print(" -- "); lcd.setCursor(0, 1); lcd.print(" 2 "); lcd.setCursor(0, 2); lcd.print(" Ro "); lcd.setCursor(0, 3); lcd.print(" -- "); delay(100); lcd.setCursor(0, 0); lcd.print(" "); lcd.setCursor(0, 1); lcd.print(" "); lcd.setCursor(0, 2); lcd.print(" "); lcd.setCursor(0, 3); lcd.print(" "); delay(200); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O| | O| "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(700); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O | | O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(700); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" |O | |O | "); lcd.setCursor(0, 2); lcd.print(" | | | | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(700); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | O| | O| "); lcd.setCursor(0, 2); lcd.print(" | | | | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(700); pisca(); } void pisca() { delay(100); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" |___| |___| "); lcd.setCursor(0, 2); lcd.print(" | O | | O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(100); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" |___| |___| "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(100); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" |___| |___| "); lcd.setCursor(0, 2); lcd.print(" | O | | O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(100); lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O | | O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); delay(1000); } void olhaDireita() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O| | O| "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void olhaEsquerda() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" |O | |O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void olhaFrente() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O | | O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void olhaBugado() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | O| "); lcd.setCursor(0, 2); lcd.print(" |O | | | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void olhaVesgo() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | | | | "); lcd.setCursor(0, 2); lcd.print(" | O| |O | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void olhaCima() { lcd.setCursor(0, 0); lcd.print(" ___ ___ "); lcd.setCursor(0, 1); lcd.print(" | O | | O | "); lcd.setCursor(0, 2); lcd.print(" | | | | "); lcd.setCursor(0, 3); lcd.print(" --- --- "); } void cls() { lcd.setCursor(0, 0); lcd.print(" "); lcd.setCursor(0, 1); lcd.print(" "); lcd.setCursor(0, 2); lcd.print(" "); lcd.setCursor(0, 3); lcd.print(" "); }
Step 9:
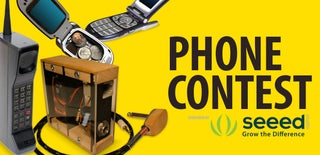
Participated in the
Phone Contest
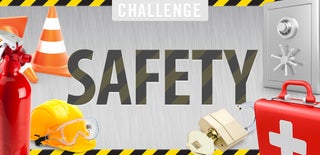
Participated in the
Safety Challenge